How to Convert Between `int64` and `string` in Golang
Grace Collins
Solutions Engineer · Leapcell
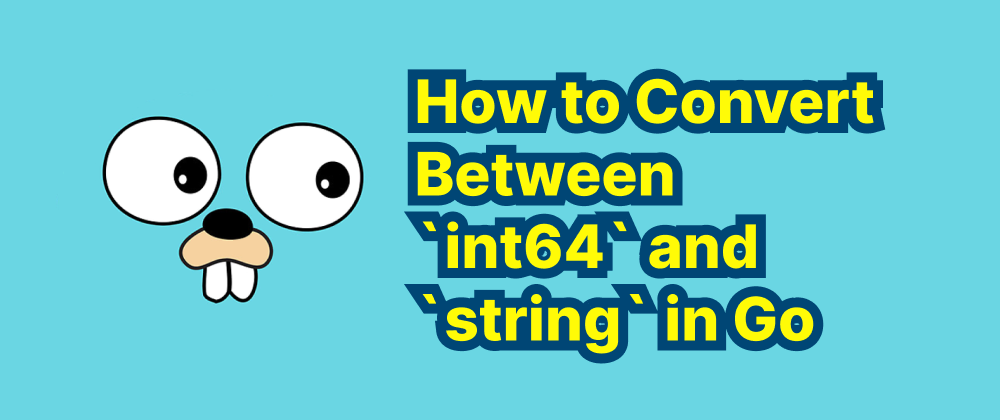
Key Takeaways
- Use
strconv.FormatInt
orfmt.Sprintf
to convertint64
tostring
. - Use
strconv.ParseInt
to convertstring
toint64
, handling errors properly. strconv
functions are more efficient, whilefmt.Sprintf
offers formatting flexibility.
How to Convert Between int64
and string
in Golang
In Golang, converting between int64
and string
is a common task when dealing with user input, data storage, or serialization. This article will cover various methods to convert int64
to string
and vice versa.
Convert int64
to string
There are multiple ways to convert an int64
to a string
in Go, depending on the use case.
Using strconv.FormatInt
The strconv
package provides the FormatInt
function to convert an int64
to a string with a specified base.
package main import ( "fmt" "strconv" ) func main() { num := int64(1234567890) str := strconv.FormatInt(num, 10) // Convert int64 to decimal string fmt.Println(str) // Output: "1234567890" }
Using fmt.Sprintf
Another approach is using fmt.Sprintf
, which is more flexible and commonly used when formatting output.
package main import ( "fmt" ) func main() { num := int64(1234567890) str := fmt.Sprintf("%d", num) // Format int64 as a decimal string fmt.Println(str) // Output: "1234567890" }
The fmt.Sprintf
function is useful when formatting numbers with additional text.
str := fmt.Sprintf("The number is %d", num) fmt.Println(str) // Output: "The number is 1234567890"
Convert string
to int64
To convert a string
back to int64
, we primarily use the strconv.ParseInt
function.
Using strconv.ParseInt
package main import ( "fmt" "strconv" ) func main() { str := "1234567890" num, err := strconv.ParseInt(str, 10, 64) // Parse as base 10 and store as int64 if err != nil { fmt.Println("Error:", err) return } fmt.Println(num) // Output: 1234567890 }
Handling Conversion Errors
If the input string is not a valid number, strconv.ParseInt
will return an error.
str := "invalid123" num, err := strconv.ParseInt(str, 10, 64) if err != nil { fmt.Println("Conversion failed:", err) } else { fmt.Println(num) }
Which Method Should You Use?
Method | Conversion Direction | Performance | Use Case |
---|---|---|---|
strconv.FormatInt | int64 → string | Fast | Recommended for simple conversions |
fmt.Sprintf | int64 → string | Slightly slower | Useful when formatting text with numbers |
strconv.ParseInt | string → int64 | Fast | Best for parsing numeric strings |
fmt.Sscanf (not covered) | string → int64 | Slower | Useful when parsing formatted strings |
Conclusion
In Golang, you can easily convert between int64
and string
using the strconv
package or fmt.Sprintf
. For performance-critical applications, strconv.FormatInt
and strconv.ParseInt
are preferred. If you need more flexibility, fmt.Sprintf
works well.
By understanding these conversion methods, you can efficiently handle numeric data in your Go applications.
FAQs
strconv.FormatInt(num, 10)
is the most efficient method.
Use strconv.ParseInt
and check the error return value.
Use fmt.Sprintf
when formatting numbers within a larger text string.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ