How to Use `json.MarshalIndent` in Golang
Grace Collins
Solutions Engineer · Leapcell
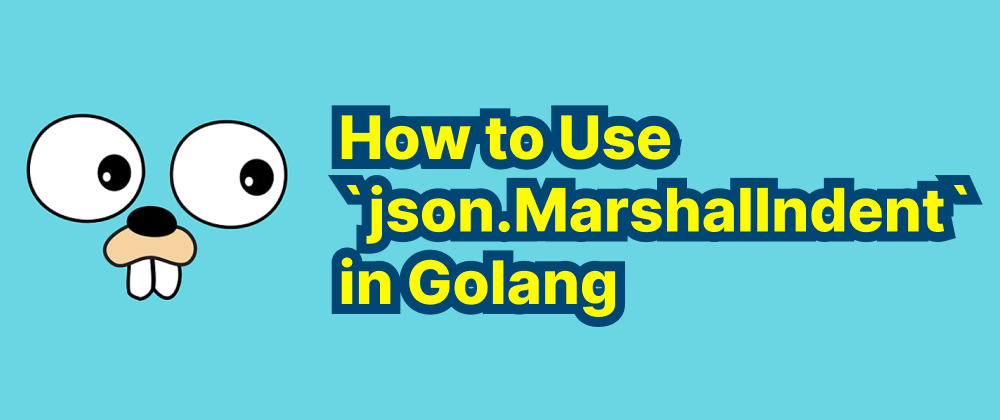
Key Takeaways
json.MarshalIndent
formats JSON with customizable indentation and prefixes.- It improves JSON readability, making it useful for debugging and logging.
- Proper error handling is necessary to avoid issues with unsupported data types.
Introduction
When working with JSON in Golang, the encoding/json
package provides the json.Marshal
function to serialize Go data structures into JSON format. However, the default output of json.Marshal
is compact, meaning it does not include any extra whitespace or indentation. If you need a human-readable, formatted JSON output, you can use json.MarshalIndent
.
This article explains how to use json.MarshalIndent
effectively and provides practical examples.
Understanding json.MarshalIndent
The json.MarshalIndent
function is similar to json.Marshal
, but it allows you to specify indentation settings. Its function signature is as follows:
func MarshalIndent(v interface{}, prefix, indent string) ([]byte, error)
v
is the Go value to be serialized into JSON.prefix
is a string that will be prefixed to each line of the output.indent
defines the indentation characters used for formatting.- The function returns a
[]byte
containing the formatted JSON and anerror
if serialization fails.
Basic Example
Let’s look at a simple example using json.MarshalIndent
:
package main import ( "encoding/json" "fmt" ) type User struct { Name string `json:"name"` Age int `json:"age"` Email string `json:"email"` } func main() { user := User{ Name: "Alice", Age: 25, Email: "alice@example.com", } // MarshalIndent with two spaces for indentation jsonData, err := json.MarshalIndent(user, "", " ") if err != nil { fmt.Println("Error:", err) return } // Convert JSON bytes to string and print fmt.Println(string(jsonData)) }
Output
{ "name": "Alice", "age": 25, "email": "alice@example.com" }
Explanation
- The second argument (
""
) is an empty prefix, meaning there will be no extra characters at the start of each line. - The third argument (
" "
) specifies that each indentation level uses two spaces. - The output JSON is properly formatted with newlines and indentation, making it easier to read.
Customizing Indentation
You can customize the indent
parameter to use different formatting styles.
Using Tabs Instead of Spaces
jsonData, err := json.MarshalIndent(user, "", "\t")
This will produce JSON with tab indentation instead of spaces.
Adding a Prefix
jsonData, err := json.MarshalIndent(user, "> ", " ")
This adds a "> "
prefix before each line:
> { > "name": "Alice", > "age": 25, > "email": "alice@example.com" > }
Handling Nested Structures
json.MarshalIndent
works well with nested structures. Here’s an example with an embedded struct:
type Address struct { City string `json:"city"` State string `json:"state"` } type UserWithAddress struct { Name string `json:"name"` Age int `json:"age"` Email string `json:"email"` Address Address `json:"address"` } func main() { user := UserWithAddress{ Name: "Bob", Age: 30, Email: "bob@example.com", Address: Address{ City: "New York", State: "NY", }, } jsonData, err := json.MarshalIndent(user, "", " ") if err != nil { fmt.Println("Error:", err) return } fmt.Println(string(jsonData)) }
Output
{ "name": "Bob", "age": 30, "email": "bob@example.com", "address": { "city": "New York", "state": "NY" } }
Error Handling
If json.MarshalIndent
encounters an unsupported type (such as a channel or function), it will return an error. Always check for errors before using the returned JSON data:
jsonData, err := json.MarshalIndent(user, "", " ") if err != nil { fmt.Println("Error:", err) return } fmt.Println(string(jsonData))
Conclusion
json.MarshalIndent
is a useful function in Golang for generating human-readable JSON with indentation. By specifying a prefix and an indent string, you can customize the output format to suit your needs. It is particularly useful when logging JSON responses, debugging, or storing configuration files in a readable format.
By mastering json.MarshalIndent
, you can improve the readability of your JSON data and make it easier to work with in development and debugging scenarios.
FAQs
json.Marshal
produces compact JSON, while json.MarshalIndent
formats JSON with indentation for better readability.
Yes, by passing "\t"
as the indent parameter in json.MarshalIndent
.
json.MarshalIndent
will return an error, so you should always handle errors properly.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ