Converting Byte Array to String in Go
James Reed
Infrastructure Engineer · Leapcell
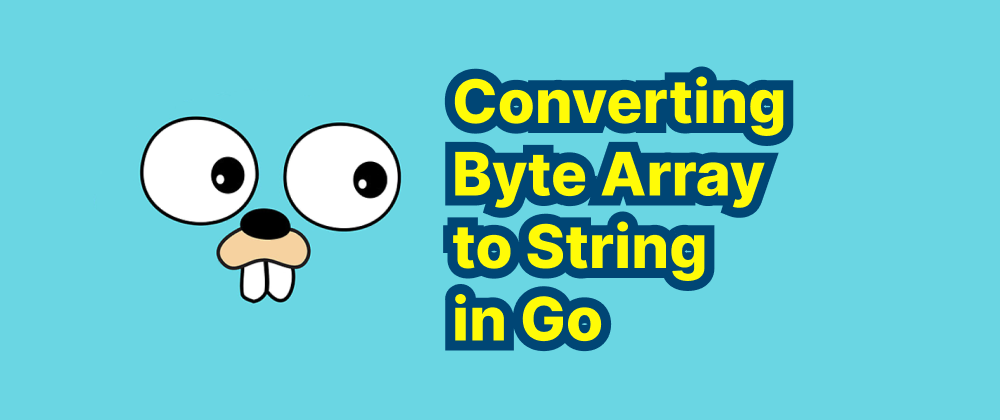
Key Takeaways
- Converting
[]byte
tostring
and vice versa in Go involves memory allocation and copying. - Performance optimizations, such as zero-copy conversions, should be used carefully.
- The
unsafe
package can enable efficient conversions but risks memory safety.
In Go, converting between byte arrays ([]byte
) and strings is a common operation, especially when dealing with data encoding, file I/O, or network communication. Understanding the methods and implications of these conversions is crucial for writing efficient and effective Go code.
Understanding Strings and Byte Arrays in Go
In Go, a string
is a read-only slice of bytes. This means that while you can view and manipulate the data within a string, you cannot modify its contents directly. On the other hand, a byte array ([]byte
) is a mutable sequence of bytes, allowing for modifications.
Converting []byte
to string
The most straightforward way to convert a byte array to a string is by using a simple type conversion:
byteArray := []byte{'H', 'e', 'l', 'l', 'o'} str := string(byteArray) fmt.Println(str) // Output: Hello
This method creates a new string by copying the data from the byte array. It's important to note that this conversion involves allocating new memory for the string, which can have performance implications if done frequently or with large byte arrays.
Converting string
to []byte
Similarly, you can convert a string to a byte array using:
str := "Hello" byteArray := []byte(str) fmt.Println(byteArray) // Output: [72 101 108 108 111]
This conversion also results in a memory allocation, as it creates a new byte array containing the data from the string.
Performance Considerations
Since both conversions involve memory allocation and data copying, they can impact performance, especially in high-throughput scenarios or when dealing with large datasets. To mitigate this, it's advisable to minimize unnecessary conversions and reuse byte arrays or strings when possible.
Zero-Copy Conversions
Starting from Go 1.22, the Go compiler introduced an optimization that allows zero-copy conversions from string
to []byte
under certain conditions. If the resulting byte slice does not escape to the heap and is only read (not modified), the compiler can optimize away the allocation. However, this optimization does not apply to conversions from []byte
to string
. Therefore, developers should still be cautious and not rely solely on the compiler for performance improvements.
Unsafe Conversions
For scenarios where performance is critical, and you are certain about the immutability and lifecycle of the data, you can use the unsafe
package to perform zero-copy conversions. However, this approach is risky and can lead to undefined behavior if not handled carefully. Here's an example:
import ( "reflect" "unsafe" ) func bytesToString(b []byte) string { return *(*string)(unsafe.Pointer(&b)) }
Warning: Using the unsafe
package bypasses Go's safety guarantees. Ensure that the byte slice is not modified after the conversion, and be aware of potential issues with garbage collection and memory management.
Conclusion
Converting between byte arrays and strings in Go is straightforward but comes with considerations regarding memory allocation and performance. While simple type conversions are easy to implement, they involve data copying. Advanced techniques, such as zero-copy conversions using the unsafe
package, should be used with caution due to potential risks. Always profile your application to understand the impact of these conversions and choose the method that best balances safety and performance for your specific use case.
FAQs
Yes, it copies the byte slice data into a new string.
Yes, Go 1.22 introduced optimizations for certain cases where the conversion does not escape.
No, unless performance is critical and you fully understand the risks of memory safety issues.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ