How to Read .env Files in Node.js (Updated for 2025)
Grace Collins
Solutions Engineer · Leapcell
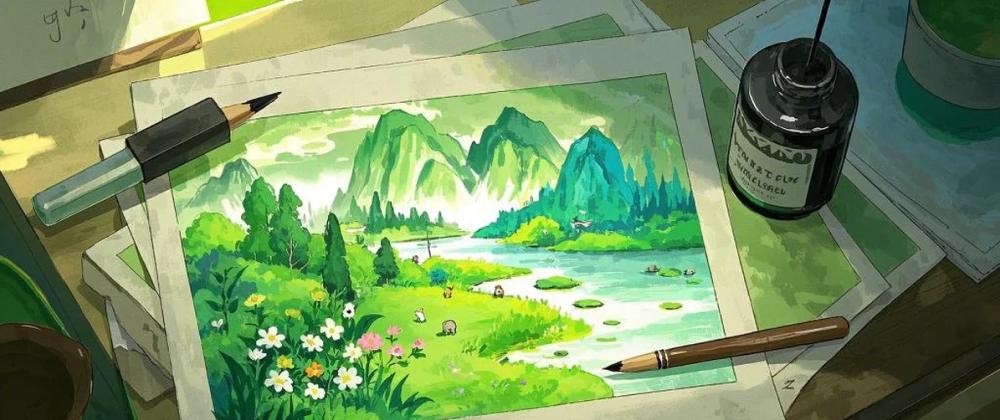
Environment variables play a crucial role in application development, enabling flexible configuration without hardcoding sensitive or environment-specific details. Node.js provides multiple ways to load and manage .env
files, depending on the version you are using. This article will cover methods for Node.js versions both above and below 20, as well as how to implement a custom solution if needed.
Key Takeaways
- Node.js > 20 supports native
.env
file handling via CLI orprocess.loadEnvFile()
. - Node.js < 20 can use
dotenv
for.env
management. - Custom
.env
file parsers offer flexibility without dependencies.
If Your Node.js Version > 20
For Node.js versions greater than 20, you can leverage built-in support to load .env
files, eliminating the need for external dependencies. Here are the options:
1. Use the --env-file
CLI Parameter
From Node.js v20.6.0, you can load a .env
file directly using the --env-file
command-line argument. This allows you to inject variables into the process.env
object without modifying your code.
-
Create a
.env
file:PORT=3000 DB_HOST=localhost DB_USER=admin DB_PASSWORD=secret
-
Run your application:
node --env-file=.env app.js
The variables defined in
.env
will now be available inprocess.env
.
2. Use the process.loadEnvFile()
API
From Node.js v20.12.0, the experimental process.loadEnvFile()
API allows you to dynamically load a .env
file within your application code.
-
Example Usage:
(async () => { await process.loadEnvFile('.env'); console.log(`Server is running on port ${process.env.PORT}`); })();
This method is particularly useful if you need programmatic control over when the
.env
file is loaded. However, since it is an experimental feature, its behavior may change in future Node.js releases.
If Your Node.js Version < 20
For earlier versions of Node.js, you will need to use external libraries or implement your own logic to handle .env
files.
1. Use the dotenv
Library
The dotenv
library is a widely used solution for managing .env
files. It works seamlessly across all Node.js versions and is simple to set up.
-
Install the library:
npm install dotenv
-
Use it in your application:
require('dotenv').config(); console.log(`Server is running on port ${process.env.PORT}`);
The
.env
file is automatically loaded, and its variables are added toprocess.env
.
2. Implement Your Own Logic
If you prefer not to use external libraries, you can write a simple function to parse .env
files and load them into process.env
.
-
Example Implementation:
const fs = require('fs'); const path = require('path'); function loadEnvFile(filePath) { try { const resolvedPath = path.resolve(filePath); const data = fs.readFileSync(resolvedPath, 'utf-8'); data.split(/\r?\n/).forEach((line) => { const trimmedLine = line.trim(); if (!trimmedLine || trimmedLine.startsWith('#')) return; const [key, value] = trimmedLine.split('='); if (key && value !== undefined) { process.env[key.trim()] = value.trim(); } }); console.log('Environment variables loaded successfully.'); } catch (error) { console.error(`Error loading .env file: ${error.message}`); } } // Usage loadEnvFile('.env'); console.log(`Server is running on port ${process.env.PORT}`);
This approach provides full control over how
.env
files are parsed and processed, which can be useful for advanced use cases or custom formats.
FAQs
It eliminates the need for third-party libraries.
No, it’s an experimental feature in Node.js v20.12.0+.
Only a custom parser allows full control over formats.
Conclusion
Depending on your Node.js version, you can choose the most suitable approach to manage .env
files:
- Node.js > 20:
- Use the
--env-file
CLI parameter for quick loading. - Use the
process.loadEnvFile()
API for dynamic programmatic control.
- Use the
- Node.js < 20:
- Use the popular
dotenv
library for reliable and easy-to-use support. - Implement your own
.env
loader for custom requirements.
- Use the popular
Each method has its benefits, and choosing the right one depends on your project needs and Node.js version.
We are Leapcell, your top choice for deploying Node.js projects to the cloud.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ