Understanding Go's `fallthrough`: How and When to Use It
James Reed
Infrastructure Engineer · Leapcell
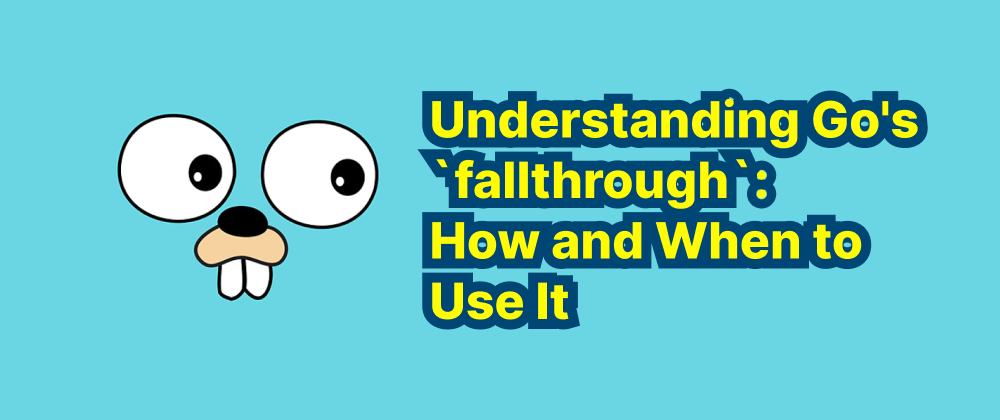
Key Takeaways
- Go’s
fallthrough
explicitly continues execution to the nextcase
, ignoring its condition. fallthrough
must be the last statement in acase
block and cannot be used in the finalcase
.- Overusing
fallthrough
may reduce code readability and is best used sparingly.
Go's switch
statement offers a clean and concise way to handle multiple conditional branches. Unlike some other languages, Go does not automatically fall through from one case to the next. Instead, it requires an explicit fallthrough
statement to continue execution in the subsequent case block. This design choice enhances code clarity and reduces unintended behaviors.
The Basics of fallthrough
In Go, each case
within a switch
statement is evaluated independently. By default, once a matching case
is found and its block is executed, the control exits the switch
statement. However, if you want to execute the code in the next case
regardless of its condition, you can use the fallthrough
keyword.
Here's an example:
package main import "fmt" func main() { number := 2 switch number { case 1: fmt.Println("One") fallthrough case 2: fmt.Println("Two") fallthrough case 3: fmt.Println("Three") } }
Output:
Two
Three
In this example, when number
is 2
, the program prints "Two" and, due to the fallthrough
, also prints "Three", even though the condition for case 3
is not evaluated.
Important Rules for Using fallthrough
-
Positioning: The
fallthrough
statement must be the last statement in acase
block. Placing any code after it will result in a compilation error. -
Unconditional Execution: When
fallthrough
is used, the nextcase
block is executed without evaluating its condition. This means that even if the condition for the nextcase
is false, its block will still run. -
Restrictions:
fallthrough
cannot be used in the finalcase
of aswitch
statement, as there is no subsequentcase
to fall through to. Additionally,fallthrough
is not permitted in type switches.
Practical Use Cases
While fallthrough
is not commonly used, it can be beneficial in certain scenarios:
- Shared Logic: When multiple cases need to execute the same code,
fallthrough
can help avoid code duplication.
switch day := "Friday"; day { case "Monday", "Tuesday", "Wednesday", "Thursday": fmt.Println("Weekday") fallthrough case "Friday": fmt.Println("Almost Weekend") case "Saturday", "Sunday": fmt.Println("Weekend") }
- Sequential Processing: In situations where processing steps are sequential and dependent on each other,
fallthrough
can facilitate the flow.
switch state := "Start"; state { case "Start": fmt.Println("Initializing...") fallthrough case "Process": fmt.Println("Processing...") fallthrough case "End": fmt.Println("Finalizing...") }
Cautionary Notes
-
Readability: Overusing
fallthrough
can make code harder to read and maintain. It's essential to use it judiciously and ensure that its purpose is clear to anyone reading the code. -
Alternative Approaches: Often, combining multiple case values or restructuring the logic can achieve the desired outcome without using
fallthrough
.
switch day := "Monday"; day { case "Monday", "Tuesday", "Wednesday", "Thursday", "Friday": fmt.Println("Weekday") case "Saturday", "Sunday": fmt.Println("Weekend") }
Conclusion
The fallthrough
keyword in Go provides a mechanism to continue execution in the next case
block of a switch
statement, regardless of its condition. While it offers flexibility in certain scenarios, it's crucial to use it appropriately to maintain code clarity and prevent unintended behaviors. Understanding its behavior and constraints ensures that developers can harness its power effectively when needed.
FAQs
No, Go does not fall through by default. You must use the fallthrough
keyword explicitly.
No, fallthrough
is not allowed in type switches, only in value-based switch
statements.
The compiler will raise an error; fallthrough
must be the last statement in the case
block.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ