16 Advanced Django Questions You Should Know
Grace Collins
Solutions Engineer ยท Leapcell
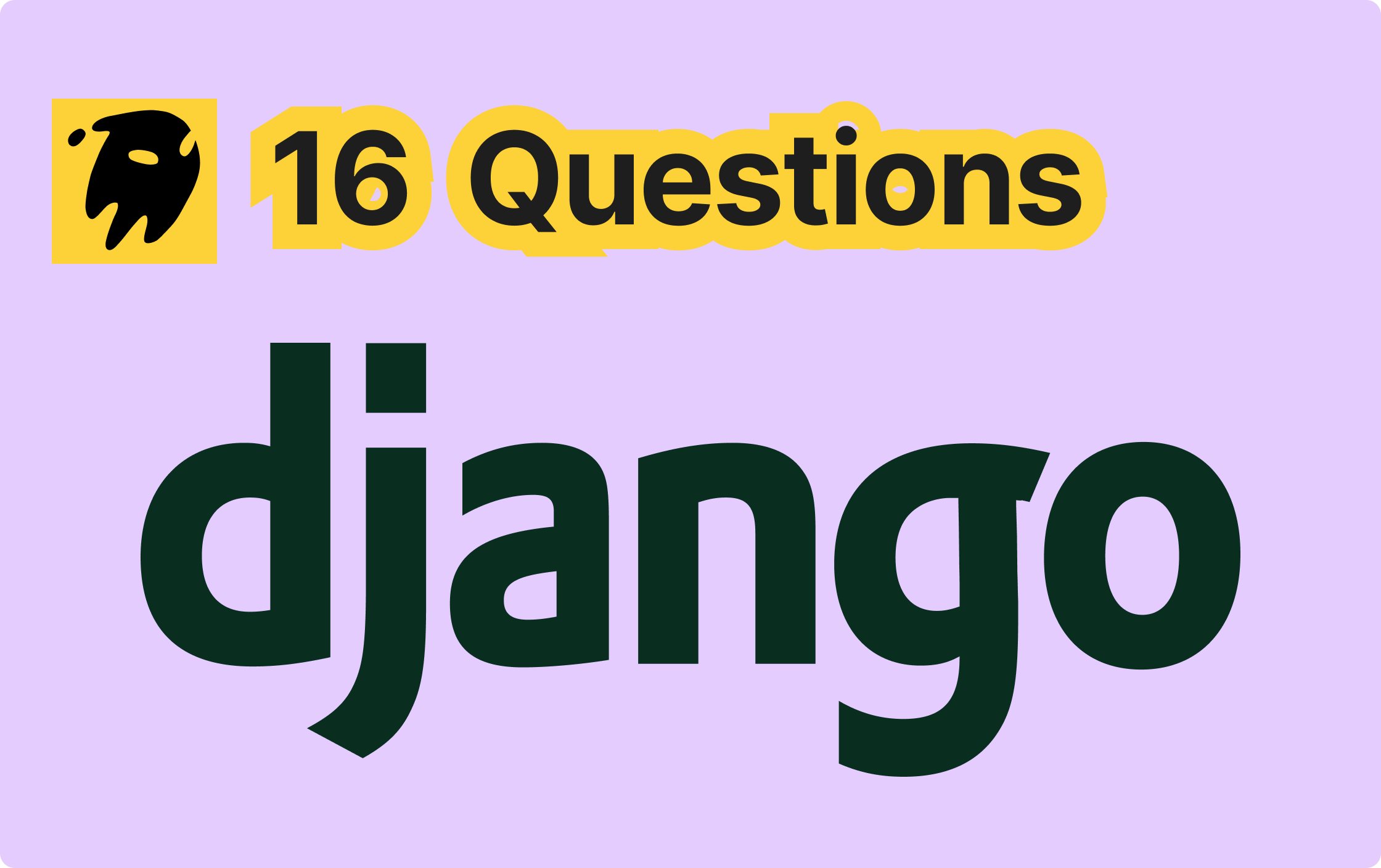
16 Common Advanced Django Questions
When moving from beginner to advanced Django development, developers often encounter complex and challenging issues. This article compiles 16 common questions in advanced Django development and provides answers with rich examples to help developers master advanced Django development techniques.
1. How to Optimize Django Model Query Performance?
Database query performance is critical in Django projects. Improper queries can cause performance bottlenecks when data volumes are large.
- Using
select_related
andprefetch_related
select_related
handlesForeignKey
andOneToOneField
relationships by reducing database query times through SQLJOIN
operations.prefetch_related
handlesManyToManyField
and reverseForeignKey
relationships by performing additional queries and merging results at the Python level.
from django.db import models class Author(models.Model): name = models.CharField(max_length=100) class Book(models.Model): title = models.CharField(max_length=200) author = models.ForeignKey(Author, on_delete=models.CASCADE) # Optimize queries with select_related books = Book.objects.select_related('author').all() for book in books: print(book.title, book.author.name) class Tag(models.Model): name = models.CharField(max_length=50) books = models.ManyToManyField(Book) # Optimize queries with prefetch_related tags = Tag.objects.prefetch_related('books').all() for tag in tags: for book in tag.books.all(): print(tag.name, book.title)
- Rational Use of Indexes
Adding indexes to model fields can speed up queries. Indexes can be added usingdb_index=True
in field definitions.
class Product(models.Model): name = models.CharField(max_length=100, db_index=True) price = models.DecimalField(max_digits=8, decimal_places=2)
2. How to Implement Asynchronous Views in Django?
Django has supported asynchronous views since version 3.1, which effectively improves processing efficiency for I/O-bound tasks.
import asyncio from django.http import JsonResponse from django.views.decorators.http import async_only @async_only async def async_view(request): await asyncio.sleep(2) # Simulate asynchronous operations data = {'message': 'This is an async view'} return JsonResponse(data)
Additionally, configure an ASGI server (e.g., uvicorn
) that supports asynchrony in settings.py
.
3. How to Manage Database Transactions in Django?
Database transactions ensure that a series of database operations either all succeed or all fail.
from django.db import transaction from.models import Account @transaction.atomic def transfer_funds(sender, receiver, amount): with transaction.atomic(): sender.balance -= amount receiver.balance += amount sender.save() receiver.save()
In the code above, the @transaction.atomic
decorator ensures that operations within the transfer_funds
function are completed within a single transaction. If any save
operation fails, the entire transaction rolls back.
4. How to Configure Caching in Django?
Django provides multiple caching methods, such as in-memory caching, file caching, and database caching.
- Cache Configuration
Configure caching insettings.py
(example using in-memory caching):
CACHES = { 'default': { 'BACKEND': 'django.core.cache.backends.memcached.PyMemcacheCache', 'LOCATION': '127.0.0.1:11211', } }
- Using Caching
from django.core.cache import cache def get_cached_data(key): data = cache.get(key) if data is None: data = calculate_data() # Assume this is a data retrieval function cache.set(key, data, 60) # Cache for 60 seconds return data
5. How to Customize Django's User Authentication System?
Django's built-in user authentication system may not always meet project requirements, necessitating customization.
from django.contrib.auth.models import AbstractBaseUser, BaseUserManager from django.db import models class CustomUserManager(BaseUserManager): def create_user(self, email, password=None): if not email: raise ValueError('Users must have an email address') user = self.model( email=self.normalize_email(email), ) user.set_password(password) user.save(using=self._db) return user def create_superuser(self, email, password): user = self.create_user( email=email, password=password, ) user.is_admin = True user.save(using=self._db) return user class CustomUser(AbstractBaseUser): email = models.EmailField( verbose_name='email address', max_length=255, unique=True, ) is_active = models.BooleanField(default=True) is_admin = models.BooleanField(default=False) objects = CustomUserManager() USERNAME_FIELD = 'email' REQUIRED_FIELDS = [] def __str__(self): return self.email def has_perm(self, perm, obj=None): return True def has_module_perms(self, app_label): return True @property def is_staff(self): return self.is_admin
Then set AUTH_USER_MODEL = 'your_app_name.CustomUser'
in settings.py
.
6. How to Handle Cross-Origin Requests in Django?
Cross-origin issues occur when the frontend and Django backend are on different domains. The django-cors-headers
library can resolve this.
- Install the library:
pip install django-cors-headers
- Configure in
settings.py
:
INSTALLED_APPS = [ ... 'corsheaders', ... ] MIDDLEWARE = [ ... 'corsheaders.middleware.CorsMiddleware', 'django.middleware.common.CommonMiddleware', ... ] CORS_ALLOWED_ORIGINS = [ "https://example.com", ]
7. How to Use Signals in Django?
Django signals allow executing custom code when specific events occur.
from django.db.models.signals import post_save from django.dispatch import receiver from.models import Order, OrderNotification @receiver(post_save, sender=Order) def create_order_notification(sender, instance, created, **kwargs): if created: OrderNotification.objects.create(order=instance, message='New order created')
The code above creates a corresponding OrderNotification
after saving the Order
model if it is a new order.
8. How to Implement File Uploads in Django?
Implementing file uploads requires corresponding settings in models, views, and templates.
- Model Definition
from django.db import models class UploadedFile(models.Model): file = models.FileField(upload_to='uploads/') uploaded_at = models.DateTimeField(auto_now_add=True)
- View Handling
from django.shortcuts import render, redirect from.forms import UploadFileForm def upload_file(request): if request.method == 'POST': form = UploadFileForm(request.POST, request.FILES) if form.is_valid(): form.save() return redirect('file_list') else: form = UploadFileForm() return render(request, 'upload.html', {'form': form})
- Template Code
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> </head> <body> <form method="post" enctype="multipart/form-data"> {% csrf_token %} {{ form.as_p }} <input type="submit" value="Upload"> </form> </body> </html>
9. How to Write Unit Tests in Django?
Django provides a testing framework for writing unit tests.
from django.test import TestCase from.models import Book class BookModelTest(TestCase): @classmethod def setUpTestData(cls): Book.objects.create(title='Test Book', author='Test Author') def test_book_title(self): book = Book.objects.get(id=1) self.assertEqual(book.title, 'Test Book')
Run tests using the python manage.py test
command.
10. How to Implement a Task Queue in Django?
Celery
can be used to implement task queues in Django for handling asynchronous tasks.
- Install
Celery
:pip install celery
- Create a
celery.py
file in the project root directory:
import os from celery import Celery os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'your_project.settings') app = Celery('your_project') app.config_from_object('django.conf:settings', namespace='CELERY') app.autodiscover_tasks()
- Import the Celery app in
__init__.py
:
from.celery import app as celery_app __all__ = ('celery_app',)
- Define tasks:
from celery import shared_task @shared_task def send_email_task(to_email, subject, message): # Email sending logic pass
11. How to Implement Multilingual Support in Django?
Django has built-in multilingual support.
- Configure languages in
settings.py
:
LANGUAGE_CODE = 'en-us' USE_I18N = True USE_L10N = True LANGUAGES = [ ('en', 'English'), ('zh-hans', 'Simplified Chinese'), ] LOCALE_PATHS = [ os.path.join(BASE_DIR, 'locale'), ]
- Use translations in templates:
{% load i18n %} <!DOCTYPE html> <html lang="{{ LANGUAGE_CODE }}"> <head> <title>{% trans "My Page" %}</title> </head> <body> <p>{% trans "Welcome to my site" %}</p> </body> </html>
- Run
python manage.py makemessages -l zh-hans
to generate translation files, edit the translation content, and runpython manage.py compilemessages
to compile the translation files.
12. How to Set Up Logging in Django?
Reasonable logging helps troubleshoot issues.
Configure logging in settings.py
:
LOGGING = { 'version': 1, 'disable_existing_loggers': False, 'handlers': { 'console': { 'class': 'logging.StreamHandler', }, }, 'root': { 'handlers': ['console'], 'level': 'DEBUG', }, }
Use logging in code:
import logging logger = logging.getLogger(__name__) def my_view(request): try: # Business logic pass except Exception as e: logger.error(f"An error occurred: {e}", exc_info=True)
13. How to Implement API Versioning in Django?
API versioning is necessary as APIs evolve. This can be achieved using libraries like drf-nested-routers
with Django REST framework
.
from rest_framework.routers import DefaultRouter from.views import v1, v2 router_v1 = DefaultRouter() router_v1.register('users', v1.UserViewSet) router_v2 = DefaultRouter() router_v2.register('users', v2.UserViewSet) urlpatterns = [ path('api/v1/', include(router_v1.urls)), path('api/v2/', include(router_v2.urls)), ]
14. How to Strengthen Django Security?
To prevent security vulnerabilities, Django projects need security hardening.
- Set security-related
settings.py
parameters
SECRET_KEY = 'your_secret_key' DEBUG = False SECURE_SSL_REDIRECT = True SESSION_COOKIE_SECURE = True CSRF_COOKIE_SECURE = True X_FRAME_OPTIONS = 'DENY'
- Prevent SQL Injection
Use Django's ORM for queries to avoid direct SQL string concatenation. - Prevent Cross-Site Scripting (XSS)
Django templates escape variables by default to prevent malicious script execution.
15. How to Use WebSockets in Django?
The channels
library enables WebSocket functionality in Django.
- Install
channels
:pip install channels
- Configure in
settings.py
:
ASGI_APPLICATION = 'your_project.asgi.application' CHANNEL_LAYERS = { 'default': { 'BACKEND': 'channels_redis.core.RedisChannelLayer', 'CONFIG': { "hosts": [('127.0.0.1', 6379)], }, }, }
- Define a
consumer
to handle WebSocket connections:
from channels.generic.websocket import AsyncWebsocketConsumer import json class ChatConsumer(AsyncWebsocketConsumer): async def connect(self): await self.accept() async def receive(self, text_data): text_data_json = json.loads(text_data) message = text_data_json['message'] await self.send(text_data=json.dumps({'message': message})) async def disconnect(self, close_code): pass
16. How to Analyze Code Performance in Django?
The django-debug-toolbar
library facilitates code performance analysis.
- Install the library:
pip install django-debug-toolbar
- Configure in
settings.py
:
INSTALLED_APPS = [ ... 'debug_toolbar', ... ] MIDDLEWARE = [ ... 'debug_toolbar.middleware.DebugToolbarMiddleware', ... ] INTERNAL_IPS = [ '127.0.0.1', ]
Once enabled, a debug toolbar will appear at the bottom of the page, displaying performance metrics such as the number of database queries and execution time.
Leapcell: The Best of Serverless Web Hosting
Finally, we recommend the best platform for deploying Python services: Leapcell
๐ Build with Your Favorite Language
Develop effortlessly in JavaScript, Python, Go, or Rust.
๐ Deploy Unlimited Projects for Free
Only pay for what you useโno requests, no charges.
โก Pay-as-You-Go, No Hidden Costs
No idle fees, just seamless scalability.
๐ Explore Our Documentation
๐น Follow us on Twitter: @LeapcellHQ