React vs Svelte: Side-by-Side Feature Analysis
Olivia Novak
Dev Intern · Leapcell
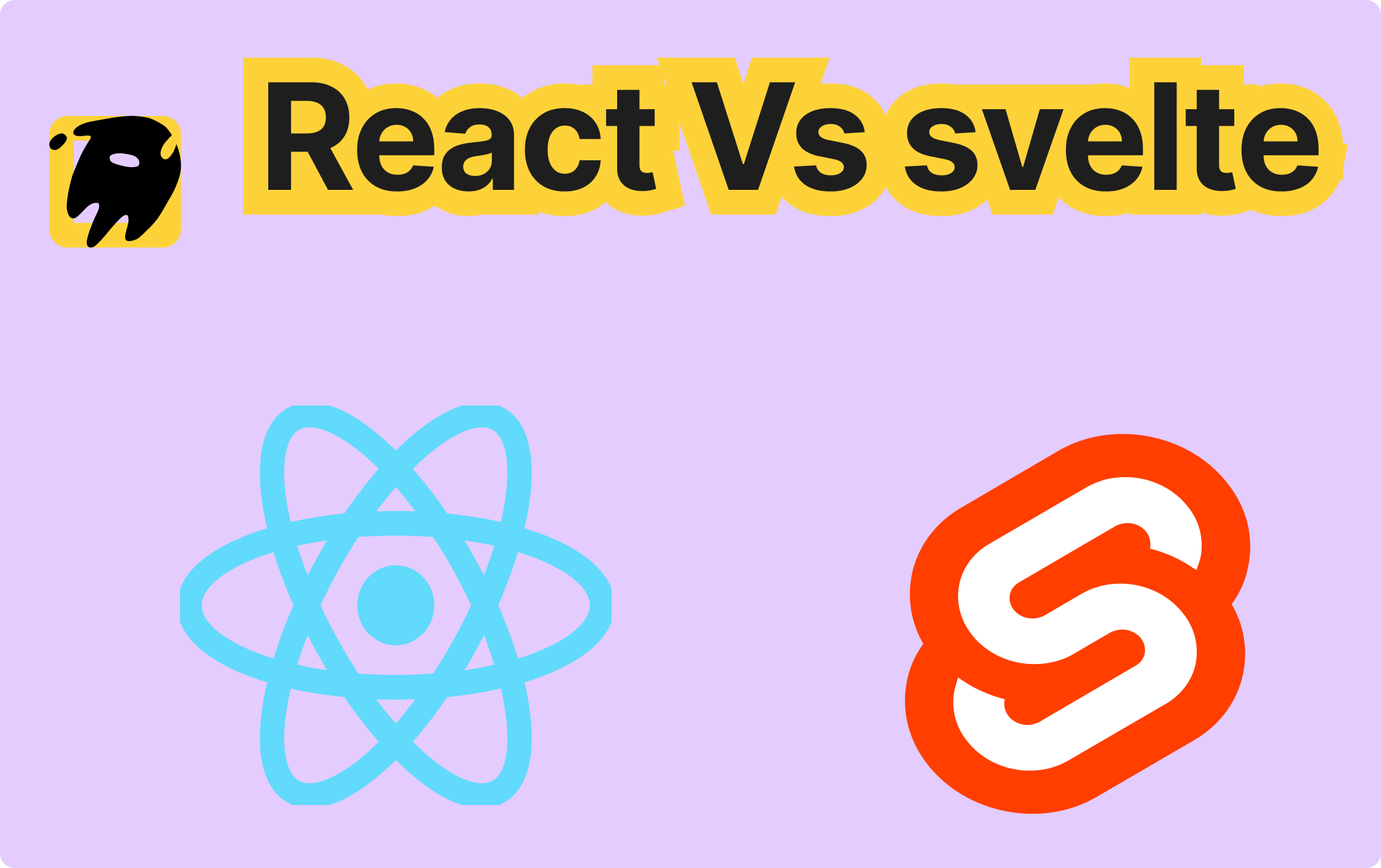
Comparison between React and Svelte Frameworks: From Rendering Modes to Functional Implementations
Like React, Svelte is a reactive UI framework used for building front-end interfaces, which enables developers to organize page code in a component-based manner. I recently watched a video on YouTube that compared the differences between the React and Svelte frameworks through ten practical examples. The content is extremely interesting and valuable for learning. Therefore, this article will organize the content in the video and add my own understanding to provide a reference for everyone and help you gain a deeper understanding of these two frameworks.
Comparison Item | React | Svelte |
---|---|---|
Rendering Mode | Calculates the parts of the page that need to be changed through the Virtual DOM. It requires a built-in Runtime, and the code size is relatively large (for example, a Hello World application in Next.js has about 70kb of JavaScript code). | Compiles the code during the build process. It uses a compiler instead of a runtime, and the final product does not contain Svelte library code. The code size is small (for example, a Hello World application is only 3kb). |
state | Uses useState to generate a reactive state and a setter function. Calling the setter triggers the UI to re-render. | Variables declared with let are reactive. When the value of the variable is changed, the framework automatically updates the UI. |
props | In functional components, properties are received as function parameters, and destructuring assignment is commonly used to obtain the property values. Properties can be a component. | Adding the export keyword when declaring a variable indicates that it is an externally passed property. It provides a syntactic sugar {property name} to pass properties, and properties cannot be components. |
children | Obtains the information of child components through props.children . | Implemented through slots slot , supporting default slots and named slots. |
Lifecycle | In functional components, the lifecycle is simulated through useEffect (for example, returning a function in useEffect is used for component unmounting). | Lifecycle functions such as onMount and onDestroy are imported in the script . |
Side Effects | Declares side effects through useEffect , and the variables on which the side effects depend need to be declared manually. | Declares side effects with a reactive expression starting with the $ symbol, and there is no need to explicitly declare the dependent variables. |
Computed Properties | Uses useMemo to create computed properties. The first parameter is a function that returns the value of the computed property, and the second parameter is the dependency array. | Uses the $ expression to create computed properties, which are automatically updated when the dependent variables change. |
Conditional Rendering | Uses JavaScript's ternary expression to express the conditional rendering logic. | Adopts a syntax similar to traditional template languages ({#if} {:else if} {:else} {/if} ), which is clearer for complex logic. |
Looping | Traverses the array using map and returns components to achieve loop rendering, and a key needs to be set. | Performs loop rendering through each , and (variable.id) represents the key of the rendering. |
Global State Management | Creates a Context through createContext , wraps child components with a Provider in the root component, and child components use useContext to obtain the state. | Uses writable to declare a global store. In components, $+variable name is used to read it, and store.update() is used to update it. The syntax is more concise. |
Asynchronous Rendering | React18 introduced the use hook to execute asynchronous code. Asynchronous components are wrapped in Suspense and can be used with ErrorBoundary to handle loading and errors. | Provides a template syntax similar to JavaScript ({#await} {:then} {:catch} {/await} ) to handle asynchronous rendering and error catching. |
0. Rendering Mode
Although both React and Svelte are reactive UI frameworks, their rendering modes are completely different. React calculates the parts of the page that need to be changed by means of the Virtual DOM. This means that every React application needs to have a built-in Runtime, that is, it contains some code for calculating the Virtual DOM and rendering the page. This will lead to an increase in the code size. For example, a Hello World application built with Next.js has 70kb of JavaScript code.
Svelte adopts a completely different strategy. During the application build stage, Svelte will compile the code written by developers, using a compiler instead of a runtime. The final generated product does not contain any Svelte library code. Therefore, a Svelte Hello World application is only 3kb.
Although Svelte compiles non-JS code into JS code, while the code of a React application is pure JS code, surprisingly, Svelte can work better with third-party libraries of native JavaScript. However, React has a more mature ecosystem.
1. state
First, compare the ways to achieve the simplest state management in the two frameworks.
In React, you need to use useState
to generate a reactive state count
and its corresponding setter function setCount()
. When calling setCount()
to update the value of count
, it will trigger the UI to re-render.
import { useState } from "react"; function Counter() { // Use useState to initialize the state count to 0 and get the update function setCount const [count, setCount] = useState(0); return ( <div> {/* When clicking the button, call setCount to increase the value of count and display the current count value */} <button onClick={() => setCount(count + 1)}>Count is {count}</button> </div> ); }
In Svelte, as long as a variable is declared with the let
keyword, it is reactive. A reactive variable count
is declared in the Svelte component code. The Svelte component code is divided into three parts: script
, style
, and template
. The difference is that in Svelte, there is no need to wrap the HTML in a template
tag. To change the value of count
, you just need to operate it like an ordinary variable, and the framework will automatically perform a reactive UI update.
<script> // Declare the reactive variable count and initialize it to 0 let count = 0; </script> {#if true} <!-- When clicking the button, increase the value of count and display the current count value --> <button on:click={() => count++}> count is {count} </button> {/if}
2. props
Next, let's see how to receive and pass properties in the two frameworks. In React's functional components, properties are received in the form of function parameters, and the destructuring assignment method is usually used to obtain the specific values of the properties.
function ColoredBox({color}) { // Use destructuring assignment to get the value of the color property and display it return ( <p>You picked: {color}</p> ) }
In Svelte, when declaring a variable, adding the export
keyword in front of it means that the variable is an externally passed property.
<script> // Declare color as an externally passed property export let color; </script> {#if color} You picked: {color} {/if}
In terms of variable passing, the syntax of the two is similar, both in the form of HTML attributes:
<App color={color} />
Svelte also provides a syntactic sugar to make property passing more concise:
<App {color} />
It should be noted that React's properties can be a component, while Svelte does not support this way.
<App head={<Head />} />
3. children
In React, you can obtain the information of child components through props.children
.
function ParentComponent(props) { // Get the content of child components through props.children and display it return ( <div> {props.children} </div> ); }
In Svelte, this function needs to be implemented through the slot slot
.
<!-- Widget.svelte --> <div> <slot> <!-- If there is no content of child components, this content will be displayed by default --> If there is no content of child components, this content will be displayed by default. </slot> </div> <!-- App.svelte --> <Widget /> <!-- ⬆️This component will display the default content --> <Widget> <p>This child component will overwrite the default content</p> </Widget>
Svelte also supports named slots:
<!-- Widget.svelte --> <div> <slot name="header" /> <p>Content between the header and the footer</p> <slot name="footer" /> </div> <!-- App.svelte --> <Widget> <h1 slot="header">Hello</h1> <p slot="footer">Svelte Industries</p> </Widget>
4. Lifecycle
In React's functional components, the lifecycle needs to be simulated through useEffect
.
useEffect(() => { // Executed when the component is initialized, equivalent to onMount console.log('Component initialized'); return () => { // Executed when the component is unmounted, equivalent to onDestroy console.log('Component unmounted'); } }, [])
In Svelte, you just need to import the corresponding lifecycle functions in the script
.
<script> import { onMount, onDestroy } from 'svelte'; onMount(() => { console.log('Component mounted'); }); onDestroy(() => { console.log('Component unmounted'); }); </script>
5. Side Effects
In React, side effects are declared through useEffect
, and the variables on which the side effects depend are declared manually through the second parameter of useEffect
.
function Counter() { const [count] = useState(0); useEffect(() => { // When count changes, update document.title document.title = `count is ${count}`; }, [count]) }
In Svelte, side effects can be declared through a reactive expression starting with the $
symbol.
<script> let count = 0; // When count changes, automatically update document.title $: document.title = `count is ${count}`; </script>
The statement after $:
will automatically have reactive functionality. When the variable referenced in the statement changes, the statement will automatically run, which is equivalent to the side effect of the variable change. In comparison, Svelte does not need to explicitly declare the variables on which the side effect statement depends, and it is more convenient to use than React.
6. Computed Properties
Computed properties refer to variables whose values depend on the state
, similar to computed
in Vue. In React, computed properties can be created through useMemo
. The first parameter of useMemo
is a function, and its return value is the value of the computed property; the second parameter is the dependency array. When the variables in the dependency array change, the value of the computed property will be recalculated.
function Counter() { const [count] = useState(0); // Use useMemo to create a computed property double, which depends on count const double = useMemo(() => count * 2, [count]); }
In Svelte, the $
expression mentioned in the previous section can also be used to create computed properties.
<script> let count = 0; // Create a computed property doubled, which depends on count. When count changes, doubled will be reassigned $: doubled = count * 2 </script>
7. Conditional Rendering
Since React uses JSX to describe the UI, JavaScript's ternary expression can be used to implement the logic of conditional rendering.
function Counter() { const [count] = useState(0); return <> {/* Conditional rendering according to the value of count */} { count > 1000 ? <p>Big</p> : <p>Small</p> } </> }
Svelte uses a syntax similar to traditional template languages to express the logic of conditional rendering.
<script> let count = 0 </script> {#if count > 1000} <p>Big</p> {:else if count > 500} <p>Medium</p> {:else} <p>Small</p> {/if}
In comparison, although Svelte's syntax is a bit more cumbersome, due to the existence of the else if
statement, it is clearer than React's ternary expression when expressing complex conditional rendering logic.
8. Looping
In React, you can use map
to traverse an array and return a series of components to achieve loop rendering.
function Looper() { const items = [ { id: 1, name: "foo" }, { id: 2, name: "bar" }, { id: 3, name: "baz" } ]; return <> {/* Use map to traverse the items array, render components and set the key */} {items.map(item => <p key={item.id}>{item.name}</p>)} </> }
In Svelte, loop rendering can be performed through each
, where (item.id)
indicates that the key
of the rendering is item.id
.
<script> const items = [ { id: 1, name: "foo" }, { id: 2, name: "bar" }, { id: 3, name: "baz" } ]; </script> {#each items as item (item.id)} <p>{item.name}</p> {/each}
9. Global State Management
In React, if you want to create a state shared by multiple components, it can be achieved through Context
. First, use createContext
to create a CountContext
, and then use the Provider
of this Context
to wrap the child components in the root component App
. In this way, in the child component Counter
, you can obtain the content in the CountContext
through useContext
.
// context.js import { createContext } from 'react'; // Create CountContext and initialize it to 0 export const CountContext = createContext(0); // Counter.jsx import { useContext } from 'react'; import { CountContext } from './context'; function Counter() { // Use useContext to get the value in CountContext const count = useContext(CountContext); return <div>{count}</div>; } // App.jsx import { CountContext } from './context'; import { Counter } from './Counter'; function App() { return <> {/* Use CountContext.Provider to wrap child components and pass the state */} <CountContext.Provider value={42}> <Counter /> </CountContext.Provider> </>; }
In Svelte, a global store can be declared through writable
. Import the store in the component that needs to use the global state, read the store through the way of $+variable name
, and call store.update()
to update the store.
// store.js import { writable } from "svelte/store"; // Declare the global store count and initialize it to 0 export const count = writable(0); // App.svelte <script> import { count } from "./store"; </script> <button onClick={() => count.update(c => c + 1)}> {/* Use $count to read the value of the global state count */} {$count} </button>
Personally, I think that Svelte's global state management syntax is more concise. There is no need to write Provider
, and you can use the global state just with a $
symbol.
10. Asynchronous Rendering
In React18, an asynchronous rendering mechanism was introduced. You can use the new use
hook to execute asynchronous code, and its effect is similar to the await
statement. A component that uses use
is an asynchronous component. Since it needs to wait for the asynchronous code to be executed before the component can be rendered.
function AsyncComponent() { const number = use(Promise.resolve(100)); return <p>{number}</p>; }
When using an asynchronous component, it can be wrapped in a Suspense
component and a fallback
component can be passed in. The fallback
component will be displayed when the asynchronous component has not been rendered yet, which is used to add a loading state. In addition, to prevent errors from occurring during the asynchronous rendering process, ErrorBoundary
can also be used to catch errors, and the corresponding fallback
component will be displayed when an error occurs to avoid the page crashing and going blank.
function App() { return ( <ErrorBoundary fallback={<ErrorPage />}> <Suspense fallback={<LoadingSpinner/>}> <ComponentWithAsyncData /> </Suspense> </ErrorBoundary> ) }
In Svelte, the framework provides a template syntax similar to JavaScript to meet the needs of asynchronous rendering and error catching.
<script> const promise = Promise.resolve(69); </script> {#await promise} <LoadingSpinner/> {:then number} <p>The number is {number}</p> {:catch error} <ErrorPage {error} /> {/await}
Summary
This article has made a detailed comparison between the React and Svelte frameworks from ten aspects, including rendering mode, state management, property passing, child component handling, lifecycle, side effects, computed properties, conditional rendering, looping, global state management, and asynchronous rendering, covering their basic usage methods. It is believed that after reading this article, readers have gained a more comprehensive understanding of Svelte. These two UI frameworks each have their own advantages. Which one do you appreciate more? You are welcome to share your views in the comment section.
References:
- https://www.youtube.com/watch?v=MnpuK0MK4yo
- https://fireship.io/lessons/svelte-for-react-developers/
Leapcell: The Best of Serverless Web Hosting
Finally, I would like to recommend a platform that is most suitable for deploying nodejs services: Leapcell
🚀 Build with Your Favorite Language
Develop effortlessly in JavaScript, Python, Go, or Rust.
🌍 Deploy Unlimited Projects for Free
Only pay for what you use—no requests, no charges.
⚡ Pay-as-You-Go, No Hidden Costs
No idle fees, just seamless scalability.
🔹 Follow us on Twitter: @LeapcellHQ