A Beginner’s Guide to Using Framer Motion
James Reed
Infrastructure Engineer · Leapcell
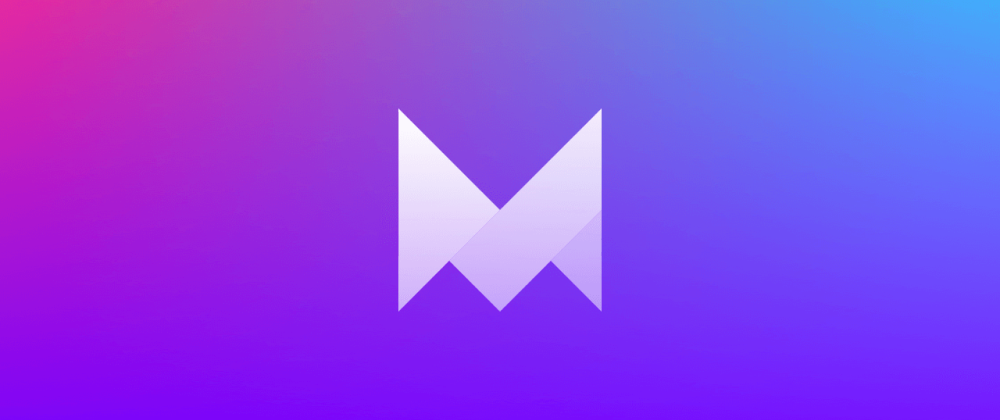
Framer Motion is a popular animation library for React, designed to help developers create fluid, visually appealing animations with minimal effort. Whether you're building a sleek user interface or a dynamic web experience, Framer Motion provides powerful tools to make animations effortless and elegant. This guide will walk you through the basics of using Framer Motion, with code examples and best practices.
Key Takeaways
- Framer Motion simplifies React animations with an intuitive API and powerful features.
- Key tools include
motion
components,AnimatePresence
, andvariants
for managing animation states. - Best practices involve starting simple, reusing variants, and optimizing performance.
What Is Framer Motion?
Framer Motion is a declarative library that simplifies animations in React applications. It offers:
- Ease of Use: Intuitive syntax and APIs.
- Flexibility: Supports both simple and complex animations.
- Performance: Optimized for smooth transitions.
- Accessibility: Handles reduced-motion preferences for users.
Installation
To get started, install Framer Motion via npm or yarn:
npm install framer-motion # or yarn add framer-motion
Core Concepts
Framer Motion is built around a few core components and hooks. Here are the essentials:
- motion Component: Replace standard HTML elements with
motion
components to animate them. - AnimatePresence: Handles mounting and unmounting animations.
- useAnimation: Provides programmatic control over animations.
- Variants: Define reusable animation states.
Basic Animation
Here’s a simple example of animating a box:
import React from 'react'; import { motion } from 'framer-motion'; const App = () => { return ( <motion.div style={{ width: 100, height: 100, backgroundColor: 'blue' }} animate={{ x: 200 }} transition={{ duration: 0.5 }} /> ); }; export default App;
In this example:
- The
motion.div
is used to create an animateddiv
. - The
animate
prop specifies the target animation state. - The
transition
prop controls the animation duration.
Adding Variants
Variants allow you to define multiple animation states and switch between them:
const boxVariants = { hidden: { opacity: 0, x: -100 }, visible: { opacity: 1, x: 0 }, }; const App = () => { return ( <motion.div variants={boxVariants} initial="hidden" animate="visible" transition={{ duration: 0.8 }} style={{ width: 100, height: 100, backgroundColor: 'red' }} /> ); };
Here:
boxVariants
defines two states:hidden
andvisible
.- The
initial
andanimate
props specify which variant to use.
Using AnimatePresence
To animate elements as they enter or leave the DOM, use AnimatePresence
:
import { motion, AnimatePresence } from 'framer-motion'; import React, { useState } from 'react'; const App = () => { const [isVisible, setIsVisible] = useState(true); return ( <div> <button onClick={() => setIsVisible(!isVisible)}>Toggle</button> <AnimatePresence> {isVisible && ( <motion.div initial={{ opacity: 0 }} animate={{ opacity: 1 }} exit={{ opacity: 0 }} transition={{ duration: 0.5 }} style={{ width: 100, height: 100, backgroundColor: 'green' }} /> )} </AnimatePresence> </div> ); }; export default App;
Best Practices
- Keep It Simple: Start with basic animations and gradually add complexity.
- Reuse Variants: Define reusable variants for consistency.
- Combine with CSS: Use CSS for static styles and Framer Motion for dynamic animations.
- Optimize Performance: Avoid animating unnecessary elements or using heavy transitions.
FAQs
Use npm install framer-motion
or yarn add framer-motion
.
It handles animations for elements entering and exiting the DOM.
Yes, use CSS for static styles and Framer Motion for dynamic animations.
Conclusion
Framer Motion is a powerful tool for creating polished animations in React applications. Its simple API and extensive features make it a go-to choice for developers aiming to enhance their user interfaces. Start experimenting with Framer Motion today to bring your designs to life!
We are Leapcell, your top choice for deploying Node.js projects to the cloud.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ