JavaScript String Formatting: Alternatives to `printf()`
James Reed
Infrastructure Engineer · Leapcell
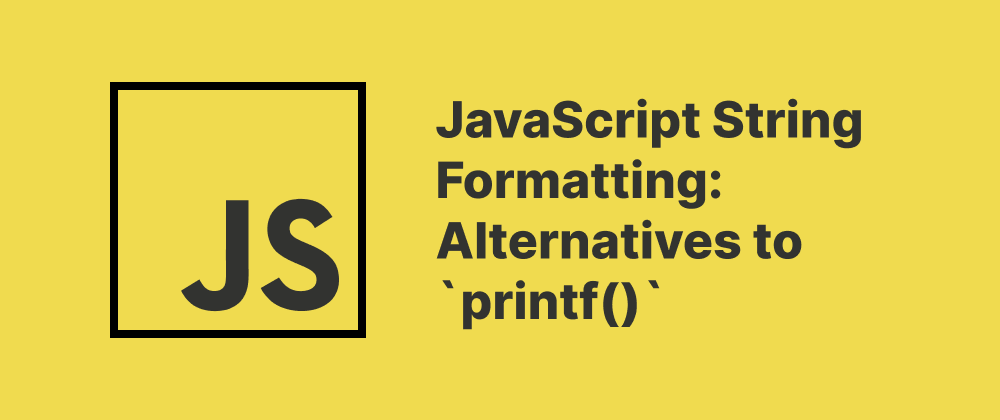
Key Takeaways
- JavaScript uses template literals (
${}
) for intuitive string interpolation. - You can create custom formatting functions to mimic
printf()
behavior. toLocaleString()
formats numbers according to locale settings.
JavaScript doesn't have a built-in printf()
function like C or String.Format()
like C#. However, it offers several effective methods to achieve similar string formatting. This guide explores the most practical approaches:
1. Template Literals (ES6+)
Template literals, introduced in ES6, allow embedding variables and expressions directly into strings using backticks (`
) and ${}
syntax.
Example:
const name = "Alice"; const age = 30; console.log(`My name is ${name} and I am ${age} years old.`); // Output: My name is Alice and I am 30 years old.
This method is concise and readable, making it ideal for most use cases.
2. Custom format()
Function
For scenarios requiring numbered placeholders (e.g., {0}
, {1}
), you can define a custom format()
function.
Example:
String.prototype.format = function () { const args = arguments; return this.replace(/{(\d+)}/g, function (match, number) { return typeof args[number] !== 'undefined' ? args[number] : match; }); }; const template = "Hello {0}, welcome to {1}."; console.log(template.format("Bob", "Los Angeles")); // Output: Hello Bob, welcome to Los Angeles.
This approach mimics the behavior of String.Format()
in languages like C#.
3. Formatting Numbers with toLocaleString()
To format numbers with locale-specific separators (e.g., commas), use the toLocaleString()
method.
Example:
const number = 1234567.89; console.log(number.toLocaleString()); // Output (en-US): 1,234,567.89
This method is useful for displaying numbers in a user-friendly format.
4. Custom printf()
Function
You can create a custom printf()
function to handle placeholder replacements.
Example:
function printf(format, ...args) { return format.replace(/{(\d+)}/g, (match, number) => { return typeof args[number] !== 'undefined' ? args[number] : match; }); } console.log(printf("The sum of {0} and {1} is {2}.", 5, 10, 15)); // Output: The sum of 5 and 10 is 15.
This function provides flexibility for dynamic string construction.
Conclusion
While JavaScript lacks a native printf()
function, the combination of template literals, custom formatting functions, and built-in methods like toLocaleString()
offers powerful alternatives for string formatting. Choose the method that best fits your specific needs.
FAQs
No, but similar functionality can be achieved with template literals or custom functions.
Template literals (${}
within backticks) are the cleanest and most modern option.
Yes, use .toLocaleString()
for locale-aware number formatting.
We are Leapcell, your top choice for hosting Node.js projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ