How to Convert JSON to a Go Struct
Grace Collins
Solutions Engineer · Leapcell
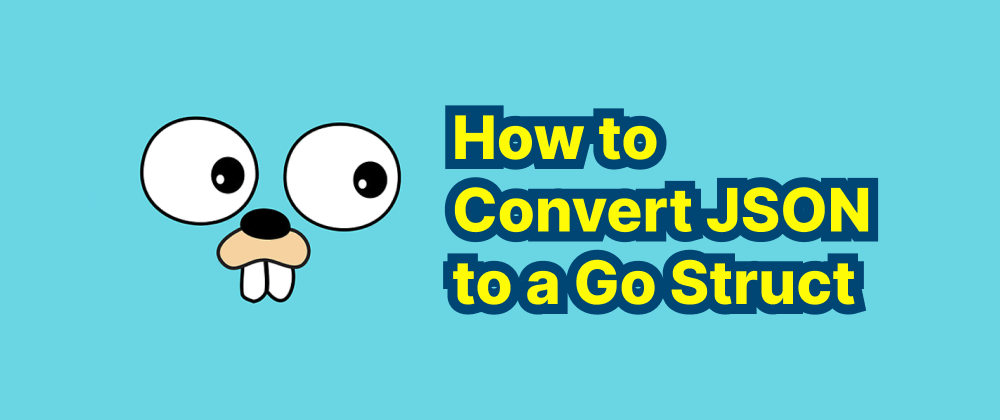
Key Takeaways
- Use struct field tags (
json:"field"
) to map JSON keys to Go struct fields. - Decode JSON into a struct using
json.Unmarshal
and encode it withjson.Marshal
. - Handle nested JSON structures by defining nested Go structs.
JSON (JavaScript Object Notation) is a widely used format for data exchange, and Go (or Golang) provides robust support for working with JSON. Converting JSON data into Go structs allows for type safety and better data handling. In this article, we will explore how to efficiently convert JSON into Go structs.
Defining a Go Struct
Before parsing JSON, you need a corresponding Go struct that matches the JSON structure. Consider the following JSON example:
{ "name": "Alice", "age": 25, "email": "alice@example.com" }
The corresponding Go struct should be defined with appropriate field names and types:
type Person struct { Name string `json:"name"` Age int `json:"age"` Email string `json:"email"` }
The struct field tags (e.g., json:"name"
) map JSON keys to struct fields.
Parsing JSON into a Struct
Go's encoding/json
package provides functions to parse JSON into a struct. Here’s how you can decode JSON into a Go struct:
package main import ( "encoding/json" "fmt" ) func main() { jsonData := `{"name": "Alice", "age": 25, "email": "alice@example.com"}` var person Person err := json.Unmarshal([]byte(jsonData), &person) if err != nil { fmt.Println("Error parsing JSON:", err) return } fmt.Println("Parsed Struct:", person) }
Encoding a Struct into JSON
You can also convert a Go struct into a JSON string using json.Marshal
:
encodedData, err := json.Marshal(person) if err != nil { fmt.Println("Error encoding JSON:", err) return } fmt.Println("JSON Output:", string(encodedData))
Handling Nested JSON Structures
If your JSON contains nested objects, define corresponding nested structs:
{ "name": "Alice", "contact": { "email": "alice@example.com", "phone": "123-456-7890" } }
The corresponding Go struct would be:
type Contact struct { Email string `json:"email"` Phone string `json:"phone"` } type Person struct { Name string `json:"name"` Contact Contact `json:"contact"` }
Using json.RawMessage
for Partial Parsing
If you need to defer parsing of a specific JSON field, use json.RawMessage
:
type Data struct { Info json.RawMessage `json:"info"` }
Conclusion
Converting JSON to Go structs is essential for handling structured data in Go applications. By defining appropriate struct types, using json.Unmarshal
and json.Marshal
, and leveraging features like json.RawMessage
, you can efficiently manage JSON data in Go.
FAQs
The corresponding struct field will have its zero value (e.g., ""
for strings, 0
for integers).
Use the json:"-"
tag to exclude a field from JSON serialization and deserialization.
Yes, use map[string]interface{}
or json.RawMessage
for flexible JSON handling.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ