How to Minify JSON: A Developer’s Guide
Grace Collins
Solutions Engineer · Leapcell
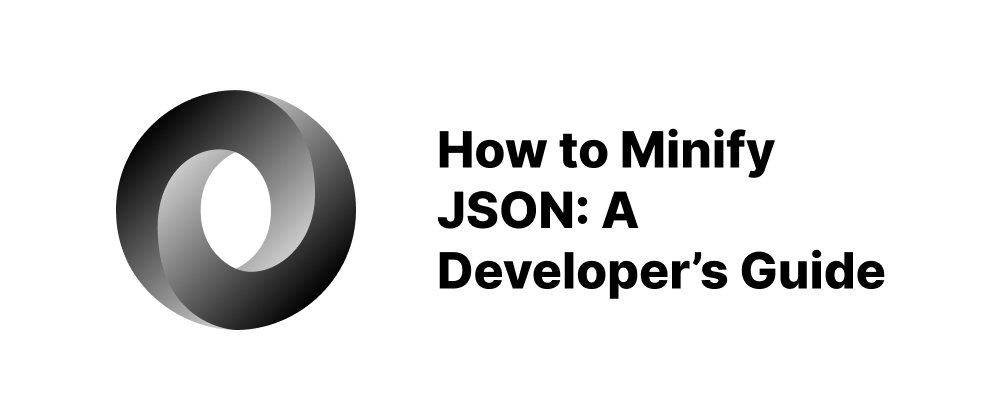
Key Takeaways
- JSON minification reduces file size by removing unnecessary characters.
- It improves performance in web and API contexts.
- You can minify JSON using code or online tools.
What Is JSON Minification?
JSON minification is the process of removing unnecessary characters—such as whitespace, line breaks, and comments—from a JSON file without altering its data structure. This results in a more compact file that is faster to transmit and parse, making it ideal for web applications, APIs, and data storage.([Bito][1])
Why Minify JSON?
Minifying JSON offers several benefits:
-
Reduced File Size: Smaller files lead to quicker downloads and lower bandwidth usage.
-
Improved Performance: Minified JSON is parsed faster by browsers and servers.([在线JSON格式化工具][2])
-
Enhanced Security: Removing comments and extraneous formatting can help prevent the accidental exposure of sensitive information. ([Json Minify][3])
How to Minify JSON
1. Using JavaScript
In JavaScript, you can minify JSON by parsing and then re-stringifying the data:
const originalJson = `{ "name": "John Doe", "email": "john@example.com", "active": true }`; const minifiedJson = JSON.stringify(JSON.parse(originalJson)); console.log(minifiedJson); // Output: {"name":"John Doe","email":"john@example.com","active":true}
This method removes all unnecessary whitespace and formatting. ([在线JSON格式化工具][2])
2. Using Online Tools
Several online tools can minify JSON without requiring any programming knowledge:([DZone][4])
-
Site24x7 JSON Minifier: Paste your JSON code and click "Minify" to get the compact version. ([Json Minify][3])
-
JSONFormatter.org: Offers both minify and beautify options for JSON data.
-
Code Beautify JSON Minifier: Allows you to upload a file or paste JSON code to minify it. ([在线压缩JS和CSS][5])
3. Using Other Programming Languages
Most programming languages provide libraries or functions to handle JSON minification:
- Python:
import json original_json = { "name": "John Doe", "email": "john@example.com", "active": True } minified_json = json.dumps(original_json, separators=(',', ':')) print(minified_json) # Output: {"name":"John Doe","email":"john@example.com","active":true}
- PHP:
<?php $original_json = array( "name" => "John Doe", "email" => "john@example.com", "active" => true ); $minified_json = json_encode($original_json); echo $minified_json; // Output: {"name":"John Doe","email":"john@example.com","active":true} ?>
- Terraform:
variable "json_text" { default = <<EOF { "name": "John Doe", "email": "john@example.com", "active": true } EOF } output "minified" { value = jsonencode(jsondecode(var.json_text)) }
This approach uses jsondecode
and jsonencode
to produce minified JSON. ([HashiCorp Help Center][6])
Best Practices
-
Validate JSON Before Minifying: Ensure your JSON is valid to prevent errors during minification.
-
Use Minification in Production: Minify JSON files in production environments to improve performance.
-
Keep Original Files for Debugging: Maintain unminified versions of JSON files for easier debugging and development.
Conclusion
Minifying JSON is a straightforward process that can significantly improve the efficiency of data transmission and parsing in your applications. Whether you choose to use built-in language functions or online tools, incorporating JSON minification into your workflow is a best practice for modern web development.([在线压缩JS和CSS][5])
FAQs
It's the process of compressing JSON by removing whitespace and formatting without changing its data.
To reduce size, speed up data transmission, and enhance performance.
Yes, many free online tools allow you to paste and minify JSON easily.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ