Mastering Rust’s Result Enum for Error Handling
James Reed
Infrastructure Engineer · Leapcell
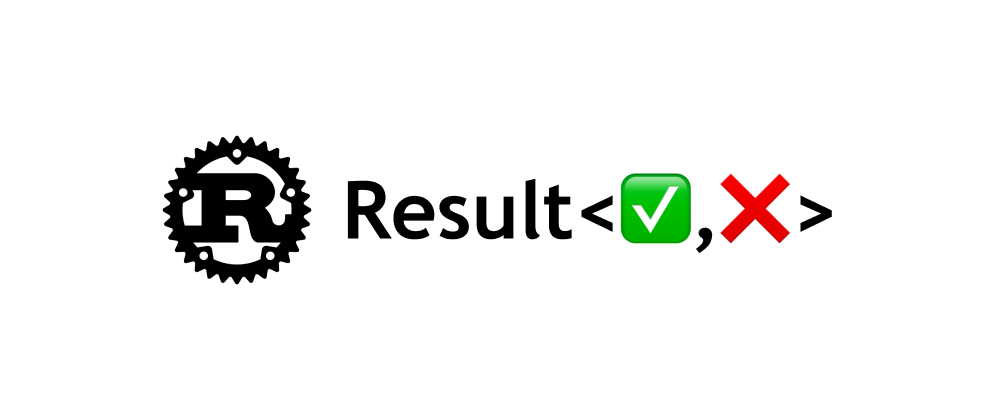
The Result
Type in Rust
Rust is a systems programming language that provides a unique error-handling mechanism. In Rust, errors are categorized into two types: recoverable errors and unrecoverable errors. For recoverable errors, Rust provides the Result
type to handle them.
Definition of the Result
Type
The Result
type is an enumeration with two variants: Ok
and Err
. The Ok
variant represents a successful operation and contains a success value, whereas the Err
variant represents a failed operation and contains an error value.
Below is the definition of the Result
type:
enum Result<T, E> { Ok(T), Err(E), }
Here, T
represents the type of the success value, and E
represents the type of the error value.
Uses of the Result
Type
The Result
type is commonly used as a function return value. When a function executes successfully, it returns an Ok
variant; when it fails, it returns an Err
variant.
Below is a simple example:
fn divide(numerator: f64, denominator: f64) -> Result<f64, String> { if denominator == 0.0 { Err("Cannot divide by zero".to_string()) } else { Ok(numerator / denominator) } } fn main() { let result = divide(4.0, 2.0); match result { Ok(value) => println!("Result: {}", value), Err(e) => println!("Error: {}", e), } }
In this example, the divide
function takes two arguments: a numerator and a denominator. If the denominator is 0
, it returns the Err
variant; otherwise, it returns the Ok
variant.
In the main
function, we call the divide
function and use a match
statement to handle the return value. If the return value is Ok
, the result is printed; if it is Err
, the error message is printed.
How to Handle Errors with Result
When calling a function that returns a Result
type, we need to handle potential errors. There are several ways to do this:
Using the match
Statement
The match
statement is the most common way to handle Result
type errors in Rust. It allows us to execute different operations based on the return value.
Here’s a simple example:
fn divide(numerator: f64, denominator: f64) -> Result<f64, String> { if denominator == 0.0 { Err("Cannot divide by zero".to_string()) } else { Ok(numerator / denominator) } } fn main() { let result = divide(4.0, 2.0); match result { Ok(value) => println!("Result: {}", value), Err(e) => println!("Error: {}", e), } }
In this example, we use a match
statement to handle the return value of the divide
function. If it returns Ok
, the result is printed; if it returns Err
, the error message is printed.
Using the if let
Statement
The if let
statement is a simplified version of match
. It can match only one case and does not require handling other cases. The if let
statement is often used when we only care about one case of the Result
type.
Here’s a simple example:
fn divide(numerator: f64, denominator: f64) -> Result<f64, String> { if denominator == 0.0 { Err("Cannot divide by zero".to_string()) } else { Ok(numerator / denominator) } } fn main() { let result = divide(4.0, 2.0); if let Ok(value) = result { println!("Result: {}", value); } }
In this example, we use the if let
statement to handle the return value of the divide
function. If it returns Ok
, the result is printed; otherwise, nothing happens.
Using the ?
Operator
The ?
operator is a special syntax in Rust that allows errors to be conveniently propagated from within a function. When calling a function that returns a Result
type, the ?
operator can simplify error handling.
Here’s a simple example:
fn divide(numerator: f64, denominator: f64) -> Result<f64, String> { if denominator == 0.0 { Err("Cannot divide by zero".to_string()) } else { Ok(numerator / denominator) } } fn calculate(numerator: f64, denominator: f64) -> Result<f64, String> { let result = divide(numerator, denominator)?; Ok(result * 2.0) } fn main() { let result = calculate(4.0, 2.0); match result { Ok(value) => println!("Result: {}", value), Err(e) => println!("Error: {}", e), } }
In this example, the calculate
function calls the divide
function internally and uses the ?
operator to simplify error handling. If divide
returns Err
, calculate
will immediately return Err
; otherwise, execution continues.
Common Methods of Result
The Result
type provides several useful methods that make error handling more convenient.
is_ok
and is_err
Methods
The is_ok
and is_err
methods check whether a Result
is an Ok
or Err
variant, respectively.
Here’s a simple example:
fn divide(numerator: f64, denominator: f64) -> Result<f64, String> { if denominator == 0.0 { Err("Cannot divide by zero".to_string()) } else { Ok(numerator / denominator) } } fn main() { let result = divide(4.0, 2.0); if result.is_ok() { println!("Result: {}", result.unwrap()); } else { println!("Error: {}", result.unwrap_err()); } }
In this example, we use the is_ok
method to check whether the return value of divide
is Ok
. If so, we use unwrap
to get the success value and print it; otherwise, we use unwrap_err
to get the error message and print it.
unwrap
and unwrap_err
Methods
The unwrap
and unwrap_err
methods retrieve the success or error value from a Result
, respectively. If the Result
is not of the expected variant, a panic occurs.
Here’s a simple example:
fn divide(numerator: f64, denominator: f64) -> Result<f64, String> { if denominator == 0.0 { Err("Cannot divide by zero".to_string()) } else { Ok(numerator / denominator) } } fn main() { let result = divide(4.0, 2.0); let value = result.unwrap(); println!("Result: {}", value); }
In this example, we use unwrap
to get the success value of the divide
function. If the return value is not Ok
, a panic will occur.
expect
and expect_err
Methods
The expect
and expect_err
methods are similar to unwrap
and unwrap_err
, but they allow a custom error message to be specified. If the Result
is not of the expected variant, a panic occurs and the specified message is printed.
Here’s a simple example:
fn divide(numerator: f64, denominator: f64) -> Result<f64, String> { if denominator == 0.0 { Err("Cannot divide by zero".to_string()) } else { Ok(numerator / denominator) } } fn main() { let result = divide(4.0, 2.0); let value = result.expect("Failed to divide"); println!("Result: {}", value); }
In this example, we use expect
to retrieve the success value of the divide
function. If the return value is not Ok
, a panic occurs and the specified error message is printed.
Features and Advantages of Result
The Result
type has the following features and advantages:
- Explicit error handling: The
Result
type forces programmers to explicitly handle errors, preventing them from being ignored or overlooked. - Type safety: The
Result
type is a generic type that can hold any type of success or error value, ensuring type safety and preventing type conversion errors. - Convenient error propagation: Rust provides the
?
operator to easily propagate errors from a function. - Easy composition: The
Result
type provides various composition methods, such asand
,or
,and_then
, andor_else
, making it easier to combine multipleResult
values.
Using Result
in Real-World Code
In real-world code, we often define a custom error type and use the Result
type to return error information.
Here’s a simple example:
use std::num::ParseIntError; type Result<T> = std::result::Result<T, MyError>; #[derive(Debug)] enum MyError { DivideByZero, ParseIntError(ParseIntError), } impl From<ParseIntError> for MyError { fn from(e: ParseIntError) -> Self { MyError::ParseIntError(e) } } fn divide(numerator: &str, denominator: &str) -> Result<f64> { let numerator: f64 = numerator.parse()?; let denominator: f64 = denominator.parse()?; if denominator == 0.0 { Err(MyError::DivideByZero) } else { Ok(numerator / denominator) } } fn main() { let result = divide("4", "2"); match result { Ok(value) => println!("Result: {}", value), Err(e) => println!("Error: {:?}", e), } }
In this example:
- We define a custom error type
MyError
, which includes two variants:DivideByZero
andParseIntError
. - We define a type alias
Result
, settingMyError
as the error type. - The
divide
function takes two string arguments and attempts to parse them intof64
. If parsing fails, the?
operator propagates the error. If the denominator is0
, anErr
variant is returned; otherwise, the function returnsOk
.
In the main
function, we call divide
and use a match
statement to handle the return value. If it returns Ok
, we print the result; if it returns Err
, we print the error message.
Handling File Read/Write Errors with Result
When working with file operations, various errors can occur, such as file not found or insufficient permissions. These errors can be handled using the Result
type.
Here’s a simple example:
use std::fs; use std::io; fn read_file(path: &str) -> Result<String, io::Error> { fs::read_to_string(path) } fn main() { let result = read_file("test.txt"); match result { Ok(content) => println!("File content: {}", content), Err(e) => println!("Error: {}", e), } }
In this example:
- The
read_file
function takes a file path as an argument and usesfs::read_to_string
to read the file content. fs::read_to_string
returns aResult
type with a success value containing the file content and an error value of typeio::Error
.- In
main
, we callread_file
and usematch
to handle the return value. If it returnsOk
, the file content is printed; if it returnsErr
, the error message is printed.
Handling Network Request Errors with Result
When making network requests, various errors can occur, such as connection timeouts or server errors. These errors can also be handled using the Result
type.
Here’s a simple example:
use std::io; use std::net::TcpStream; fn connect(host: &str) -> Result<TcpStream, io::Error> { TcpStream::connect(host) } fn main() { let result = connect("example.com:80"); match result { Ok(stream) => println!("Connected to {}", stream.peer_addr().unwrap()), Err(e) => println!("Error: {}", e), } }
In this example:
- The
connect
function takes a host address as an argument and usesTcpStream::connect
to establish a TCP connection. TcpStream::connect
returns aResult
type with a success value of typeTcpStream
and an error value of typeio::Error
.- In
main
, we callconnect
and usematch
to handle the return value. If it returnsOk
, the connection information is printed; if it returnsErr
, the error message is printed.
Best Practices for Result
and Error Handling
When handling errors with Result
, the following best practices can help write better code:
- Define a custom error type: A custom error type helps organize and manage error information more effectively.
- Use the
?
operator to propagate errors: The?
operator makes it easy to propagate errors from a function. - Avoid excessive use of
unwrap
andexpect
: These methods cause a panic if anErr
variant is encountered. Instead, handle errors properly usingmatch
orif let
. - Use composition methods to combine multiple
Result
values: Methods likeand
,or
,and_then
, andor_else
help combine multipleResult
values efficiently.
We are Leapcell, your top choice for hosting Rust projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ