How to Write JSON to a File in Python
Wenhao Wang
Dev Intern · Leapcell
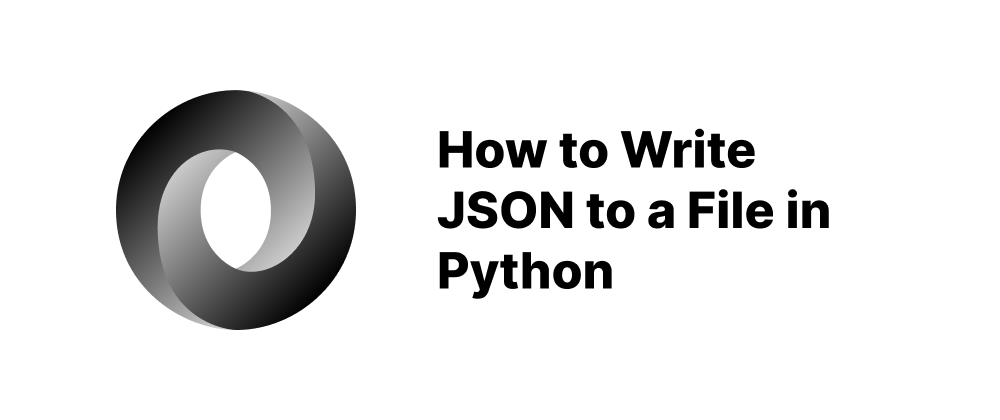
Key Takeaways
- Use Python's built-in
json
module for JSON file operations. json.dump()
writes Python dictionaries to a file as JSON.- Adding
indent
improves readability of the output.
JSON (JavaScript Object Notation) is a lightweight data format widely used for data exchange between systems. In Python, working with JSON is simple thanks to the built-in json
module. This article will guide you through the steps to write JSON data to a file using Python.
Why Use JSON in Python?
JSON is both human-readable and easy to parse, making it ideal for storing configuration, transmitting data between a server and a client, or even saving application data locally. Python's json
module provides convenient methods to handle JSON serialization and deserialization.
Step 1: Import the JSON Module
To work with JSON in Python, you first need to import the json
module:
import json
Step 2: Create a Python Dictionary
Before writing to a JSON file, you typically have your data in the form of a Python dictionary or list:
data = { "name": "Alice", "age": 30, "email": "alice@example.com", "skills": ["Python", "Data Analysis", "Machine Learning"] }
Step 3: Write JSON to a File
To write JSON data to a file, use the json.dump()
method:
with open("data.json", "w") as file: json.dump(data, file)
This will create a file named data.json
and write the dictionary into it in JSON format.
Optional: Pretty Printing the JSON Output
For better readability, you can format the JSON with indentation:
with open("data_pretty.json", "w") as file: json.dump(data, file, indent=4)
This adds whitespace and line breaks, making the file easier to read.
Step 4: Confirm the File Contents (Optional)
You can verify that the JSON data was written correctly by opening the file manually or reading it back in Python:
with open("data.json", "r") as file: loaded_data = json.load(file) print(loaded_data)
Conclusion
Writing JSON data to a file in Python is straightforward using the json.dump()
method. Whether you're saving user settings, application logs, or exporting data, JSON is a flexible and widely supported format. Remember to format your output with indent
if you want a human-readable result.
FAQs
You can write Python dictionaries, lists, strings, numbers, and booleans.
Use the indent
parameter in json.dump()
to format the output.
No, json.dump()
writes to a file, while json.dumps()
returns a JSON string.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ