Redis Commands: A Comprehensive Overview
Grace Collins
Solutions Engineer · Leapcell
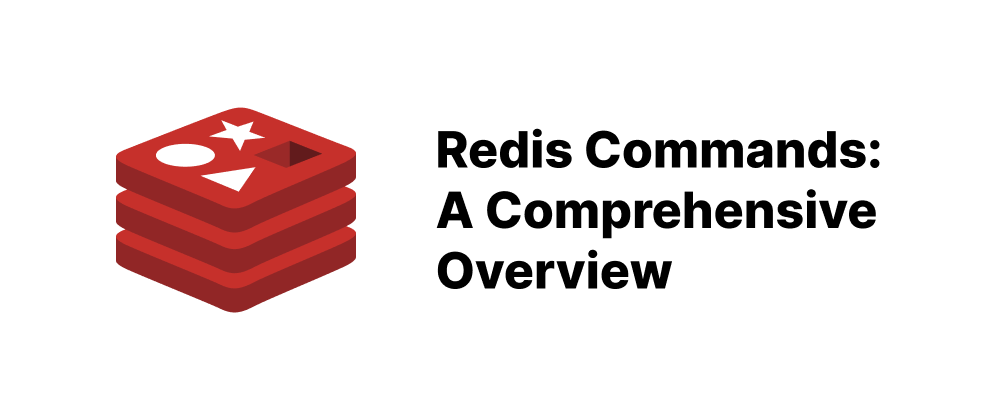
Key Takeaways
- Redis offers specialized commands for different data types like strings, lists, sets, hashes, and sorted sets.
- Effective key management and transactions are crucial for optimizing Redis performance and data integrity.
- Understanding core commands enables efficient data storage, retrieval, and manipulation in Redis.
Redis is an open-source, in-memory data structure store, widely used as a database, cache, and message broker. It offers a rich set of commands tailored to its diverse data types, enabling efficient data manipulation and retrieval. This guide provides an overview of essential Redis commands, categorized by data types and functionalities.
1. String Commands
Strings are the most basic Redis data type, capable of storing text or binary data up to 512 MB. (Complete Guide to Redis Commands | GeeksforGeeks)
- SET key value: Assigns a value to a key.
SET mykey "Hello"
- GET key: Retrieves the value of a key.
GET mykey
- DEL key [key ...]: Deletes one or more keys.
DEL mykey
- EXISTS key: Checks if a key exists.
EXISTS mykey
- INCR key / DECR key: Increments or decrements the integer value of a key.
INCR counter DECR counter
- APPEND key value: Appends a value to an existing key.
APPEND mykey " World"
- TTL key: Returns the remaining time to live of a key in seconds.
TTL mykey
- EXPIRE key seconds: Sets a key's time to live in seconds.
EXPIRE mykey 60
(Complete Guide to Redis Commands | GeeksforGeeks, Redis Basic Commands Tutorial - KoderHQ)
2. List Commands
Redis lists are ordered collections of strings, implemented as linked lists. (Redis Lists - Tutorialspoint)
- LPUSH key value [value ...]: Prepends one or multiple values to a list.
LPUSH mylist "World" LPUSH mylist "Hello"
- RPUSH key value [value ...]: Appends one or multiple values to a list.
RPUSH mylist "!"
- LRANGE key start stop: Retrieves a range of elements from a list.
LRANGE mylist 0 -1
- LPOP key / RPOP key: Removes and returns the first or last element of a list.
LPOP mylist RPOP mylist
- LLEN key: Returns the length of a list.
LLEN mylist
- LSET key index value: Sets the list element at index to value.
LSET mylist 0 "Hi"
- LREM key count value: Removes elements equal to value from the list.
LREM mylist 1 "Hello"
- LTRIM key start stop: Trims a list to the specified range.
LTRIM mylist 0 1
(Redis Lists - Tutorialspoint)
3. Set Commands
Sets are unordered collections of unique strings.
- SADD key member [member ...]: Adds one or more members to a set.
SADD myset "Hello" "World"
- SMEMBERS key: Returns all members of the set.
SMEMBERS myset
- SISMEMBER key member: Determines if a given value is a member of the set.
SISMEMBER myset "Hello"
- SREM key member [member ...]: Removes one or more members from a set.
SREM myset "Hello"
- SCARD key: Returns the set cardinality (number of elements).
SCARD myset
- SUNION key [key ...]: Returns the union of all given sets.
SUNION set1 set2
- SINTER key [key ...]: Returns the intersection of all given sets.
SINTER set1 set2
- SDIFF key [key ...]: Returns the difference between the first set and all the successive sets.
SDIFF set1 set2
(Commands | Docs - Redis, Redis Cheat Sheet & Quick Reference)
4. Hash Commands
Hashes are maps between string field and string values, ideal for representing objects. (Redis Commands - Tutorialspoint)
- HSET key field value [field value ...]: Sets field in the hash stored at key to value.
HSET user:1000 name "John" age "30"
- HGET key field: Gets the value of a field in the hash.
HGET user:1000 name
- HMGET key field [field ...]: Gets the values of all specified fields.
HMGET user:1000 name age
- HGETALL key: Gets all fields and values in the hash.
HGETALL user:1000
- HDEL key field [field ...]: Deletes one or more hash fields.
HDEL user:1000 age
- HEXISTS key field: Determines if a hash field exists.
HEXISTS user:1000 name
- HLEN key: Returns the number of fields in the hash.
HLEN user:1000
5. Sorted Set (ZSet) Commands
Sorted sets are similar to sets but where every member has an associated score, allowing sorted retrieval.
- ZADD key score member [score member ...]: Adds one or more members to a sorted set, or updates its score if it already exists.
ZADD leaderboard 100 "Player1" 200 "Player2"
- ZRANGE key start stop [WITHSCORES]: Returns a range of members in a sorted set, by index.
ZRANGE leaderboard 0 -1 WITHSCORES
- ZREVRANGE key start stop [WITHSCORES]: Returns a range of members in a sorted set, by index, with scores ordered from high to low.
ZREVRANGE leaderboard 0 -1 WITHSCORES
- ZSCORE key member: Returns the score of a member in the sorted set.
ZSCORE leaderboard "Player1"
- ZREM key member [member ...]: Removes one or more members from a sorted set.
ZREM leaderboard "Player1"
- ZCARD key: Returns the number of members in a sorted set.
ZCARD leaderboard
- ZRANK key member: Determines the index of a member in a sorted set.
ZRANK leaderboard "Player2"
6. Key Management Commands
These commands are used to manage keys in Redis.
- KEYS pattern: Finds all keys matching the given pattern.
KEYS user:*
- SCAN cursor [MATCH pattern] [COUNT count]: Incrementally iterates the keys space.
SCAN 0 MATCH user:*
- RENAME key newkey: Renames a key.
RENAME oldkey newkey
- EXPIRE key seconds: Sets a timeout on a key.
EXPIRE mykey 60
- TTL key: Returns the remaining time to live of a key.
TTL mykey
- TYPE key: Returns the data type of the value stored at key.
TYPE mykey
(Complete Guide to Redis Commands | GeeksforGeeks)
7. Transaction Commands
Redis supports transactions through a group of commands that are executed atomically.
- MULTI: Marks the start of a transaction block.
MULTI
- EXEC: Executes all commands issued after MULTI.
EXEC
- DISCARD: Flushes all previously queued commands in a transaction.
DISCARD
- WATCH key [key ...]: Watches the given keys to determine execution of the MULTI/EXEC block.
FAQs
Commands like KEYS
, EXPIRE
, TTL
, and RENAME
help manage Redis keys efficiently.
Redis uses MULTI
, EXEC
, WATCH
, and DISCARD
commands to ensure atomic execution of grouped commands.
Sorted sets store unique elements with scores, allowing ordered retrieval based on scores.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ