Understanding Absolute Value in JavaScript
James Reed
Infrastructure Engineer · Leapcell
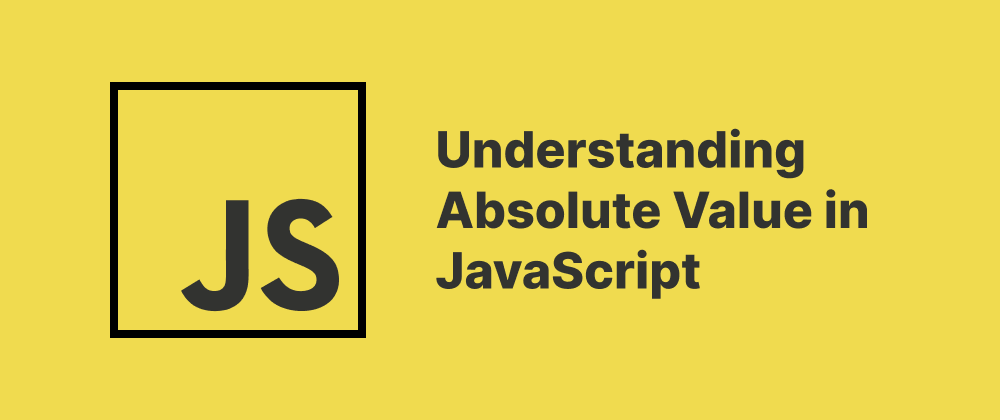
Key Takeaways
- Use
Math.abs()
to get the absolute (non-negative) value of a number in JavaScript. Math.abs()
coerces non-numeric values to numbers or returnsNaN
if conversion fails.- Common use cases include distance calculations and sanitizing numeric input.
Introduction
When working with numbers in JavaScript, you may often need to convert negative values into positive ones or calculate the distance between numbers regardless of direction. This is where absolute value comes in. The absolute value of a number is its non-negative value, regardless of its sign. For example, the absolute value of both -10
and 10
is 10
.
JavaScript provides a built-in way to get the absolute value of a number using the Math.abs()
function.
Using Math.abs()
The simplest and most common way to calculate the absolute value of a number in JavaScript is with the Math.abs()
method. This method is part of the Math
object and returns the absolute value of a given number.
Syntax
Math.abs(x)
x
: A number whose absolute value is to be determined.
Examples
console.log(Math.abs(-5)); // Output: 5 console.log(Math.abs(0)); // Output: 0 console.log(Math.abs(7)); // Output: 7 console.log(Math.abs(-3.14)); // Output: 3.14
If the input is not a number, JavaScript attempts to convert it. If the conversion fails, the result is NaN
(Not a Number):
console.log(Math.abs("10")); // Output: 10 (string is coerced to number) console.log(Math.abs(null)); // Output: 0 console.log(Math.abs("abc")); // Output: NaN
Common Use Cases
-
Distance Calculations: Determine the difference between two numbers regardless of order.
let a = 10; let b = 4; let distance = Math.abs(a - b); // Output: 6
-
Sanitizing Data: Ensure inputs are always positive.
function sanitizePrice(input) { return Math.abs(Number(input)); }
-
Animation and UI: Compute values for smooth transitions or effects.
Conclusion
The Math.abs()
function in JavaScript is a simple but powerful tool for working with absolute values. It's commonly used in mathematical calculations, user input handling, and other real-world scenarios. Always ensure the input is a valid number to avoid unexpected NaN
results.
FAQs
JavaScript tries to convert the string to a number; if it fails, it returns NaN
.
Yes, it returns the absolute value regardless of whether the number is an integer or a float.
Yes, it always returns a non-negative number, even if the input is negative.
We are Leapcell, your top choice for hosting Node.js projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ