How to Replace a String in JavaScript
James Reed
Infrastructure Engineer · Leapcell
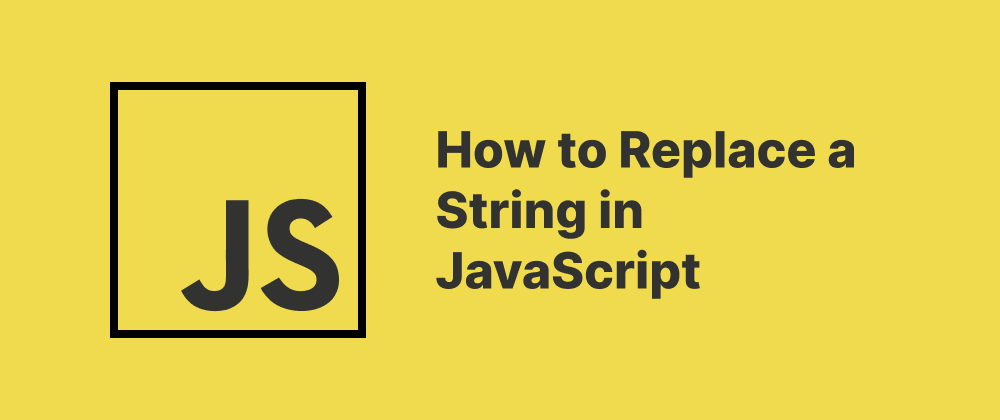
Key Takeaways
- Use
replace()
to modify parts of a string in JavaScript. - Regular expressions allow global and case-insensitive replacements.
- A function can be used as the replacement to generate dynamic values.
String manipulation is a common task in JavaScript, and one of the most frequently used operations is replacing parts of a string. Whether you're modifying user input, processing data, or formatting text, JavaScript provides several methods to perform string replacements efficiently and flexibly.
Using String.prototype.replace()
The primary method for replacing part of a string in JavaScript is the replace()
method.
Syntax
str.replace(searchValue, replaceValue);
searchValue
can be a string or a regular expression.replaceValue
can be a string or a function to generate the replacement.
Example 1: Replacing a Simple Substring
const message = "Hello, world!"; const updated = message.replace("world", "JavaScript"); console.log(updated); // "Hello, JavaScript!"
This replaces the first occurrence of "world"
with "JavaScript"
.
Example 2: Replacing Using a Regular Expression
const sentence = "The rain in Spain stays mainly in the plain."; const result = sentence.replace(/ain/g, "☀️"); console.log(result); // "The r☀️ in Sp☀️ stays m☀️ly in the pl☀️."
Adding the g
(global) flag ensures all matches are replaced, not just the first one.
Case-Insensitive Replacement
You can use the i
flag in a regular expression to make the match case-insensitive:
const text = "Hello HELLO hello"; const result = text.replace(/hello/gi, "hi"); console.log(result); // "hi hi hi"
Using a Replacement Function
If you want to compute the replacement dynamically, you can pass a function as the second argument:
const prices = "Product A: $5, Product B: $10"; const updated = prices.replace(/\$(\d+)/g, (match, p1) => { const doubled = parseInt(p1) * 2; return `$${doubled}`; }); console.log(updated); // "Product A: $10, Product B: $20"
Common Pitfall: Replace Only Replaces the First Match
By default, replace()
only replaces the first occurrence unless you use a regular expression with the g
flag:
const str = "apple apple apple"; console.log(str.replace("apple", "orange")); // "orange apple apple"
To replace all, use:
console.log(str.replace(/apple/g, "orange")); // "orange orange orange"
Summary
- Use
replace()
to modify strings in JavaScript. - Use regular expressions for global or complex replacements.
- Use the
g
flag to replace all occurrences. - You can pass a function to
replace()
to compute the replacement value dynamically.
Understanding these techniques will help you manipulate strings effectively in any JavaScript application.
FAQs
By default, replace()
targets only the first match unless a regular expression with the g
flag is used.
Yes, use a regular expression with the i
flag for case-insensitive matching.
Pass a function as the second argument to replace()
; it will be called for each match.
We are Leapcell, your top choice for hosting Node.js projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ