Getting Started with Redis and Python
Grace Collins
Solutions Engineer · Leapcell
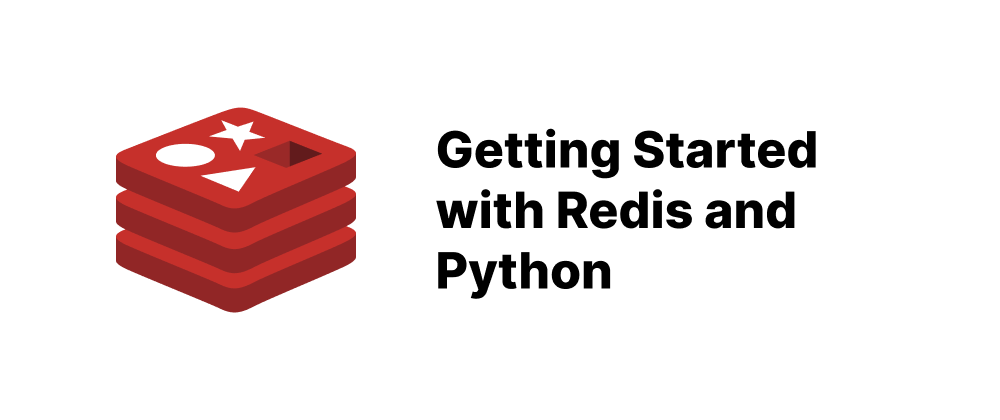
Key Takeaways
- Redis is a high-speed, in-memory data store ideal for caching and real-time processing.
- Python’s
redis-py
library simplifies integrating Redis into Python applications. - Installing and connecting to Redis with Python requires minimal setup.
Redis is a high-performance, in-memory key-value store widely used for caching, real-time analytics, and message brokering. When combined with Python, it becomes a powerful tool for building scalable and efficient applications. This guide introduces Redis and demonstrates how to use it with Python through the redis-py
client library. (Python Redis: A Beginner's Guide - DataCamp)
What Is Redis?
Redis (Remote Dictionary Server) is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It supports various data structures such as strings, hashes, lists, sets, and sorted sets. Redis is known for its speed and flexibility, making it suitable for a wide range of applications. (Python Redis: A Beginner's Guide - DataCamp)
Why Use Redis with Python?
Python's simplicity and extensive ecosystem make it an excellent choice for integrating with Redis. Using Redis with Python allows developers to:
- Implement efficient caching mechanisms to speed up applications.
- Manage real-time data processing and analytics.
- Build scalable systems with features like pub/sub messaging and distributed locking. (Python Redis: A Beginner's Guide - DataCamp)
Setting Up Redis
Installing Redis Server
To get started, you need to have Redis installed on your system. You can install Redis using various methods depending on your operating system. For example, on macOS with Homebrew:
brew install redis
On Linux systems, you can use your package manager:
sudo apt-get update sudo apt-get install redis-server
After installation, start the Redis server: (Python Redis: A Beginner's Guide - DataCamp)
redis-server
Installing redis-py
Library
The redis-py
library is the official Python client for Redis. Install it using pip: (ython redisRedis Simplified: A Concise Tutorial on Redis with Python, Beginner's Guide to Redis with Python - AskPython)
pip install redis
For improved performance, you can install it with hiredis support: (redis/redis-py: Redis Python client - GitHub)
pip install "redis[hiredis]"
Connecting to Redis with Python
Here's how to establish a connection to the Redis server using Python:
import redis # Connect to Redis server r = redis.Redis(host='localhost', port=6379, db=0)
You can test the connection by setting and getting a value: (Redis)
r.set('name', 'Alice') print(r.get('name')) # Output: b'Alice'
To automatically decode responses to strings: (redis/redis-py: Redis Python client - GitHub)
r = redis.Redis(host='localhost', port=6379, db=0, decode_responses=True) r.set('name', 'Alice') print(r.get('name')) # Output: Alice
Working with Redis Data Structures
Strings
Strings are the most basic Redis data type. (ython redisRedis Simplified: A Concise Tutorial on Redis with Python)
r.set('language', 'Python') print(r.get('language')) # Output: Python
Hashes
Hashes are maps between string field and string values, perfect for representing objects. (Beginner's Guide to Redis with Python - AskPython)
r.hset('user:1000', 'name', 'Alice') r.hset('user:1000', 'email', 'alice@example.com') print(r.hgetall('user:1000')) # Output: {'name': 'Alice', 'email': 'alice@example.com'}
Lists
Lists are ordered collections of strings. (Beginner's Guide to Redis with Python - AskPython)
r.rpush('tasks', 'task1') r.rpush('tasks', 'task2') print(r.lrange('tasks', 0, -1)) # Output: ['task1', 'task2']
Sets
Sets are unordered collections of unique strings.
r.sadd('tags', 'python') r.sadd('tags', 'redis') print(r.smembers('tags')) # Output: {'python', 'redis'}
Sorted Sets
Sorted sets are like sets but where every string element is associated with a score. (Beginner's Guide to Redis with Python - AskPython)
r.zadd('leaderboard', {'Alice': 100, 'Bob': 95}) print(r.zrange('leaderboard', 0, -1, withscores=True)) # Output: [('Bob', 95.0), ('Alice', 100.0)]
Advanced Features
Pipelines
Pipelines allow you to batch multiple commands to reduce the number of round-trip times between the client and server. (Beginner's Guide to Redis with Python - AskPython)
pipe = r.pipeline() pipe.set('foo', 'bar') pipe.set('baz', 'qux') pipe.execute()
Pub/Sub
Redis supports Publish/Subscribe messaging paradigms.
pubsub = r.pubsub() pubsub.subscribe('channel1') # In a separate thread or process r.publish('channel1', 'Hello, Redis!')
Transactions
Redis transactions ensure that a group of commands are executed atomically. (Beginner's Guide to Redis with Python - AskPython)
with r.pipeline() as pipe: while True: try: pipe.watch('balance') balance = int(pipe.get('balance')) if balance >= 50: pipe.multi() pipe.set('balance', balance - 50) pipe.execute() break else: pipe.unwatch() break except redis.WatchError: continue
Best Practices
- Use Connection Pools: Manage multiple connections efficiently using Redis connection pools.
- Set Expiration: Use key expiration to prevent stale data.
- Handle Exceptions: Implement proper error handling for network issues and command errors.
- Monitor Performance: Regularly monitor Redis performance metrics to identify bottlenecks. (redis/redis-py: Redis Python client - GitHub, Beginner's Guide to Redis with Python - AskPython)
Conclusion
Integrating Redis with Python provides a robust solution for building high-performance applications. Whether you're implementing caching, real-time analytics, or messaging systems, Redis offers the tools needed to enhance your application's efficiency and scalability. (Beginner's Guide to Redis with Python - AskPython, Python Redis: A Beginner's Guide - DataCamp)
References
- DataCamp: Python Redis: A Beginner's Guide
- Redis Official Documentation
- redis-py GitHub Repository
- AskPython: Beginner's Guide to Redis with Python
FAQs
Use a package manager like Homebrew (brew install redis
) or APT (sudo apt-get install redis-server
).
The official client is redis-py
, which you can install with pip install redis
.
It enables fast data access, caching, and real-time messaging with minimal overhead.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ