Understanding Operator Overloading in Python
Daniel Hayes
Full-Stack Engineer · Leapcell
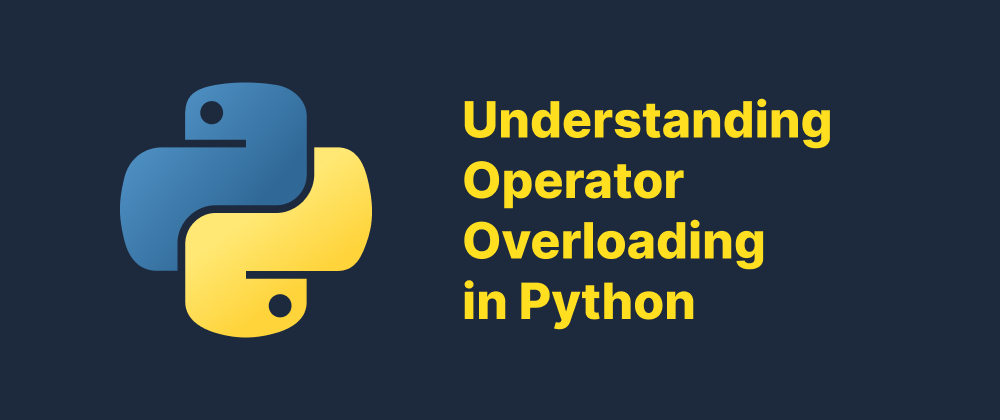
Key Takeaways
- Python allows user-defined classes to customize built-in operator behavior via special methods.
- Operator overloading improves code readability and enables intuitive syntax for custom objects.
- Common operators like
+
,-
, and==
can be mapped to methods such as__add__
,__sub__
, and__eq__
.
Python is well-known for its simplicity and readability, but it also offers powerful features that enable developers to write intuitive and expressive code. One such feature is operator overloading, which allows custom objects to interact using standard operators like +
, -
, *
, and more. This article explores what operator overloading is, why it's useful, and how to implement it in Python.
What Is Operator Overloading?
Operator overloading refers to the ability to redefine the behavior of built-in operators for user-defined classes. In other words, you can "teach" Python how to evaluate expressions like a + b
when a
and b
are instances of a custom class.
Python achieves this by mapping operators to special methods, also known as dunder methods (short for “double underscore”). For example:
+
corresponds to__add__
-
corresponds to__sub__
*
corresponds to__mul__
/
corresponds to__truediv__
==
corresponds to__eq__
<
corresponds to__lt__
By defining these methods in your class, you can control how objects behave when used with those operators.
Why Use Operator Overloading?
Operator overloading improves code readability and usability, especially for mathematical or data-structure-heavy applications. Instead of writing verbose method calls, you can use natural and familiar syntax.
For example, consider a class Vector
that represents a two-dimensional vector. Without operator overloading:
v1.add(v2)
With operator overloading:
v1 + v2
The latter is not only shorter but also more intuitive for anyone familiar with basic math.
Example: Overloading the +
Operator
Here is a simple example of overloading the +
operator in a Vector
class:
class Vector: def __init__(self, x, y): self.x = x self.y = y def __add__(self, other): return Vector(self.x + other.x, self.y + other.y) def __repr__(self): return f"Vector({self.x}, {self.y})" v1 = Vector(2, 3) v2 = Vector(4, 1) print(v1 + v2) # Output: Vector(6, 4)
List of Common Overloadable Operators
Here are some commonly used operator methods and their meanings:
Operator | Method Name | Description |
---|---|---|
+ | __add__ | Addition |
- | __sub__ | Subtraction |
* | __mul__ | Multiplication |
/ | __truediv__ | Division |
// | __floordiv__ | Floor Division |
% | __mod__ | Modulo |
** | __pow__ | Exponentiation |
== | __eq__ | Equal to |
!= | __ne__ | Not equal to |
< | __lt__ | Less than |
<= | __le__ | Less than or equal to |
> | __gt__ | Greater than |
>= | __ge__ | Greater than or equal to |
[] | __getitem__ | Indexing |
in | __contains__ | Membership test |
() | __call__ | Callable objects |
Best Practices
- Keep operations intuitive: Only overload operators where it makes logical sense.
- Return new instances: Avoid mutating existing instances unless that's the intended behavior.
- Ensure compatibility: Always handle edge cases and validate types when performing operations.
Conclusion
Operator overloading in Python allows developers to write cleaner, more expressive code by customizing how objects interact with built-in operators. It's especially useful in mathematical, graphical, and data-rich applications. However, like any powerful feature, it should be used judiciously to maintain code clarity and integrity.
FAQs
Operator overloading allows more natural and readable expressions, especially in mathematical or domain-specific code.
No, Python supports overloading for comparisons, indexing, membership tests, and even function calls.
No, operator overloading applies only to user-defined classes; built-in types remain unchanged.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ