Understanding Anonymous Structs in Golang
Grace Collins
Solutions Engineer · Leapcell
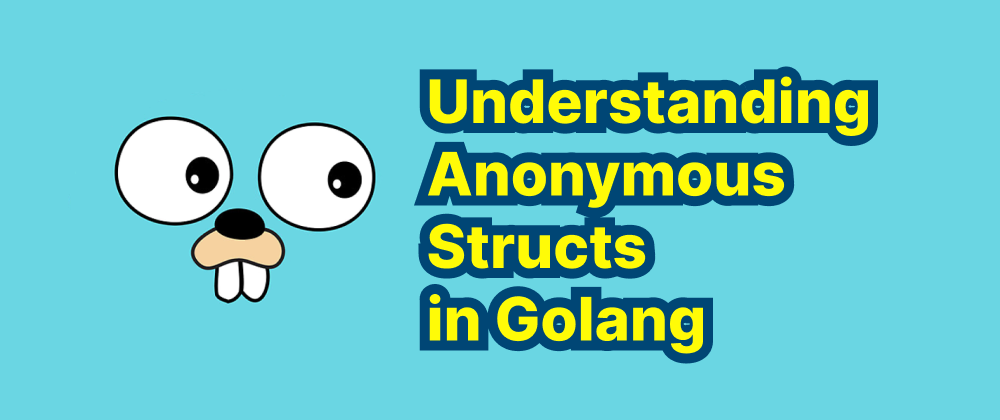
Key Takeaways
- Anonymous structs in Go are ideal for defining temporary, one-off data structures.
- They are particularly useful in scenarios like JSON marshalling and table-driven tests.
- Overusing anonymous structs can impact code readability and reusability.
In Go (Golang), an anonymous struct is a struct type defined without a name, allowing for quick, one-off data structures without the need for a formal type declaration. This feature is particularly useful when a specific struct is only relevant within a limited scope, promoting cleaner and more concise code.
Defining Anonymous Structs
To create an anonymous struct, you define and instantiate it simultaneously. Here's an example:
newCar := struct { make string model string mileage int }{ make: "Ford", model: "Taurus", mileage: 200000, }
In this snippet, newCar
is an instance of an anonymous struct with fields make
, model
, and mileage
. Since the struct has no name, it's confined to the scope where it's defined and cannot be reused elsewhere without redefining its structure.
Use Cases for Anonymous Structs
1. JSON Marshalling and Unmarshalling
Anonymous structs are particularly handy when dealing with JSON data in HTTP handlers. They allow for direct unmarshalling of JSON payloads into struct fields without the need for predefined types. Here's how you might use an anonymous struct to unmarshal JSON data:
func createCarHandler(w http.ResponseWriter, req *http.Request) { defer req.Body.Close() decoder := json.NewDecoder(req.Body) newCar := struct { Make string `json:"make"` Model string `json:"model"` Mileage int `json:"mileage"` }{} err := decoder.Decode(&newCar) if err != nil { log.Println(err) return } makeCar(newCar.Make, newCar.Model, newCar.Mileage) }
In this function, the JSON payload is decoded directly into the newCar
anonymous struct, streamlining the process and keeping the scope limited to where it's needed.
2. Table-Driven Tests
Anonymous structs are also beneficial in writing table-driven tests, a common testing pattern in Go. They allow for concise definitions of test cases without polluting the global namespace with additional types. Here's an example:
var tests = []struct { input string expected string }{ {"input1", "expected1"}, {"input2", "expected2"}, // more test cases } for _, test := range tests { result := someFunction(test.input) if result != test.expected { t.Errorf("For input %s, expected %s but got %s", test.input, test.expected, result) } }
In this setup, each test case is represented by an anonymous struct, making the test definitions straightforward and contained within the test scope.
Considerations When Using Anonymous Structs
While anonymous structs offer flexibility and brevity, they come with certain limitations:
-
Reusability: Since anonymous structs lack a defined type name, you must redefine their structure each time you use them, which can lead to code duplication if the same structure is needed in multiple places.
-
Readability: Overusing anonymous structs, especially nested ones, can make the code harder to read and maintain. In cases where a struct is used in multiple locations or has a complex structure, defining a named struct type is preferable.
For example, when nesting anonymous structs, the syntax can become cumbersome:
data := struct { JsonRpc string `json:"jsonrpc"` Method string `json:"method"` Id string `json:"id"` Params struct { Format string `json:"format"` Cmds []string `json:"cmds"` Version int `json:"version"` } `json:"params"` }{ JsonRpc: "2.0", Method: "someMethod", Id: "someId", Params: struct { Format string Cmds []string Version int }{ Format: "json", Cmds: []string{"some", "list", "of", "commands"}, Version: 1, }, }
In such scenarios, using named structs can enhance clarity and reduce redundancy.
Conclusion
Anonymous structs in Go provide a convenient way to define temporary, one-off data structures without cluttering the global namespace with additional type declarations. They are particularly useful in scenarios like JSON marshalling/unmarshalling and writing table-driven tests. However, it's essential to use them judiciously, considering factors like code readability and reusability. For structures that are used across multiple functions or packages, defining a named struct type is often the better approach.
FAQs
Use anonymous structs for temporary, localized data structures, especially within functions.
Yes, but excessive nesting can reduce code readability, so named structs are better for complex data.
Overuse can lead to redundant code and decreased maintainability.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ