Rust’s Copy vs. Clone: What's the Difference?
Grace Collins
Solutions Engineer · Leapcell
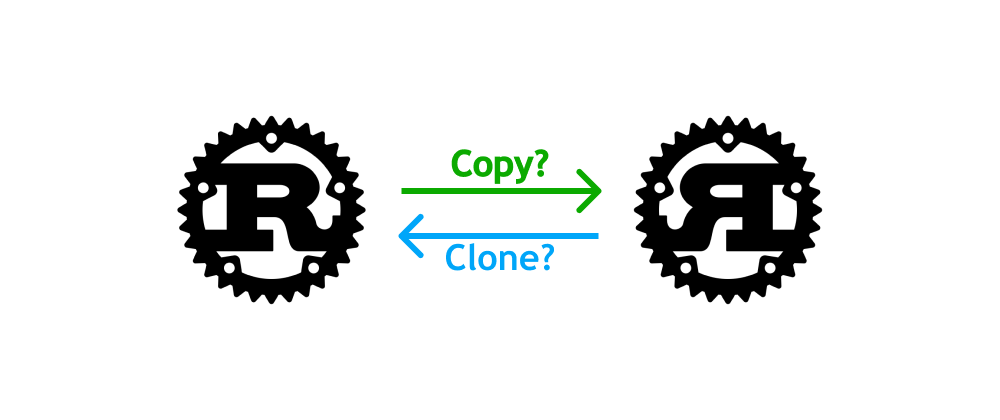
Good Brothers: Copy and Clone in Rust
In Rust, the Copy
and Clone
traits control the copying behavior of types. They allow you to define how values of a type are copied and under what circumstances copying is allowed. This article will introduce the purpose and usage of these two traits in detail, along with code examples demonstrating their usage.
The Copy
Trait
The Copy
trait signifies that a type can be copied bit by bit. When a type implements the Copy
trait, its values are automatically duplicated when assigned, passed as arguments, or returned.
What is the Copy
Trait?
The Copy
trait is a marker trait, meaning it does not define any methods. It simply marks a type as being eligible for bitwise copying.
#[derive(Copy)] struct Point { x: i32, y: i32, }
How to Implement the Copy
Trait?
To implement the Copy
trait, you need to add the #[derive(Copy)]
attribute to the type definition. Additionally, the type must also implement the Clone
trait because all types that implement Copy
must also implement Clone
.
#[derive(Copy, Clone)] struct Point { x: i32, y: i32, }
If you attempt to implement Copy
without also implementing Clone
, the compiler will throw an error:
#[derive(Copy)] struct Point { x: i32, y: i32, } // error[E0277]: the trait bound `Point: std::clone::Clone` is not satisfied
The error message indicates that the Point
type does not implement the Clone
trait, and therefore it cannot implement Copy
.
This requirement exists because all Copy
types must also implement Clone
. When you explicitly call the clone
method, Rust assumes you intend to create a copy and wants to ensure that copying behavior is well-defined. Thus, if you want to implement Copy
, you must also implement Clone
.
Which Types Can Implement Copy
?
Not all types can implement Copy
. Only types that meet the following criteria are eligible:
- The type itself is a Plain Old Data (POD) type, meaning it does not contain any pointers or references.
- All fields of the type must also implement
Copy
.
For example, the following type cannot implement Copy
because it contains a reference field:
struct Foo<'a> { x: &'a i32, } // error[E0204]: the trait `Copy` may not be implemented for this type impl Copy for Foo<'_> {}
Why Do We Need the Copy
Trait?
The Copy
trait allows control over a type’s copying behavior. When a type implements Copy
, its values are automatically duplicated upon assignment, function parameter passing, and returning. This eliminates the need to explicitly call clone()
to copy values.
Moreover, since Copy
types always undergo bitwise copying, the performance overhead is minimal. This is particularly useful for optimizing performance in Rust programs.
The Clone
Trait
Unlike Copy
, the Clone
trait allows explicit copying of a type’s value. When a type implements Clone
, you can call its clone()
method to create a new instance.
What is the Clone
Trait?
Unlike Copy
, Clone
is a regular trait that contains a method: clone()
. This method is responsible for creating a new copy of the value.
#[derive(Clone)] struct Point { x: i32, y: i32, }
How to Implement the Clone
Trait?
To implement the Clone
trait, you can either add the #[derive(Clone)]
attribute or manually implement the clone()
method.
#[derive(Clone)] struct Point { x: i32, y: i32, } // Manually implementing the `clone()` method impl Clone for Point { fn clone(&self) -> Self { Self { x: self.x, y: self.y } } }
Which Types Can Implement Clone
?
Almost all types can implement Clone
. As long as you can define how to create a new copy of a value, you can implement Clone
.
Why Do We Need the Clone
Trait?
The Clone
trait allows explicit duplication of values. This is particularly useful for types that cannot be bitwise copied, such as those containing pointers or references.
Additionally, Clone
allows customization of the copying process. You can add any necessary logic inside the clone()
method to perform specific actions during copying.
Differences and Relationship Between Copy
and Clone
Both Copy
and Clone
control how types are copied, but they have key differences:
Copy
is a marker trait, indicating that a type supports bitwise copying. When a type implementsCopy
, its values are automatically duplicated upon assignment, passing as function arguments, and returning.Clone
is a regular trait that contains a method:clone()
. When a type implementsClone
, you can explicitly callclone()
to create a new copy.
Additionally, all Copy
types must also implement Clone
. This ensures that when you explicitly call clone()
, Rust assumes you know what you are doing and allows bitwise copying.
Example Analysis
Below is an example demonstrating the usage of Copy
and Clone
:
#[derive(Copy, Clone)] struct Point { x: i32, y: i32, } fn main() { let p1 = Point { x: 1, y: 2 }; let p2 = p1; // Automatically copied let p3 = p1.clone(); // Explicitly copied }
In this example, we define a Point
type and implement both the Copy
and Clone
traits. In the main
function, we create a Point
value and assign it to another variable. Since Point
implements Copy
, the assignment operation automatically duplicates the value. Additionally, we explicitly call clone()
to create another copy of the value.
We are Leapcell, your top choice for hosting Rust projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ