How to Capitalize the First Letter of a String in JavaScript
Grace Collins
Solutions Engineer · Leapcell
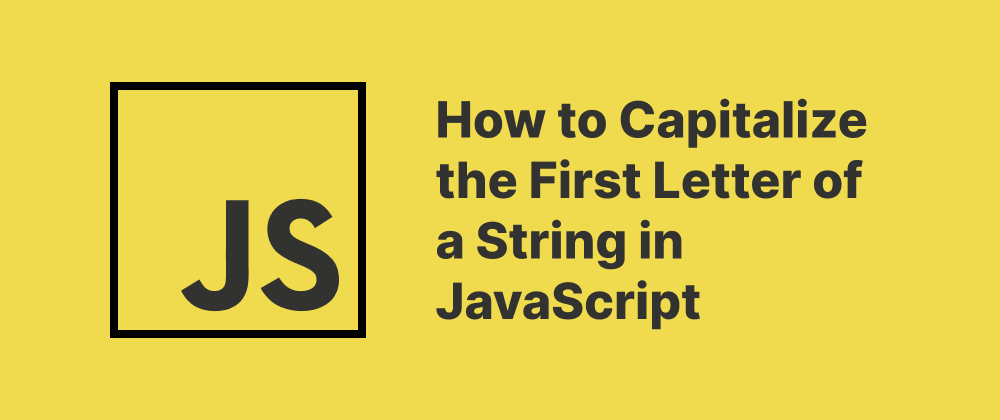
Key Takeaways
- You can capitalize the first letter using
charAt(0).toUpperCase()
combined withslice(1)
. - Always handle empty strings or non-string inputs to avoid errors.
- Use
split()
andmap()
to capitalize each word in a sentence if needed.
In JavaScript, string manipulation is a common task—especially when formatting user input, titles, or messages. One frequent need is capitalizing the first letter of a string. This article walks through simple and effective ways to accomplish this.
Basic Approach Using String Methods
JavaScript provides built-in string methods that make capitalizing the first letter straightforward. Here’s the most common technique:
function capitalizeFirstLetter(str) { if (!str) return ''; return str.charAt(0).toUpperCase() + str.slice(1); }
Explanation:
str.charAt(0)
retrieves the first character..toUpperCase()
converts it to uppercase.str.slice(1)
returns the rest of the string starting from index 1.- The two parts are then concatenated.
Example Usage:
capitalizeFirstLetter('hello'); // "Hello" capitalizeFirstLetter('javaScript'); // "JavaScript"
Handling Edge Cases
You should consider edge cases, such as an empty string, null
, or undefined
:
function safeCapitalize(str) { if (typeof str !== 'string' || str.length === 0) { return ''; } return str[0].toUpperCase() + str.slice(1); }
Capitalizing Every Word (Bonus)
If you want to capitalize the first letter of each word in a sentence, you can use split()
and map()
:
function capitalizeEachWord(sentence) { return sentence .split(' ') .map(word => capitalizeFirstLetter(word)) .join(' '); } capitalizeEachWord('hello world from javascript'); // "Hello World From Javascript"
Summary
Capitalizing the first letter of a string in JavaScript is easy with charAt()
and toUpperCase()
. Always remember to handle invalid input gracefully, and consider writing helper functions if you need to apply the logic across multiple parts of your application.
FAQs
The function should return an empty string or handle the input safely to avoid errors.
The basic version only affects the first letter of the entire string. Use split()
and map()
to handle each word.
No, JavaScript does not have a built-in capitalize()
method—you need to implement it manually.
We are Leapcell, your top choice for hosting Node.js projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ