How to Convert HTML Form Data to JSON Using JavaScript
James Reed
Infrastructure Engineer · Leapcell
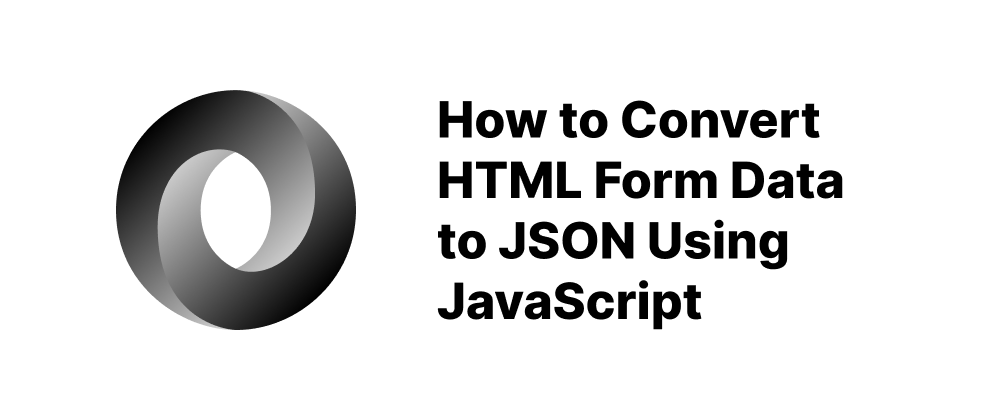
Key Takeaways
FormData
andObject.fromEntries()
offer a quick way to get JSON from forms.- Use
getAll()
or manual loops to handle multiple fields with the same name. - Always validate and process JSON before sending to a server.
Converting HTML form data into JSON format is a common task in web development, especially when interacting with APIs or storing data locally. This guide will walk you through various methods to achieve this using JavaScript.
Why Convert Form Data to JSON?
- API Integration: Many modern APIs accept data in JSON format.
- Data Storage: JSON is a lightweight format ideal for storing structured data.
- Consistency: Using JSON ensures uniformity across different parts of your application.
Method 1: Using FormData
and Object.fromEntries()
The FormData
API provides a convenient way to capture form data. Combined with Object.fromEntries()
, you can easily convert this data into a JSON object.
Example:
<form id="myForm"> <label> Name: <input type="text" name="name" /> </label> <label> Email: <input type="email" name="email" /> </label> <button type="submit">Submit</button> </form> <script> const form = document.getElementById('myForm'); form.addEventListener('submit', function (event) { event.preventDefault(); // Prevent default form submission const formData = new FormData(form); const jsonData = Object.fromEntries(formData.entries()); console.log(jsonData); // To convert to JSON string: // console.log(JSON.stringify(jsonData)); }); </script>
Note: This method works well for simple forms but may not handle multiple inputs with the same name (e.g., checkboxes) as expected.
Method 2: Handling Multiple Inputs with the Same Name
For form elements like checkboxes or multi-select fields, where multiple inputs share the same name, FormData.getAll()
can be used to retrieve all values.
Example:
<form id="myForm"> <label> Name: <input type="text" name="name" /> </label> <label> Email: <input type="email" name="email" /> </label> <fieldset> <legend>Topics:</legend> <label> <input type="checkbox" name="topics" value="JavaScript" /> JavaScript </label> <label> <input type="checkbox" name="topics" value="HTML" /> HTML </label> <label> <input type="checkbox" name="topics" value="CSS" /> CSS </label> </fieldset> <button type="submit">Submit</button> </form> <script> const form = document.getElementById('myForm'); form.addEventListener('submit', function (event) { event.preventDefault(); const formData = new FormData(form); const jsonData = Object.fromEntries(formData.entries()); // Handle multiple selections jsonData.topics = formData.getAll('topics'); console.log(jsonData); }); </script>
Output Example:
{ "name": "Alice", "email": "alice@example.com", "topics": ["JavaScript", "CSS"] }
Method 3: Manual Conversion Using forEach()
For more control over the conversion process, you can manually iterate over the FormData
entries.
Example:
<form id="myForm"> <label> Name: <input type="text" name="name" /> </label> <label> Email: <input type="email" name="email" /> </label> <fieldset> <legend>Topics:</legend> <label> <input type="checkbox" name="topics" value="JavaScript" /> JavaScript </label> <label> <input type="checkbox" name="topics" value="HTML" /> HTML </label> <label> <input type="checkbox" name="topics" value="CSS" /> CSS </label> </fieldset> <button type="submit">Submit</button> </form> <script> const form = document.getElementById('myForm'); form.addEventListener('submit', function (event) { event.preventDefault(); const formData = new FormData(form); const jsonData = {}; formData.forEach((value, key) => { if (jsonData.hasOwnProperty(key)) { // If the key already exists, convert it to an array or append to the existing array jsonData[key] = [].concat(jsonData[key], value); } else { jsonData[key] = value; } }); console.log(jsonData); }); </script>
This approach ensures that multiple values for the same key are captured correctly.
Additional Considerations
- Checkbox Values: When a checkbox is checked, its value is included; otherwise, it's omitted. Ensure to handle unchecked checkboxes if necessary.
- File Inputs:
FormData
captures file inputs asFile
objects. You may need to handle file uploads separately. - Validation: Always validate form data before processing or sending it to a server.
Conclusion
Converting HTML form data to JSON in JavaScript can be achieved through various methods, each suited to different scenarios. The FormData
API combined with Object.fromEntries()
offers a concise solution for simple forms, while manual iteration provides greater flexibility for complex forms.
FAQs
Yes, but you must use getAll()
or loop manually to collect all selected values.
Unchecked checkboxes are not included in FormData
; handle them explicitly if needed.
FormData
includes file objects, but they must be processed separately from JSON.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ