How to Pretty Print JSON in Go
Grace Collins
Solutions Engineer · Leapcell
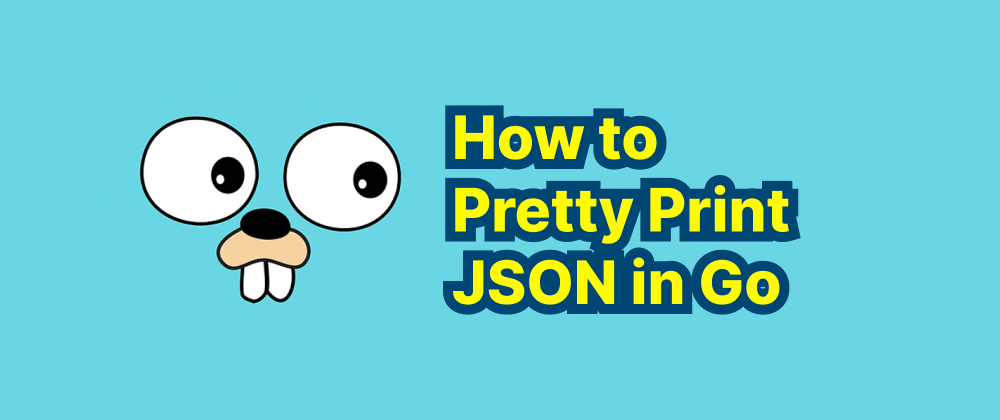
Key Takeaways
- Use
json.MarshalIndent
to format Go data structures into readable JSON. json.Indent
can be used to pretty print existing JSON strings.json.Encoder
provides a memory-efficient way to format JSON output.
In Go, "pretty printing" JSON refers to formatting JSON data with indentation and line breaks to enhance readability. This is particularly useful when displaying JSON in logs or console outputs. Go's standard library provides built-in support for this through the encoding/json
package.
Using json.MarshalIndent
to Pretty Print JSON
The encoding/json
package offers the MarshalIndent
function, which serializes Go data structures into JSON with indentation. Here's how to use it:
package main import ( "encoding/json" "fmt" ) func main() { // Sample data structure data := map[string]interface{}{ "Name": "Alice", "Age": 30, "Active": true, "Skills": []string{"Go", "Docker", "Kubernetes"}, } // Convert to pretty-printed JSON jsonData, err := json.MarshalIndent(data, "", " ") if err != nil { fmt.Println("Error marshalling JSON:", err) return } // Print the formatted JSON fmt.Println(string(jsonData)) }
In this example:
data
is a map representing a sample data structure.json.MarshalIndent
serializesdata
into a JSON-formatted byte slice with indentation.- The second argument (
""
) specifies the prefix for each line (none in this case). - The third argument (
" "
) specifies the indentation string (four spaces here). - The resulting
jsonData
is converted to a string and printed.
The output will be:
{ "Active": true, "Age": 30, "Name": "Alice", "Skills": [ "Go", "Docker", "Kubernetes" ] }
Pretty Printing Existing JSON Strings
If you have a JSON string and wish to pretty print it, you can use the json.Indent
function:
package main import ( "bytes" "encoding/json" "fmt" ) func main() { // Minified JSON string jsonString := `{"Name":"Alice","Age":30,"Active":true,"Skills":["Go","Docker","Kubernetes"]}` // Convert string to byte slice jsonData := []byte(jsonString) // Create a buffer to hold the formatted JSON var prettyJSON bytes.Buffer // Indent the JSON err := json.Indent(&prettyJSON, jsonData, "", " ") if err != nil { fmt.Println("Error indenting JSON:", err) return } // Print the formatted JSON fmt.Println(prettyJSON.String()) }
In this code:
jsonString
contains the minified JSON data.json.Indent
reads the minified JSON and writes a formatted version toprettyJSON
.- The formatted JSON is then printed as a string.
The output will be identical to the previous example.
Using json.Encoder
with Indentation
Alternatively, you can use json.Encoder
to pretty print JSON directly to an io.Writer
, such as os.Stdout
:
package main import ( "encoding/json" "os" ) func main() { // Sample data structure data := map[string]interface{}{ "Name": "Alice", "Age": 30, "Active": true, "Skills": []string{"Go", "Docker", "Kubernetes"}, } // Create a JSON encoder with indentation encoder := json.NewEncoder(os.Stdout) encoder.SetIndent("", " ") // Encode and print the data err := encoder.Encode(data) if err != nil { fmt.Println("Error encoding JSON:", err) } }
This approach is efficient when you want to write formatted JSON directly to an output stream without holding it in memory.
Conclusion
Go's encoding/json
package provides multiple methods to pretty print JSON data, enhancing readability in logs and console outputs. Whether using json.MarshalIndent
, json.Indent
, or json.Encoder
with indentation, you can choose the method that best fits your use case.
FAQs
json.MarshalIndent
formats Go data structures into JSON, while json.Indent
reformats existing JSON strings.
Use json.Encoder
when writing directly to an io.Writer
to avoid unnecessary memory usage.
Yes, the third argument in json.MarshalIndent
specifies the indentation string (e.g., spaces or tabs).
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ