Understanding Getters in Go
James Reed
Infrastructure Engineer · Leapcell
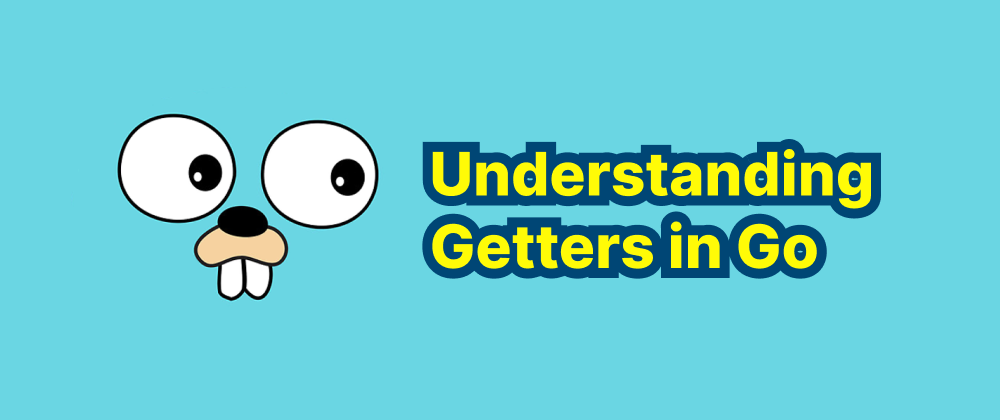
Key Takeaways
- Go prefers direct field access over traditional getter methods.
- Getters are useful when validation, computation, or encapsulation is required.
- Idiomatic Go avoids the
Get
prefix, using method names that match field names.
In Go programming, the use of getters—methods that retrieve the value of a field—differs from practices in some other programming languages. This article explores how Go handles getters, emphasizing its idiomatic approach and the rationale behind it.
Go's Approach to Getters
In languages like Java or C#, it's common to define explicit getter methods to access private fields:
private int count; public int getCount() { return count; }
This pattern encapsulates the field, allowing controlled access. However, Go encourages a more straightforward approach.
Exported Fields
Go's visibility rules are based on capitalization. An identifier (variable, type, function name, etc.) is exported from a package if it begins with an uppercase letter. Thus, to make a struct field accessible from other packages, you capitalize its name:
package counter type Counter struct { Count int // Exported field }
With this design, external packages can directly access and modify the Count
field:
package main import ( "fmt" "counter" ) func main() { c := counter.Counter{Count: 10} fmt.Println(c.Count) // Direct access }
When to Use Getters
While direct field access is idiomatic in Go, there are scenarios where getters (and setters) are appropriate:
-
Validation or Computation: If retrieving a field's value requires validation or computation, a method is suitable.
-
Interface Implementation: To satisfy an interface that demands specific method signatures.
-
Unexported Fields: When a field shouldn't be modified directly by external packages, you can provide controlled access through methods.
Defining Getters in Go
When defining a getter in Go, it's customary to name the method after the field itself, avoiding the Get
prefix:
package counter type Counter struct { count int // Unexported field } // Method to retrieve 'count' func (c *Counter) Count() int { return c.count }
This convention aligns with Go's emphasis on simplicity and readability.
Conclusion
Go's design philosophy promotes straightforward and readable code. Direct access to exported fields is preferred, reducing boilerplate code associated with traditional getter methods. However, when additional control or functionality is necessary, defining methods without the Get
prefix is the idiomatic approach. Understanding these conventions ensures that Go code remains clean, efficient, and in harmony with the language's core principles.
FAQs
Go favors simplicity and direct field access for readability and efficiency.
Use getters when additional logic, validation, or encapsulation is needed.
Go conventions prioritize clarity; methods are named after the field itself.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ