Understanding JSON Arrays of Arrays
Grace Collins
Solutions Engineer · Leapcell
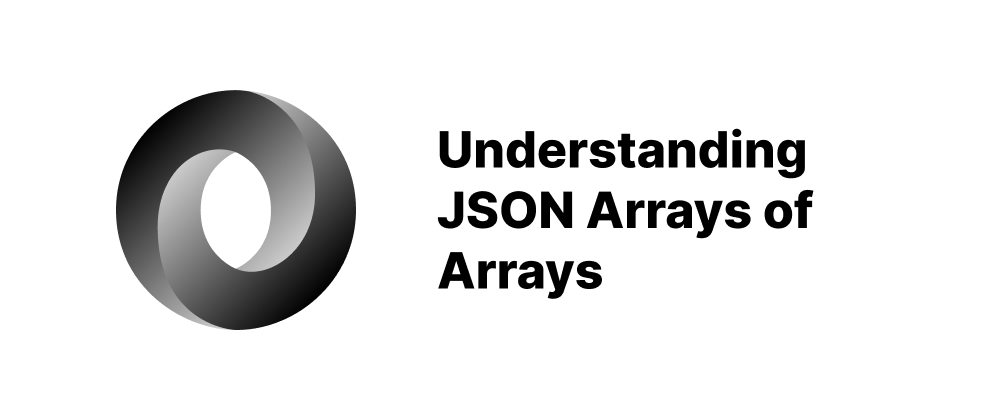
Key Takeaways
- JSON arrays of arrays are useful for representing multi-dimensional data.
- Each element in the outer array can itself be an array, enabling structured organization.
- Proper parsing and validation are essential when handling nested JSON arrays.
JSON (JavaScript Object Notation) is a lightweight data-interchange format widely used for transmitting structured data over the web. One of its powerful features is the ability to nest arrays within arrays, enabling the representation of complex, multi-dimensional data structures.
What Is a JSON Array of Arrays?
A JSON array of arrays, also known as a multi-dimensional array, is a structure where each element of the main array is itself an array. This nesting allows for the organization of data in a tabular or matrix-like format.
Example:
{ "matrix": [ ["a", "b", "c"], ["d", "e", "f"], ["g", "h", "i"] ] }
In this example, the matrix
key holds an array containing three arrays, each representing a row in the matrix.
Use Cases
JSON arrays of arrays are particularly useful in scenarios such as:
- Tabular Data Representation: Storing spreadsheet-like data where each sub-array represents a row.
- Grid-Based Systems: Representing game boards or seating arrangements.
- Mathematical Matrices: Handling numerical data for computations.
Accessing Elements
Accessing elements within nested arrays involves specifying the indices for each level of nesting.
Example:
const data = { matrix: [ ["a", "b", "c"], ["d", "e", "f"], ["g", "h", "i"] ] }; console.log(data.matrix[0][1]); // Outputs: "b"
Here, data.matrix[0][1]
accesses the first row (["a", "b", "c"]
) and then the second element within that row ("b"
).
Parsing JSON Arrays of Arrays
When working with JSON data in programming languages, parsing nested arrays requires iterating through each level.
Example in JavaScript:
const jsonData = '{"matrix": [["a", "b"], ["c", "d"]]}'; const obj = JSON.parse(jsonData); obj.matrix.forEach((row, rowIndex) => { row.forEach((value, colIndex) => { console.log(`Value at [${rowIndex}][${colIndex}]: ${value}`); }); });
This script parses the JSON string and iterates through each row and column, outputting the values with their respective indices.
Best Practices
- Consistency: Ensure that all sub-arrays have the same length to maintain a uniform structure.
- Validation: When parsing JSON data, validate the structure to handle unexpected formats or missing elements gracefully.
- Performance: Be mindful of performance implications when dealing with large nested arrays, especially in resource-constrained environments.
Conclusion
JSON arrays of arrays provide a flexible way to represent complex data structures. Understanding how to create, access, and manipulate these nested arrays is essential for developers working with JSON in various applications.
FAQs
It's a nested structure where each item in the main array is itself an array.
They’re used in grids, matrices, and tabular data representations.
Use multiple indices like array[outer][inner]
.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ