Simulating Tuples in Go: Structs, Generics, and Practical Workarounds
James Reed
Infrastructure Engineer · Leapcell
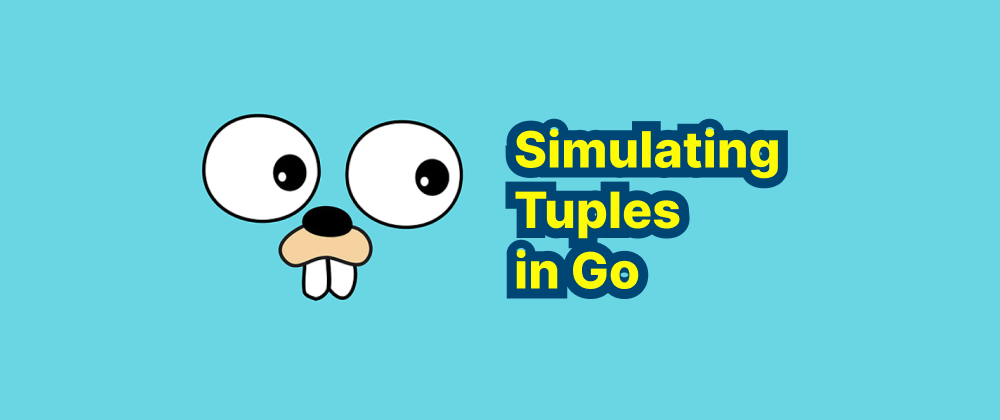
Key Takeaways
- Go does not have built-in tuple types, but multiple workarounds exist.
- Structs (including generics) are the most type-safe way to simulate tuples.
- Third-party libraries offer tuple utilities, especially for functional programming use cases.
Introduction
In programming, tuples are ordered collections of elements that can be of varying types. Languages like Python and Haskell offer native support for tuples, allowing developers to group multiple values without defining a formal structure. However, Go (Golang) takes a different approach. While Go supports multiple return values from functions, it doesn't have a built-in tuple type as a first-class citizen in its type system.
Simulating Tuples in Go
Despite the absence of native tuple support, Go developers have devised several methods to emulate tuple-like behavior:
1. Using Structs
The most straightforward way to group multiple values is by defining a struct
.
type Pair struct { First int Second string }
This method provides clarity and type safety but requires defining a new type for each combination of grouped values.
2. Anonymous Structs
For quick, one-off groupings, anonymous structs can be used:
pair := struct { First int Second string }{First: 1, Second: "two"}
While this avoids the need to define a new type, it can become cumbersome and less readable for complex structures.
3. Slices of interface{}
Another approach is using slices of empty interfaces:
tuple := []interface{}{1, "two", 3.0}
This method offers flexibility but sacrifices type safety, leading to potential runtime errors if not handled carefully.
Leveraging Generics for Tuples
With the introduction of generics in Go 1.18, developers can now create more versatile and type-safe tuple structures.
type Pair[T, U any] struct { First T Second U }
This generic Pair
struct allows for the grouping of any two types without sacrificing type safety.
Third-Party Tuple Libraries
Several libraries have emerged to provide tuple functionalities in Go:
1. go-tuple
by Bar Weiss
This library offers tuple implementations ranging from 1 to 9 elements. It provides methods for creating tuples, accessing their values, and even JSON marshalling.
import "github.com/barweiss/go-tuple" tup := tuple.New2(5, "hi!") fmt.Println(tup.V1) // Outputs: 5 fmt.Println(tup.V2) // Outputs: hi!
The library also supports unpacking tuples into variables and using them in various data structures like maps and channels.
2. go-fp/tuple
by Justin Knueppel
This package focuses on functional programming paradigms, providing an immutable Pair
type with helper functions like Fst
and Snd
to access elements.
import "github.com/JustinKnueppel/go-fp/tuple" p := tuple.NewPair(1, "hello") fmt.Println(tuple.Fst(p)) // Outputs: 1 fmt.Println(tuple.Snd(p)) // Outputs: hello
It's particularly useful for scenarios where immutability and functional patterns are preferred.
Future of Tuples in Go
There have been discussions and proposals about introducing native tuple types in Go. One such proposal suggests syntax like (int, string)
to define tuple types and using (a, b)
for tuple values. However, the Go team has expressed concerns about the added complexity and potential redundancy, given that structs already offer similar functionalities.
Conclusion
While Go doesn't natively support tuples as first-class types, developers have several tools and patterns at their disposal to emulate tuple behavior. With the advent of generics and third-party libraries, creating and managing tuple-like structures has become more accessible and type-safe. As the language evolves, it's possible that more native support for tuples may be introduced, but for now, these methods provide effective solutions.
FAQs
No. Go lacks native tuple support but allows multiple return values and other constructs to simulate them.
Use a struct—either named or anonymous. With generics, you can also define reusable typed pairs.
Yes. Libraries like go-tuple
and go-fp/tuple
are actively used and provide reliable APIs for tuple-like behavior.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ