How to Sleep in JavaScript Using Async/Await
Daniel Hayes
Full-Stack Engineer · Leapcell
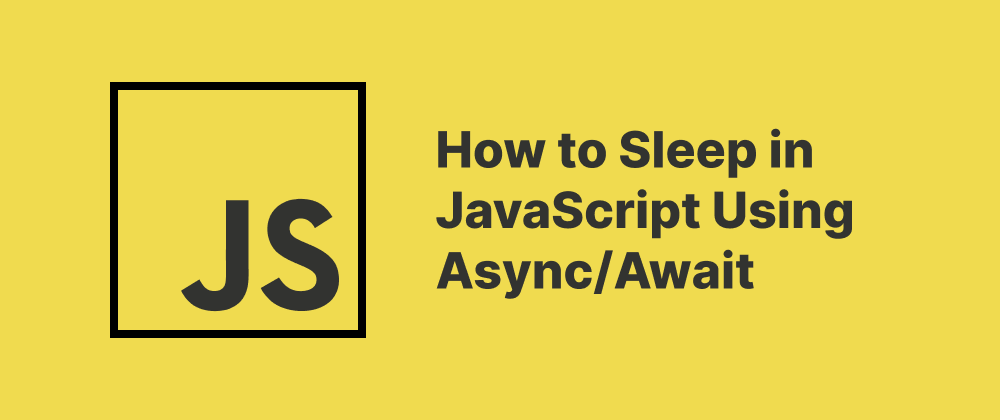
Key Takeaways
- JavaScript doesn’t have a built-in
sleep()
but it can be simulated withsetTimeout
and Promises. - Using
async/await
allows you to pause execution in asynchronous functions. - This non-blocking sleep method keeps the main thread responsive.
In many programming languages, a built-in sleep()
function allows developers to pause code execution for a specified duration. However, JavaScript does not have a native sleep()
function. Nevertheless, you can achieve similar functionality using asynchronous programming techniques. This article explores various methods to implement a sleep-like behavior in JavaScript.
Understanding JavaScript's Asynchronous Nature
JavaScript is inherently asynchronous and single-threaded. Functions like setTimeout()
and setInterval()
allow you to schedule code execution after a delay, but they do not pause the execution of the code that follows. For example:
console.log('Start'); setTimeout(() => { console.log('Delayed'); }, 1000); console.log('End');
Output:
Start
End
Delayed
As seen above, the setTimeout()
function schedules the console.log('Delayed')
statement to run after 1 second, but the code does not wait for this delay and proceeds to execute console.log('End')
immediately.
Creating a Custom Sleep Function Using Promises
To simulate a sleep function, you can create a function that returns a Promise
which resolves after a specified delay. This approach allows you to use async/await
syntax to pause execution within asynchronous functions.
function sleep(ms) { return new Promise(resolve => setTimeout(resolve, ms)); }
Usage Example:
async function demoSleep() { console.log('Sleeping for 2 seconds...'); await sleep(2000); console.log('Awake now!'); } demoSleep();
Output:
Sleeping for 2 seconds...
Awake now!
In this example, the demoSleep
function is declared as async
, allowing the use of the await
keyword to pause execution until the sleep
function's promise resolves after 2 seconds.
One-Liner Sleep Function
For concise code, you can define the sleep function as a one-liner using arrow function syntax:
const sleep = ms => new Promise(resolve => setTimeout(resolve, ms));
This version functions identically to the previous example but offers a more compact syntax.
Using Sleep in Loops
The custom sleep function can be particularly useful within loops where you want to introduce delays between iterations.
async function countWithDelay() { for (let i = 1; i <= 5; i++) { console.log(`Count: ${i}`); await sleep(1000); // Wait for 1 second } console.log('Counting completed.'); } countWithDelay();
Output:
Count: 1
Count: 2
Count: 3
Count: 4
Count: 5
Counting completed.
In this example, the loop waits for 1 second between each iteration, effectively pausing execution as desired.
Important Considerations
-
Async Functions Only: The
await
keyword can only be used inside functions declared with theasync
keyword. Attempting to useawait
outside of anasync
function will result in a syntax error. -
Non-Blocking Behavior: The custom sleep function does not block the main thread. It pauses execution within the
async
function but allows other operations to continue running concurrently. -
Avoid Blocking Loops: Do not use busy-wait loops (e.g., while loops that check the current time) to implement delays, as they block the main thread and can make your application unresponsive.
Conclusion
While JavaScript lacks a native sleep()
function, you can effectively simulate this behavior using Promises and async/await
syntax. This approach allows you to pause execution within asynchronous functions without blocking the main thread, enabling more readable and maintainable code when dealing with delays.
FAQs
No, await sleep()
must be used inside an async
function.
No, it’s non-blocking and allows other tasks to run concurrently.
Yes: const sleep = ms => new Promise(r => setTimeout(r, ms));
We are Leapcell, your top choice for hosting Node.js projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ