Resource Pooling in Go Explained: Best Practices, Anti-Patterns, and Monitoring
Takashi Yamamoto
Infrastructure Engineer · Leapcell
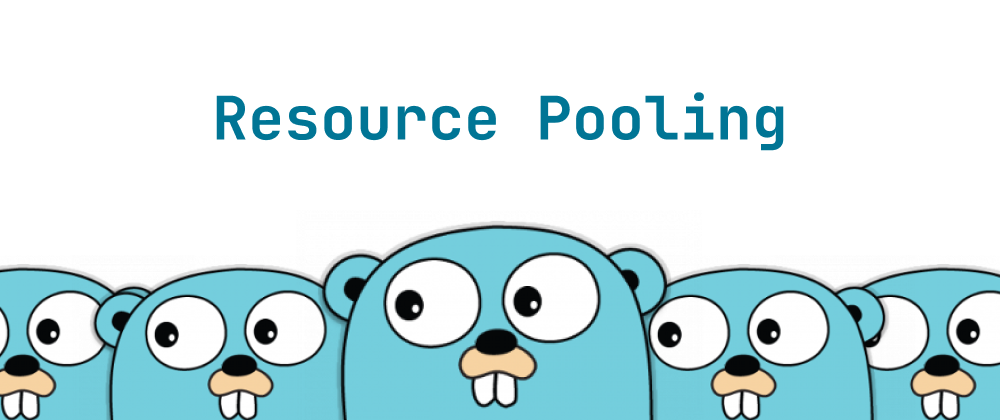
In daily development, we often encounter, or at least hear about, issues like services crashing due to resource exhaustion, sudden spikes in memory caused by repeated object creation, and performance problems arising from frequent database connection creation. The common thread in these issues is the repeated creation of resources and the lack of effective resource utilization. Pooling technology offers an excellent way to address these problems.
Basic Concepts of Pooling Design
Pooling is a design pattern that pre-creates and manages resource instances to avoid the overhead of frequent creation and destruction. In this article, we will learn from and borrow the design ideas of the connection pool implementation in Go’s database/sql package, which is a model of pooling technology.
Core Values of Pooling
- Performance improvement: Reuse existing resources, reducing creation/destruction overhead.
- Resource control: Prevent resource exhaustion and system crashes.
- Enhanced stability: Smooth out traffic spikes, avoid instant pressure surges.
- Unified management: Centralized handling of resource lifecycle and health status.
Pooling Definition in database/sql
Take the following simplified struct as an example, which defines the core parameters of a database connection pool, such as the maximum number of connections, idle connections, and connection lifetime.
// Key pooling fields in the DB struct type DB struct { freeConn []*driverConn // Idle connection pool connRequests connRequestSet // Waiting queue numOpen int // Currently open connections maxOpen int // Maximum open connections maxIdle int // Maximum idle connections maxLifetime time.Duration // Max connection lifetime ··· }
Best Practices for Connection Pool Design
Resource Lifecycle Management
Key points:
- Clearly define strategies for resource creation, validation, reuse, and destruction.
- Implement resource health checks and automatic reclamation.
// Lifecycle management fields in driverConn type driverConn struct { db *DB createdAt time.Time // Creation timestamp returnedAt time.Time // Last return time closed bool // Closed state flag needReset bool // Whether a reset is needed before use ··· }
Configuration recommendations:
// Recommended settings db.SetMaxOpenConns(100) // Determined by load testing db.SetMaxIdleConns(20) // About 20-30% of MaxOpen db.SetConnMaxLifetime(30*time.Minute) // Avoid using the same connection for too long db.SetConnMaxIdleTime(5*time.Minute) // Timely reclaim of idle resources
Concurrency-Safe Design
Key points:
- Use atomic operations for counters.
- Fine-grained lock design.
- Non-blocking waiting mechanisms.
By using atomic operations, we reduce the performance cost of locking. Core variable assignments and asynchronous database connection operations are protected by write locks.
// Concurrency control in database/sql type DB struct { // Atomic counters waitDuration atomic.Int64 numClosed atomic.Uint64 mu sync.Mutex // Protects core fields openerCh chan struct{} // Channel for async connection creation ··· }
Resource Allocation Strategies
Key points:
- Combine lazy loading and pre-warming.
- Design reasonable waiting queues.
- Provide timeout control mechanisms.
The connection pool (sql.DB) only creates and allocates database connections when a database operation is actually performed for the first time. When you call db.Query() or db.Exec(), sql.DB tries to fetch a connection from the pool. If there is no idle connection, it will try to create a new connection according to the maximum number of connections configured.
database/sql manages connection allocation via a connection pool. The pool size is affected by SetMaxOpenConns and SetMaxIdleConns. If there are no idle connections, the pool uses a queue mechanism to wait for an available connection.
database/sql supports query timeouts using context, which is especially useful when database operations become slow due to network latency or database load. With QueryContext, ExecContext, and similar methods, you can specify a context for each query operation, which will automatically abort the query if it times out or is canceled.
// Use user-provided context to implement context control func (db *DB) QueryContext(ctx context.Context, query string, args ...any) (*Rows, error) { var rows *Rows var err error err = db.retry(func(strategy connReuseStrategy) error { rows, err = db.query(ctx, query, args, strategy) return err }) return rows, err }
Comparison of waiting strategies:
Fail Fast
- Pros: Fast response
- Cons: Poor user experience
- Suitable scenarios: High-concurrency writes
Blocking Wait
- Pros: Guaranteed success
- Cons: May block for a long time
- Suitable scenarios: Critical business processes
Timeout Wait
- Pros: Balanced experience
- Cons: More complex implementation
- Suitable scenarios: Most scenarios
Exception Handling & Robustness
Monitoring Metric Design:
type DBStats struct { MaxOpenConnections int // Pool capacity OpenConnections int // Current connections InUse int // Connections in use Idle int // Idle connections WaitCount int64 // Number of waits WaitDuration int64 // Total wait time MaxIdleClosed int64 // Closed due to idleness MaxLifetimeClosed int64 // Closed due to expiration }
Example of Using Monitoring Metrics
// View the status of the connection pool stats := sqlDB.Stats() fmt.Printf("Open connections: %d\n", stats.OpenConnections) fmt.Printf("In-use connections: %d\n", stats.InUse) fmt.Printf("Idle connections: %d\n", stats.Idle)
Anti-Patterns and Common Pitfalls
Practices to Avoid
Connection Leak:
// Incorrect Example: Forgetting to close the connection rows, err := db.Query("SELECT...") // Missing rows.Close()
Improper Pool Size Configuration:
// Incorrect Configuration: No limit on the maximum number of connections db.SetMaxOpenConns(0) // Unlimited
Ignoring Connection State:
// Dangerous Operation: Not handling errors conn, _ := db.Conn(context.Background()) conn.Close() // Returned to the pool, but the state may be polluted
Proper Resource Handling Pattern
Correct example for transaction handling:
// transferMoney performs a transfer operation func transferMoney(fromID, toID, amount int) error { // Start transaction tx, err := db.Begin() if err != nil { return fmt.Errorf("failed to begin transaction: %w", err) } // Automatically roll back on function exit if there is an error defer func() { if err != nil { // Roll back the transaction if rbErr := tx.Rollback(); rbErr != nil { log.Printf("Error rolling back transaction: %v", rbErr) } } }() // Perform debit operation _, err = tx.Exec("UPDATE accounts SET balance = balance - ? WHERE id = ?", amount, fromID) if err != nil { return fmt.Errorf("failed to deduct amount from account %d: %w", fromID, err) } // Perform credit operation _, err = tx.Exec("UPDATE accounts SET balance = balance + ? WHERE id = ?", amount, toID) if err != nil { return fmt.Errorf("failed to credit amount to account %d: %w", toID, err) } // Commit the transaction if err := tx.Commit(); err != nil { return fmt.Errorf("failed to commit transaction: %w", err) } // No errors, transaction committed successfully return nil }
Performance Optimization Recommendations
Connection Pre-Warming:
// Pre-warm the connection pool on service startup func warmUpPool(db *sql.DB, count int) { var wg sync.WaitGroup for i := 0; i < count; i++ { wg.Add(1) go func() { defer wg.Done() db.Ping() }() } wg.Wait() }
Batch Operation Optimization:
// Use batch inserts to reduce the number of connection acquisitions func bulkInsert(db *sql.DB, items []Item) error { tx, err := db.Begin() if err != nil { return err } stmt, err := tx.Prepare("INSERT...") if err != nil { tx.Rollback() return err } for _, item := range items { if _, err = stmt.Exec(...); err != nil { tx.Rollback() return err } } return tx.Commit() }
Connection Pool Monitoring Dashboard:
Metrics: Connection wait time
- Healthy threshold: < 100ms
- Alert strategy: Trigger alert if threshold is exceeded 3 times in a row
Metrics: Connection utilization
- Healthy threshold: 30%-70%
- Alert strategy: If out of range for 10 minutes
Metrics: Error rate
- Healthy threshold: < 0.1%
- Alert strategy: Increases by 10x within 5 minutes
Summary
The connection pool implementation in database/sql demonstrates excellent pooling design principles:
- Transparency: Hides complex details from the user.
- Elasticity: Dynamically adjusts resources according to the load.
- Robustness: Comprehensive error handling and automatic recovery.
- Controllability: Provides rich configuration and monitoring metrics.
Applying these principles to other pooling scenarios (such as thread pools, memory pools, object pools) can help you build equally efficient and reliable resource management systems. Remember, a good pooling design should be like database/sql: keep simple things simple, and make complex things possible.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ