Golang String Comparison: A Comprehensive Guide
Lukas Schneider
DevOps Engineer · Leapcell
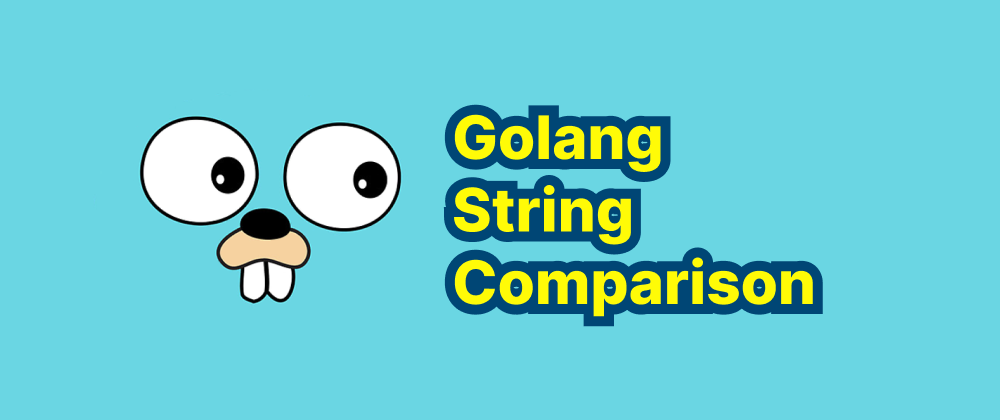
Key Takeaways
- Use
==
for efficient equality checks and<
,>
for lexicographical comparison. - Use
strings.EqualFold
for case-insensitive string comparison. bytes.Compare
is useful for performance-critical string comparisons.
String comparison is a fundamental operation in any programming language, and Golang (or Go) provides multiple ways to compare strings efficiently. In this article, we will explore different methods of comparing strings in Go, covering equality checks, lexicographical comparisons, case-insensitive comparisons, and performance considerations.
1. Comparing Strings for Equality
The simplest way to compare two strings in Go is using the ==
and !=
operators. These operators check whether two strings are identical in terms of content.
Example:
package main import "fmt" func main() { str1 := "hello" str2 := "hello" str3 := "world" fmt.Println(str1 == str2) // true fmt.Println(str1 == str3) // false fmt.Println(str1 != str3) // true }
The ==
operator returns true
if both strings have the same sequence of characters; otherwise, it returns false
.
2. Lexicographical String Comparison
Golang allows lexicographical (dictionary order) string comparison using relational operators such as <
, <=
, >
, and >=
.
Example:
package main import "fmt" func main() { str1 := "apple" str2 := "banana" fmt.Println(str1 < str2) // true fmt.Println(str1 > str2) // false fmt.Println(str1 <= str2) // true fmt.Println(str1 >= str2) // false }
Here, "apple"
is considered smaller than "banana"
because, in Unicode order, "a"
comes before "b"
.
3. Case-Insensitive String Comparison
Since ==
is case-sensitive, comparing "Go"
and "go"
directly will return false
. To perform a case-insensitive comparison, we can use the strings.EqualFold
function from the strings
package.
Example:
package main import ( "fmt" "strings" ) func main() { str1 := "Go" str2 := "go" fmt.Println(strings.EqualFold(str1, str2)) // true }
strings.EqualFold
ignores case differences and returns true
if the strings are equivalent.
4. Comparing Strings with bytes.Compare
The bytes.Compare
function provides another efficient way to compare strings, especially when performance matters. While bytes.Compare
is primarily used for byte slices, it can also be used for string comparison after converting strings to byte slices.
Example:
package main import ( "bytes" "fmt" ) func main() { str1 := "hello" str2 := "world" result := bytes.Compare([]byte(str1), []byte(str2)) if result == 0 { fmt.Println("Strings are equal") } else if result < 0 { fmt.Println("First string is smaller") } else { fmt.Println("First string is larger") } }
This method is useful when dealing with large amounts of data, as bytes.Compare
is optimized for performance.
5. Performance Considerations
- Direct comparison (
==
) is the most efficient way to check equality, as Go internally optimizes string comparison. - Lexicographical comparison (
<
,>
) is useful when sorting strings or implementing custom ordering logic. strings.EqualFold
is ideal for case-insensitive comparison but may be slower than==
due to additional processing.bytes.Compare
is efficient for large-scale comparisons and when dealing with byte slices instead of strings.
Conclusion
Go provides multiple ways to compare strings depending on the use case. The ==
operator is the simplest and most efficient for equality checks, while relational operators (<
, >
) help with ordering. For case-insensitive comparison, strings.EqualFold
is a reliable choice, and bytes.Compare
is useful for performance-critical applications. Understanding these methods allows developers to write more efficient and readable Go code.
FAQs
Using ==
for equality checks is the most efficient.
Use strings.EqualFold(str1, str2)
.
Use it when comparing large byte slices or optimizing performance.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ