How to Use Python Regex for String Replacement
Grace Collins
Solutions Engineer · Leapcell
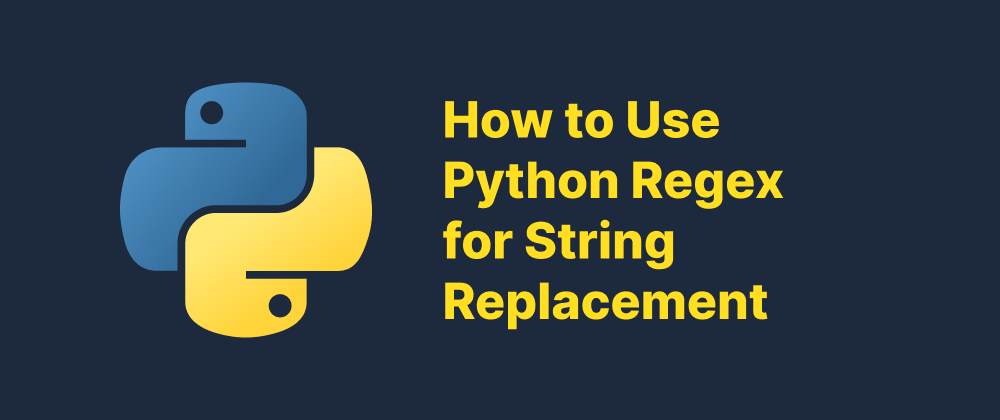
Key Takeaways
- Python's
re.sub()
allows flexible pattern-based string replacement. - Grouping and backreferences help restructure matched content.
- Functions can be used for dynamic, custom replacements.
Regular expressions (regex) are powerful tools for searching and manipulating strings. In Python, the re
module allows developers to perform regex-based search and replace operations efficiently. This article explains how to use Python regex to replace substrings with re.sub()
.
Importing the re
Module
To use regex in Python, first import the built-in re
module:
import re
Basic Usage of re.sub()
The main function used for regex replacement is re.sub(pattern, repl, string)
. It searches for all non-overlapping matches of the pattern
in the string
and replaces them with the string repl
.
Example 1: Replacing Digits with a Symbol
import re text = "Order number: 12345" result = re.sub(r'\d', '*', text) print(result)
Output:
Order number: *****
Explanation: The pattern \d
matches any digit, and all digits are replaced with *
.
Using Groups in Replacement
You can capture parts of the match using parentheses ()
and refer to them in the replacement string using \1
, \2
, etc.
Example 2: Reformatting Dates
text = "Today is 2025-04-09" result = re.sub(r'(\d{4})-(\d{2})-(\d{2})', r'\2/\3/\1', text) print(result)
Output:
Today is 04/09/2025
Explanation: The regex captures the year, month, and day separately and rearranges them in MM/DD/YYYY format.
Using a Function for Dynamic Replacement
If you want more control over how matches are replaced, you can pass a function as the repl
argument.
Example 3: Incrementing Numbers
def add_one(match): number = int(match.group()) return str(number + 1) text = "Levels: 1, 2, 3" result = re.sub(r'\d+', add_one, text) print(result)
Output:
Levels: 2, 3, 4
Explanation: The function takes each number and increments it by 1.
Flags and Case-Insensitive Replacement
The re.sub()
function accepts a flags
parameter for modifiers like case insensitivity.
Example 4: Case-Insensitive Replacement
text = "Hello hello HeLLo" result = re.sub(r'hello', 'hi', text, flags=re.IGNORECASE) print(result)
Output:
hi hi hi
Conclusion
Python's re.sub()
is a versatile function that supports simple replacements, group-based reordering, and even dynamic substitution using functions. With proper use of regex patterns, you can perform powerful text processing tasks in just a few lines of code.
FAQs
The re.sub()
function is used to perform regex-based replacements.
Yes, by passing a function to re.sub()
, you can transform each match programmatically.
Use capturing groups and refer to them in the replacement string using \1
, \2
, etc.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ