How to Check if an Object Is Empty in JavaScript
James Reed
Infrastructure Engineer · Leapcell
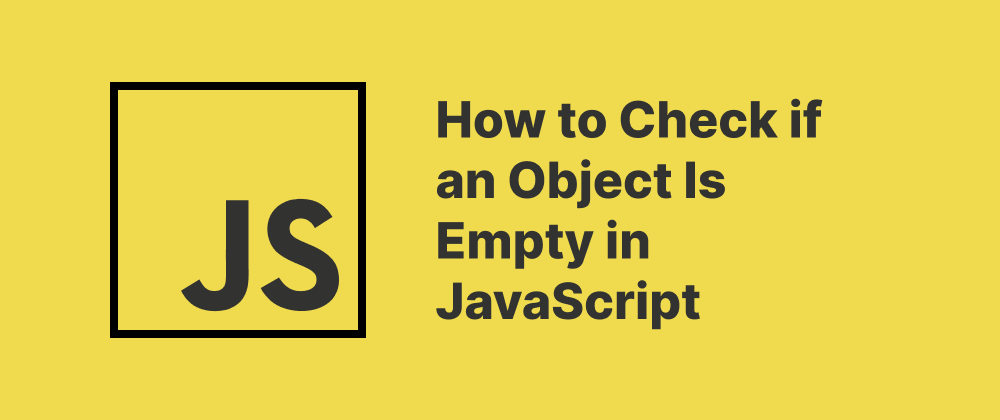
Key Takeaways
- Use
Object.keys(obj).length === 0
as the most reliable way to check for emptiness. - Always validate that the input is a non-null object before checking.
- Avoid using
JSON.stringify()
due to performance and reliability concerns.
When working with JavaScript, it's common to encounter situations where you need to determine whether an object is empty—that is, it has no own properties. Knowing how to perform this check accurately is essential for writing robust and bug-free code. In this article, we’ll explore different methods to check if an object is empty, and when to use each.
What Does "Empty" Mean?
An object is considered "empty" when it has no enumerable own properties. For example:
const obj = {};
This object is empty, while the following is not:
const obj = { name: "Alice" };
Method 1: Using Object.keys()
The most common and reliable way is to use Object.keys()
:
function isEmpty(obj) { return Object.keys(obj).length === 0; }
This method returns an array of the object's own property names. If the length is zero, the object is empty.
Example:
isEmpty({}); // true isEmpty({ a: 1 }); // false
Method 2: Using Object.entries()
You can also use Object.entries()
, which returns an array of key-value pairs:
function isEmpty(obj) { return Object.entries(obj).length === 0; }
This method is equally valid and behaves similarly to Object.keys()
.
Method 3: JSON String Comparison (Not Recommended)
Some developers use JSON serialization to compare:
function isEmpty(obj) { return JSON.stringify(obj) === "{}"; }
While this works in most cases, it’s generally not recommended due to performance concerns and potential edge cases with non-serializable properties like functions or circular references.
Method 4: Manual Iteration with for...in
You can also loop through properties manually:
function isEmpty(obj) { for (let key in obj) { if (obj.hasOwnProperty(key)) { return false; } } return true; }
This method is safe and avoids prototype properties, but is more verbose.
Caveat: Null and Non-Objects
Be careful to check that the value is actually an object and not null
, which is also technically an object in JavaScript:
function isEmpty(obj) { return obj && typeof obj === "object" && Object.keys(obj).length === 0; }
Conclusion
The most reliable and concise way to check if an object is empty in modern JavaScript is using Object.keys(obj).length === 0
. Always ensure the value is truly an object and not null
or another data type to avoid runtime errors.
Summary Table
Method | Recommended | Notes |
---|---|---|
Object.keys().length | ✅ | Simple and fast for most use cases |
Object.entries().length | ✅ | Equivalent alternative |
JSON.stringify() | ❌ | Not performant or safe for all inputs |
for...in loop | ✅ | Safe but more verbose |
By understanding these approaches, you can confidently write code that handles empty objects correctly in any JavaScript environment.
FAQs
Yes, it's efficient and widely supported in modern JavaScript engines.
You should add a type check to ensure it’s a non-null object before evaluating its keys.
It can fail for objects with non-serializable properties and is less performant.
We are Leapcell, your top choice for hosting Node.js projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ