How to Copy a File in Go (Golang)
James Reed
Infrastructure Engineer · Leapcell
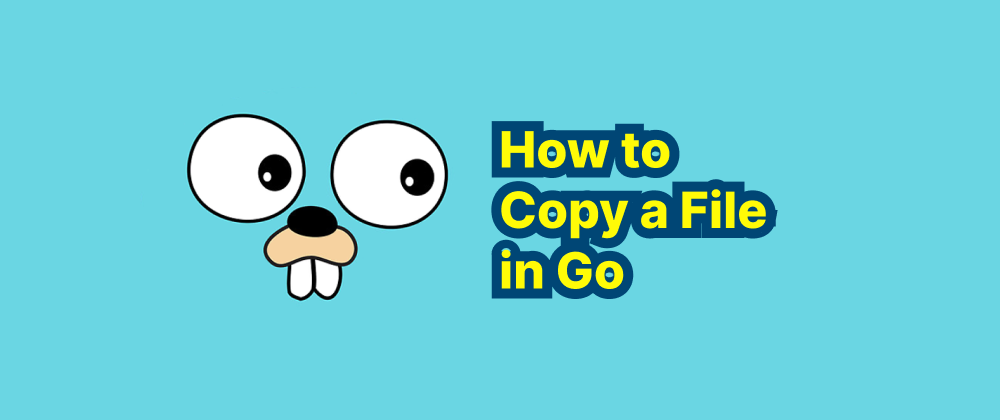
Key Takeaways
- You can copy files in Go using
io.Copy
,os.Open
, andos.Create
. - Proper error handling and resource cleanup (via
defer
) are essential for safe file operations. - To preserve permissions, use
os.Stat
andos.Chmod
after copying.
Copying files is a common task in many applications, whether you're writing logs, processing uploads, or managing local backups. In Go (Golang), the standard library provides a powerful and efficient way to handle file operations, including copying files. This article walks through how to copy a file in Go using idiomatic code and best practices.
Prerequisites
Before diving in, make sure you have:
- Go installed (version 1.16 or higher recommended)
- Basic knowledge of Go syntax
- A working Go development environment
Using io.Copy
to Copy Files
The most straightforward way to copy a file in Go is by using the io.Copy
function from the standard library. Here's a complete example:
package main import ( "fmt" "io" "os" ) func copyFile(src, dst string) error { // Open the source file sourceFile, err := os.Open(src) if err != nil { return fmt.Errorf("failed to open source file: %w", err) } defer sourceFile.Close() // Create the destination file destinationFile, err := os.Create(dst) if err != nil { return fmt.Errorf("failed to create destination file: %w", err) } defer destinationFile.Close() // Copy the content _, err = io.Copy(destinationFile, sourceFile) if err != nil { return fmt.Errorf("failed to copy file: %w", err) } // Flush file metadata to disk err = destinationFile.Sync() if err != nil { return fmt.Errorf("failed to sync destination file: %w", err) } return nil } func main() { err := copyFile("example.txt", "copy.txt") if err != nil { fmt.Println("Error:", err) } else { fmt.Println("File copied successfully.") } }
Explanation
os.Open()
opens the source file for reading.os.Create()
creates (or truncates) the destination file.io.Copy()
efficiently transfers the data from the source to the destination.defer
is used to ensure that file handles are properly closed.Sync()
ensures the data is flushed to disk, reducing the risk of data loss in case of unexpected shutdown.
Error Handling
Note the use of %w
in fmt.Errorf
to wrap errors. This allows error unwrapping later with errors.Is()
or errors.As()
if needed, which is particularly useful in larger applications for debugging and error tracing.
Bonus: Preserving File Permissions
If you want to preserve the original file's permissions, you can extend the function slightly:
import "io/fs" // After copying and syncing: info, err := os.Stat(src) if err != nil { return fmt.Errorf("failed to get source file info: %w", err) } err = os.Chmod(dst, info.Mode().Perm()) if err != nil { return fmt.Errorf("failed to set file permissions: %w", err) }
Conclusion
Copying files in Go is simple and efficient with the standard library. Whether you're working on CLI tools, web servers, or file management applications, understanding how to perform file I/O properly is essential.
For more advanced use cases—such as copying large directories, handling symbolic links, or preserving metadata—you may want to explore third-party libraries like spf13/afero or write custom recursive logic.
FAQs
io.Copy
is efficient and handles buffered I/O internally, making the code simpler and faster.
Use file.Sync()
on the destination file to flush OS buffers to disk.
Use os.Stat()
to get the original mode and os.Chmod()
to apply it to the new file.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ