Understanding `json.dumps()` in Python
Grace Collins
Solutions Engineer · Leapcell
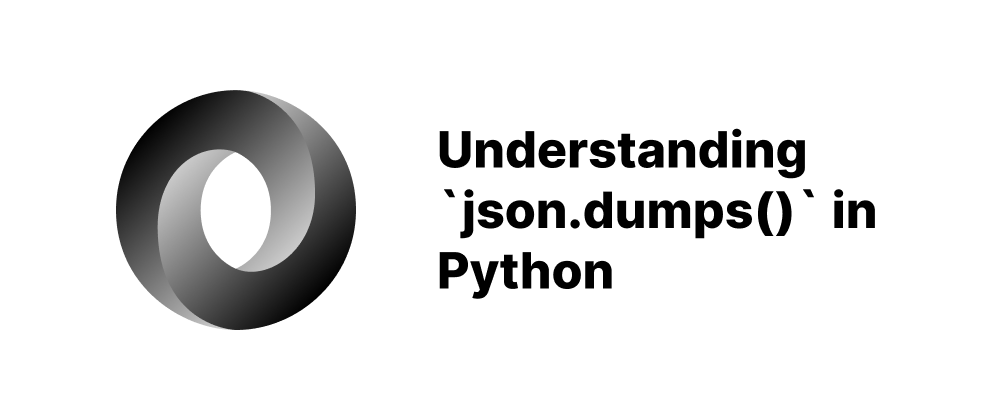
Key Takeaways
json.dumps()
converts Python objects into JSON-formatted strings.- You can customize output with parameters like
indent
,sort_keys
, andensure_ascii
. - Non-serializable objects require a custom
default
function for conversion.
Working with JSON (JavaScript Object Notation) data is a common task in Python development, particularly in web development, data interchange, and APIs. Python’s built-in json
module provides various methods to handle JSON data. One of the most frequently used functions is json.dumps()
. This article explains what json.dumps()
does, how to use it, and some useful parameters that can help customize the output.
What is json.dumps()
?
The json.dumps()
function in Python is used to convert a Python object into a JSON-formatted string. The “s” in dumps
stands for “dump string.” Unlike json.dump()
which writes JSON data directly to a file, dumps()
returns the JSON data as a string, making it useful when you need to store or transmit JSON data.
Basic Usage
Here’s a simple example of how to use json.dumps()
:
import json data = { "name": "Alice", "age": 30, "is_student": False } json_string = json.dumps(data) print(json_string)
Output:
{"name": "Alice", "age": 30, "is_student": false}
Notice how the Python False
is converted to JSON’s false
, and keys are wrapped in double quotes.
Common Parameters
json.dumps()
accepts several optional parameters that allow you to customize the output:
1. indent
Adds indentation to make the JSON string more readable:
json_string = json.dumps(data, indent=4) print(json_string)
Output:
{ "name": "Alice", "age": 30, "is_student": false }
2. sort_keys
Sorts the keys alphabetically in the output:
json_string = json.dumps(data, sort_keys=True) print(json_string)
Output:
{"age": 30, "is_student": false, "name": "Alice"}
3. separators
Controls item and key-value separators. The default is (", ", ": ")
, but you can make it more compact:
json_string = json.dumps(data, separators=(",", ":")) print(json_string)
Output:
{"name":"Alice","age":30,"is_student":false}
4. ensure_ascii
By default, all non-ASCII characters are escaped. To allow Unicode characters to be output as-is:
data = {"name": "Alice"} json_string = json.dumps(data, ensure_ascii=False) print(json_string)
Output:
{"name": "Alice"}
Handling Non-Serializable Objects
Some Python objects (like sets, custom classes, datetime objects) aren’t serializable by default. You can handle them using the default
parameter:
from datetime import datetime def serialize_obj(obj): if isinstance(obj, datetime): return obj.isoformat() raise TypeError("Type not serializable") data = {"created": datetime.now()} json_string = json.dumps(data, default=serialize_obj) print(json_string)
Conclusion
The json.dumps()
function is a powerful tool in Python for converting complex data structures into JSON-formatted strings. Whether you're logging data, storing it, or sending it across networks, understanding how to customize json.dumps()
gives you flexibility and control over your data representation.
FAQs
json.dump()
writes to a file; json.dumps()
returns a JSON string.
Use the indent
parameter to format the JSON string with line breaks and spaces.
Not by default. Use the default
parameter to define how such objects should be serialized.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ