Python Cheat Sheet: A Quick Guide to Core Syntax and Features
Grace Collins
Solutions Engineer · Leapcell
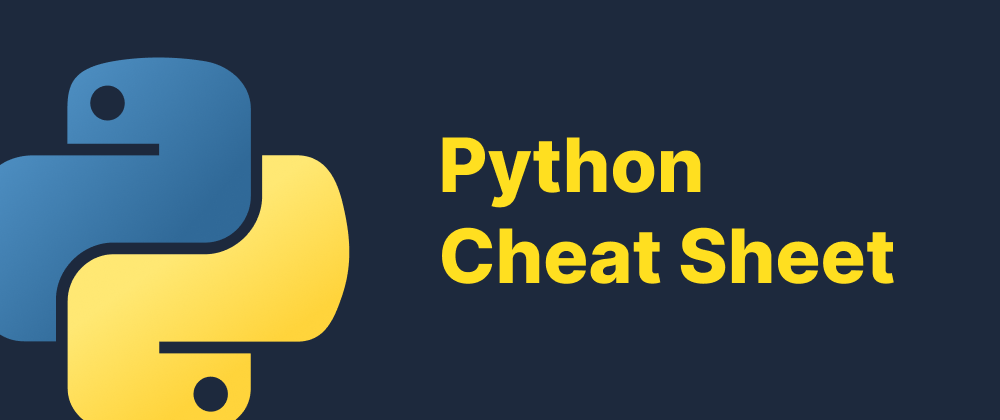
Key Takeaways
- Python has simple, readable syntax ideal for beginners.
- Core structures like lists, dictionaries, and loops are essential to master.
- Understanding basic file handling and exceptions improves code robustness.
Python is a versatile and widely-used programming language known for its readability and efficiency. This cheat sheet provides a quick reference to essential Python concepts, syntax, and commands.
Basic Syntax
Printing Output
To display output in Python, use the print()
function:
print("Hello, World!")
Comments
Use the #
symbol to add comments in your code:
# This is a single-line comment """ This is a multi-line comment. """
Variables and Data Types
Variables
Assign values to variables using the =
operator:
message = "Hello, Python!"
Data Types
Common data types in Python include:
- Integer (
int
): Whole numbers, e.g.,42
- Float (
float
): Decimal numbers, e.g.,3.14
- String (
str
): Sequence of characters, e.g.,"Python"
- Boolean (
bool
):True
orFalse
Control Flow
Conditional Statements
Use if
, elif
, and else
to execute code based on conditions:
x = 10 if x > 0: print("Positive") elif x == 0: print("Zero") else: print("Negative")
Loops
For Loop
Iterate over a sequence (like a list or range):
for i in range(5): print(i)
While Loop
Execute a block of code as long as a condition is true:
count = 0 while count < 5: print(count) count += 1
Functions
Define reusable blocks of code using functions:
def greet(name): return f"Hello, {name}!" print(greet("Alice"))
Data Structures
Lists
Ordered, mutable collections:
fruits = ["apple", "banana", "cherry"] fruits.append("date") # Adds "date" to the list
Tuples
Ordered, immutable collections:
coordinates = (4, 5)
Dictionaries
Unordered collections of key-value pairs:
person = {"name": "Alice", "age": 30} print(person["name"]) # Outputs: Alice
Sets
Unordered collections of unique elements:
unique_numbers = {1, 2, 3, 3, 4} print(unique_numbers) # Outputs: {1, 2, 3, 4}
File Handling
Read from and write to files using the open()
function:
# Writing to a file with open("example.txt", "w") as file: file.write("Hello, File!") # Reading from a file with open("example.txt", "r") as file: content = file.read() print(content)
Exception Handling
Manage errors gracefully using try
and except
blocks:
try: result = 10 / 0 except ZeroDivisionError: print("Cannot divide by zero!")
This cheat sheet covers fundamental aspects of Python programming. For more detailed information, consider exploring official Python documentation and other comprehensive resources.
FAQs
Use #
for single-line comments and triple quotes ("""
) for multi-line explanations.
Use the def
keyword followed by the function name and parameters.
Start with lists, dictionaries, tuples, and sets for foundational knowledge.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ