Mastering `fmt.Fprintf` in Go: A Guide to Formatted Output
James Reed
Infrastructure Engineer · Leapcell
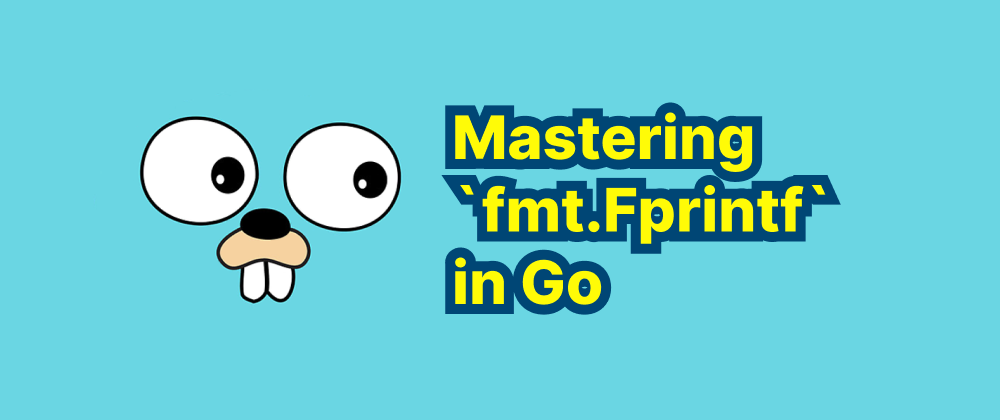
Key Takeaways
fmt.Fprintf
enables formatted output to anyio.Writer
destination.- It is versatile for writing to stdout, stderr, files, and buffers.
- Using
Fprintf
simplifies code and can enhance performance.
In Go, the fmt
package provides various functions for formatted I/O operations, among which Fprintf
stands out for its versatility in directing formatted output to specified destinations. Understanding Fprintf
is essential for developers aiming to have precise control over their program's output.
Overview of fmt.Fprintf
The fmt.Fprintf
function allows developers to format strings and write them to an io.Writer
. This capability is particularly useful when you need to send output to destinations other than the standard output, such as files, network connections, or buffers. The function signature is as follows:
func Fprintf(w io.Writer, format string, a ...any) (n int, err error)
w io.Writer
: The destination where the formatted string will be written. Any type that implements theio.Writer
interface can serve as a destination.format string
: A format specifier string that dictates how subsequent arguments are formatted.a ...any
: Variadic parameters representing the values to be formatted according to the format specifier.
The function returns the number of bytes written and any error encountered during the write operation.
Practical Examples
Writing to Standard Output
To write formatted output to the standard output (os.Stdout
):
package main import ( "fmt" "os" ) func main() { name := "Alice" age := 30 fmt.Fprintf(os.Stdout, "%s is %d years old.\n", name, age) }
Output:
Alice is 30 years old.
Writing to Standard Error
For error messages, it's common to write to the standard error (os.Stderr
):
package main import ( "fmt" "os" ) func main() { _, err := os.Open("nonexistent_file.txt") if err != nil { fmt.Fprintf(os.Stderr, "Error: %v\n", err) } }
This approach ensures that error messages are directed to the standard error stream, which can be separately handled or logged.
Writing to a File
To write formatted output directly to a file:
package main import ( "fmt" "os" ) func main() { file, err := os.Create("output.txt") if err != nil { fmt.Fprintf(os.Stderr, "Failed to create file: %v\n", err) return } defer file.Close() name := "Bob" age := 25 fmt.Fprintf(file, "%s is %d years old.\n", name, age) }
This code creates (or truncates) a file named output.txt
and writes the formatted string into it.
Writing to a Buffer
Using a buffer allows for in-memory writing, which can be useful for constructing strings before outputting them:
package main import ( "bytes" "fmt" ) func main() { var buffer bytes.Buffer name := "Charlie" age := 35 fmt.Fprintf(&buffer, "%s is %d years old.\n", name, age) fmt.Print(buffer.String()) }
Output:
Charlie is 35 years old.
Advantages of Using fmt.Fprintf
- Flexibility:
Fprintf
can write to any destination that implements theio.Writer
interface, providing greater flexibility compared to functions likefmt.Printf
, which writes only to the standard output. - Direct Writing: Using
Fprintf
to write directly to a destination can be more efficient than combiningfmt.Sprintf
with a separate write operation. For example, replacingw.Write([]byte(fmt.Sprintf(...)))
withfmt.Fprintf(w, ...)
simplifies the code and may improve performance.
Conclusion
The fmt.Fprintf
function is a powerful tool in Go's fmt
package, enabling developers to direct formatted output to various destinations seamlessly. Its versatility in handling different io.Writer
implementations makes it indispensable for tasks requiring formatted output beyond the standard console.
FAQs
It formats and writes output to any destination implementing the io.Writer
interface.
While Printf
writes to stdout, Fprintf
allows specifying the output destination.
Yes, as it avoids creating intermediary strings and writes directly to the destination.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ