Understanding Environment Variables in Golang
Grace Collins
Solutions Engineer · Leapcell
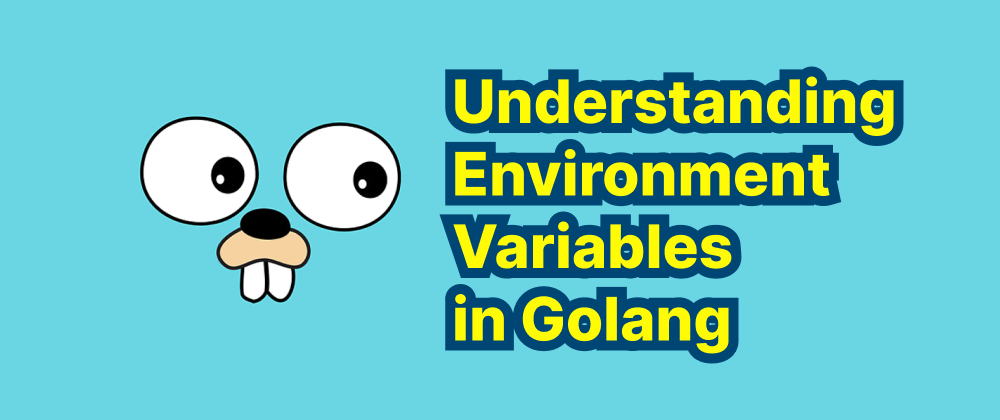
Key Takeaways
- Golang provides built-in support for reading, setting, and listing environment variables via the
os
package. - Using
.env
files with libraries likegodotenv
simplifies environment variable management in development. - Best practices include avoiding hardcoding secrets, using defaults, and securing sensitive data.
Environment variables are a crucial part of any application, allowing developers to configure settings dynamically without modifying the source code. In Golang, handling environment variables efficiently is essential for building scalable and maintainable applications. This article explores how to work with environment variables in Golang, covering basic operations, best practices, and common use cases.
What Are Environment Variables?
Environment variables are key-value pairs stored outside an application’s codebase, typically set at the operating system level. They provide a way to manage configuration settings such as database credentials, API keys, and runtime configurations without hardcoding them.
For example, in a Unix-based system, you can set an environment variable using:
export APP_ENV=production
In Windows, you can set an environment variable using:
set APP_ENV=production
Golang provides built-in support for reading and managing environment variables through the os
package.
Reading Environment Variables in Golang
To retrieve an environment variable in Golang, you can use the os.Getenv
function:
package main import ( "fmt" "os" ) func main() { appEnv := os.Getenv("APP_ENV") if appEnv == "" { appEnv = "development" // Default value if the variable is not set } fmt.Println("Application Environment:", appEnv) }
If the variable is not set, os.Getenv
returns an empty string, so it’s common to define a default value.
Setting Environment Variables in Golang
You can also set environment variables within your application using os.Setenv
:
os.Setenv("APP_MODE", "debug") fmt.Println("APP_MODE:", os.Getenv("APP_MODE"))
However, this change only affects the running process and does not persist after the program exits.
Listing All Environment Variables
To get all environment variables, you can use os.Environ
, which returns a slice of strings in "KEY=VALUE"
format:
for _, env := range os.Environ() { fmt.Println(env) }
This is useful for debugging or logging configuration settings.
Using os.LookupEnv
for Safer Lookups
Instead of checking for an empty string, os.LookupEnv
allows you to distinguish between an unset variable and an empty value:
val, exists := os.LookupEnv("APP_ENV") if !exists { fmt.Println("APP_ENV is not set") } else { fmt.Println("APP_ENV:", val) }
Loading Environment Variables from a .env
File
Many applications store environment variables in a .env
file, especially during development. The popular github.com/joho/godotenv
package helps load these variables into the environment.
Installation
go get github.com/joho/godotenv
Usage
Create a .env
file:
APP_ENV=production
DB_HOST=localhost
Then load it in your Go application:
package main import ( "fmt" "log" "os" "github.com/joho/godotenv" ) func main() { err := godotenv.Load() if err != nil { log.Fatal("Error loading .env file") } fmt.Println("APP_ENV:", os.Getenv("APP_ENV")) fmt.Println("DB_HOST:", os.Getenv("DB_HOST")) }
Best Practices for Using Environment Variables
-
Do Not Hardcode Sensitive Information
Always store API keys, credentials, and other secrets in environment variables or a secure vault. -
Use Default Values
Ensure your application does not crash if an environment variable is missing by providing sensible defaults. -
Separate Configuration for Different Environments
Maintain different.env
files for development, testing, and production environments. -
Avoid Overuse of Environment Variables
Keep them minimal; use configuration files or a dedicated service for complex configurations. -
Securely Manage Secrets
Use secret management tools like AWS Secrets Manager, HashiCorp Vault, or Kubernetes secrets for better security.
Conclusion
Golang provides robust support for environment variables, making it easy to configure applications dynamically. By following best practices, you can ensure your application remains secure, flexible, and maintainable. Whether using built-in functions or external libraries like godotenv
, properly managing environment variables is essential for developing reliable Golang applications.
FAQs
Use os.LookupEnv
, which returns both the value and a boolean indicating existence.
It allows loading environment variables from a .env
file, simplifying development configurations.
Use os.Environ()
, which returns all variables as a slice of "KEY=VALUE"
strings.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ