Machine Learning in Golang: An Overview of Popular Libraries and Examples
James Reed
Infrastructure Engineer · Leapcell
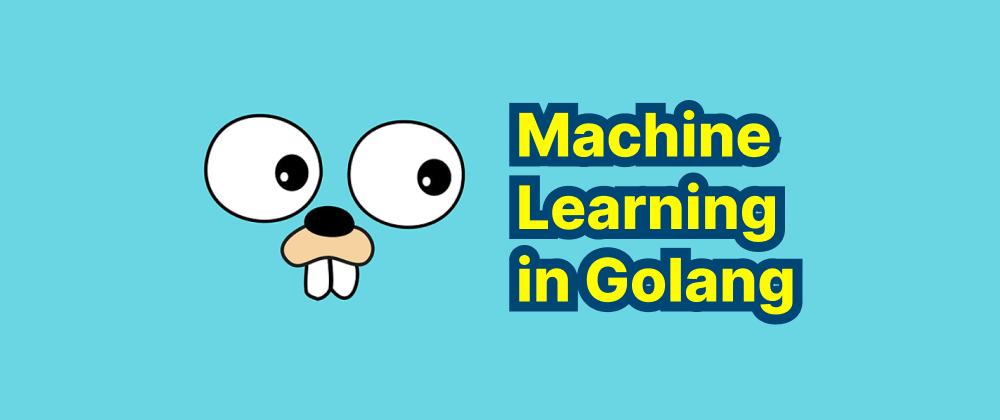
Key Takeaways
- Golang offers efficient machine learning with libraries like GoLearn, Gorgonia, and Gonum.
- GoLearn is ideal for beginners, providing simple APIs for basic ML models.
- Golang's performance and concurrency make it suitable for scalable ML applications.
Golang, known for its efficiency and scalability, is increasingly being used for machine learning (ML) applications. While Python remains the dominant language in the ML space, Go provides advantages such as better performance, static typing, and easy concurrency handling. In this article, we will explore some of the most popular machine learning libraries in Golang and provide a simple example to demonstrate their usage.
Popular Machine Learning Libraries in Golang
Although Go does not have as many mature ML libraries as Python, several libraries support a wide range of ML tasks, from data preprocessing to deep learning. Here are some of the most commonly used ones:
1. Gorgonia
Gorgonia is a powerful library designed for deep learning and numerical computation in Go. It provides a tensor-based computation engine similar to TensorFlow and PyTorch. Gorgonia allows developers to build complex neural networks while taking advantage of Go's concurrency features.
2. Gonum
Gonum is a collection of numerical computing tools, including matrix manipulation, optimization algorithms, and statistical analysis. While it is not specifically designed for ML, it serves as a solid foundation for implementing custom machine learning models.
3. GoLearn
GoLearn is one of the most user-friendly machine learning libraries in Golang. It provides a variety of ML algorithms, including decision trees, k-nearest neighbors (KNN), and support vector machines (SVM). The library is designed with simplicity in mind, making it a great choice for beginners.
4. Fuego
Fuego is a relatively new deep learning library that provides a flexible framework for building neural networks. It includes support for auto-differentiation and GPU acceleration.
Implementing a Simple Machine Learning Model in Go
To demonstrate how machine learning works in Go, we will use the GoLearn library to implement a basic classification model. In this example, we will train a decision tree classifier on the famous Iris dataset.
Installation
First, install GoLearn by running:
go get github.com/sjwhitworth/golearn
Code Example: Classifying the Iris Dataset
package main import ( "fmt" "log" "github.com/sjwhitworth/golearn/base" "github.com/sjwhitworth/golearn/evaluation" "github.com/sjwhitworth/golearn/trees" ) func main() { // Load the Iris dataset data, err := base.ParseCSVToInstances("iris.csv", true) if err != nil { log.Fatal(err) } // Split dataset into training (80%) and test (20%) sets trainData, testData := base.InstancesTrainTestSplit(data, 0.80) // Create a Decision Tree classifier tree := trees.NewID3DecisionTree(0.6) // Train the classifier err = tree.Fit(trainData) if err != nil { log.Fatal(err) } // Make predictions on the test set predictions, err := tree.Predict(testData) if err != nil { log.Fatal(err) } // Evaluate the model's performance confusionMat, err := evaluation.GetConfusionMatrix(testData, predictions) if err != nil { log.Fatal(err) } // Print accuracy fmt.Println(evaluation.GetSummary(confusionMat)) }
Explanation
- Loading the Dataset: The
base.ParseCSVToInstances()
function reads the dataset from a CSV file. - Splitting the Data: The dataset is split into training and testing sets using
base.InstancesTrainTestSplit()
. - Training the Model: We create an ID3 decision tree classifier and train it with the training data.
- Making Predictions: The model predicts outcomes for the test dataset.
- Evaluating Performance: We compute a confusion matrix to measure accuracy.
Conclusion
While Go is not traditionally used for machine learning, libraries like Gorgonia, GoLearn, and Gonum provide powerful tools for ML development. Go is especially useful for ML applications that require performance optimization, concurrency, or integration into large-scale backend systems.
If you are exploring machine learning in Go, starting with GoLearn for simple models and moving to Gorgonia for deep learning tasks is a great approach. As the Go ML ecosystem grows, we can expect more robust tools and frameworks to emerge.
FAQs
Gorgonia is best suited for deep learning tasks in Go.
Yes, but it's more efficient for basic and smaller-scale machine learning models.
Golang provides high performance, efficient concurrency, and easy integration into backend systems.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ