Understanding SQL Variables: Declaration, Usage, and Best Practices
Grace Collins
Solutions Engineer · Leapcell
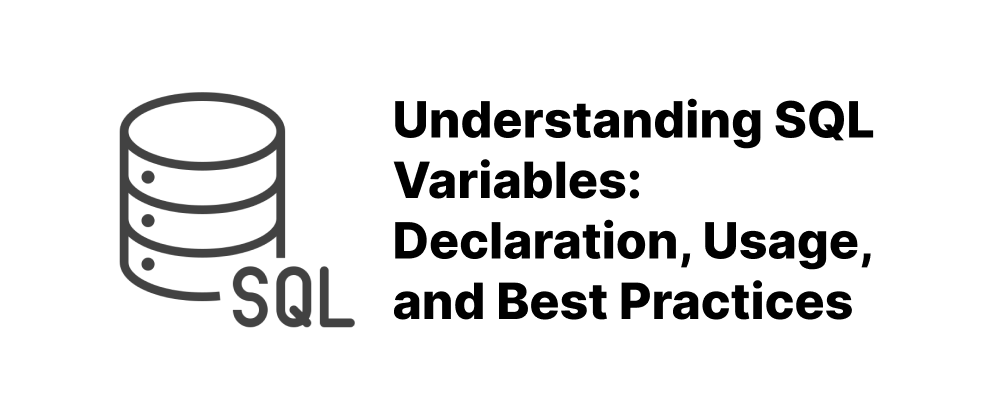
Key Takeaways
- SQL variables store and manipulate data temporarily within queries and procedures.
- The declaration and usage of variables differ across SQL database systems.
- Managing scope and initialization is crucial for reliable SQL code.
SQL variables are essential tools in database programming, allowing developers to store temporary data, control the flow of execution, and create dynamic, reusable queries. This article explores the concept of SQL variables, their declaration, assignment, scope, and usage across different database management systems (DBMS).
What Are SQL Variables?
An SQL variable is a named storage location that holds a single data value of a specific type during the execution of SQL statements. Variables are commonly used in stored procedures, functions, scripts, and batches to:
- Store intermediate results.
- Control program flow using conditional logic.
- Enhance code readability and maintainability.
- Facilitate dynamic SQL query construction.
Declaring Variables
The syntax for declaring variables varies among different DBMS. Below are examples for some popular systems:
SQL Server (Transact-SQL)
In SQL Server, variables are declared using the DECLARE
statement.
DECLARE @MyVariable INT; SET @MyVariable = 10;:contentReference[oaicite:39]{index=39}
You can also declare and initialize a variable in a single statement:
DECLARE @MyVariable INT = 10;:contentReference[oaicite:45]{index=45}
Multiple variables can be declared simultaneously:
DECLARE @Var1 INT = 5, @Var2 VARCHAR(50) = 'Hello';:contentReference[oaicite:51]{index=51}
MySQL
In MySQL, variables can be declared within stored procedures using the DECLARE
statement:
DECLARE myVar INT; SET myVar = 10;:contentReference[oaicite:59]{index=59}
For session-level variables, you can use the SET
statement:
SET @myVar = 10;:contentReference[oaicite:65]{index=65}
PostgreSQL
In PostgreSQL, variables are typically used within procedural code blocks:
DO $$ DECLARE myVar INT := 10; BEGIN -- Use myVar here END $$;:contentReference[oaicite:77]{index=77}
Oracle (PL/SQL)
Oracle uses PL/SQL blocks for variable declaration:
DECLARE myVar NUMBER := 10; BEGIN -- Use myVar here END;:contentReference[oaicite:87]{index=87}
Assigning Values to Variables
After declaring a variable, you can assign values using the SET
or SELECT
statements.
Using SET
The SET
statement assigns a single value to a variable:
SET @MyVariable = 20;:contentReference[oaicite:97]{index=97}
Using SELECT
The SELECT
statement can assign values from a query result:
SELECT @MyVariable = column_name FROM table_name WHERE condition;:contentReference[oaicite:103]{index=103}
Note: If the SELECT
statement returns multiple rows, the variable will be assigned the value from the last row.
Variable Scope
The scope of a variable determines where it can be accessed within the SQL code.
-
SQL Server: Variables have a local scope within the batch, stored procedure, or function where they are declared. They are not accessible outside of this scope.
-
MySQL: Variables declared within stored procedures are local to those procedures. Session variables (prefixed with
@
) are accessible throughout the session. -
PostgreSQL and Oracle: Variables declared within a block are local to that block and its nested sub-blocks.
Best Practices
-
Use Descriptive Names: Choose meaningful variable names to enhance code readability.
-
Initialize Variables: Always initialize variables to avoid unexpected
NULL
values. -
Manage Scope Carefully: Be aware of variable scope to prevent conflicts and unintended behavior.
-
Use
SET
for Single Assignments: PreferSET
when assigning a single value to a variable. -
Use
SELECT
for Assignments from Queries: UseSELECT
when assigning values from query results, ensuring the query returns only one row.
Conclusion
SQL variables are powerful tools that enhance the flexibility and efficiency of SQL programming. By understanding how to declare, assign, and manage variables across different DBMS, developers can write more dynamic and maintainable SQL code.
FAQs
To temporarily store values for use in SQL queries, scripts, and procedures.
Use the DECLARE
statement, e.g., DECLARE @MyVar INT;
.
No, variable scope rules differ by database system.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ