How to Convert a Python Dictionary to JSON
James Reed
Infrastructure Engineer · Leapcell
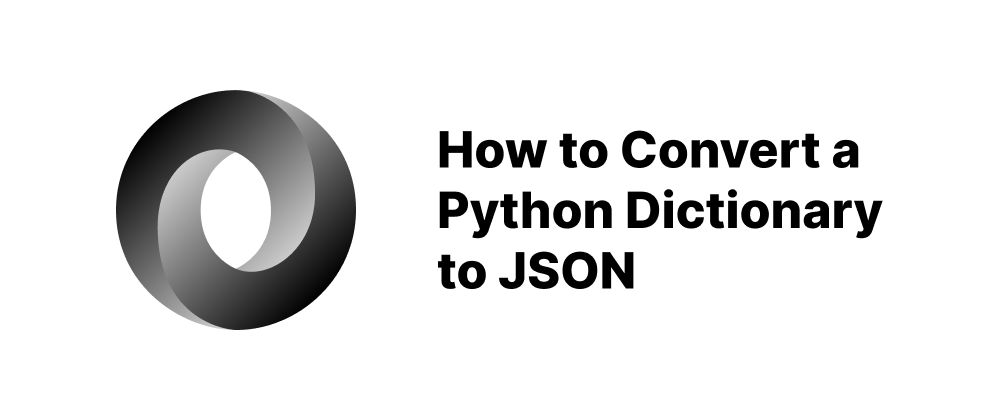
Key Takeaways
- Use
json.dumps()
to convert a Python dictionary into a JSON-formatted string. - Use
json.dump()
to write JSON data directly to a file. - The
indent
parameter improves JSON readability with pretty-printing.
When working with Python, especially in web development or data exchange, converting a dictionary to JSON (JavaScript Object Notation) is a common and essential task. JSON is a lightweight data-interchange format that is easy for humans to read and write, and easy for machines to parse and generate.
In this article, we will explore how to convert a Python dictionary into a JSON string using the built-in json
module, along with practical examples and tips.
Importing the json
Module
Python provides a standard library called json
that enables you to encode and decode JSON data easily. Before you can use it, you need to import it:
import json
Creating a Python Dictionary
Let’s start with a sample dictionary:
data = { "name": "Alice", "age": 30, "is_employee": True, "departments": ["HR", "Finance"], "details": { "position": "Manager", "full_time": True } }
Converting Dictionary to JSON String
You can use the json.dumps()
method to convert a Python dictionary to a JSON-formatted string.
json_string = json.dumps(data) print(json_string)
Output:
{"name": "Alice", "age": 30, "is_employee": true, "departments": ["HR", "Finance"], "details": {"position": "Manager", "full_time": true}}
Note that:
- Python’s
True
andFalse
becometrue
andfalse
in JSON. - The dictionary is converted to a single-line JSON string by default.
Pretty-Printing JSON
To make the output more readable, you can use the indent
parameter:
json_string = json.dumps(data, indent=4) print(json_string)
Output:
{ "name": "Alice", "age": 30, "is_employee": true, "departments": [ "HR", "Finance" ], "details": { "position": "Manager", "full_time": true } }
Writing JSON to a File
If you want to save the JSON data to a file, use json.dump()
instead of dumps()
:
with open("data.json", "w") as file: json.dump(data, file, indent=4)
Conclusion
Converting a Python dictionary to JSON is straightforward with the json
module. You can serialize your data for APIs, configuration files, or data storage with just a few lines of code. Remember to use dumps()
for strings and dump()
for writing directly to files, and leverage parameters like indent
to improve readability.
FAQs
dumps()
returns a string; dump()
writes directly to a file.
Python True
and False
become true
and false
in JSON.
Yes, use the indent
parameter in dumps()
or dump()
.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ