Simulating Default Parameters in Go
James Reed
Infrastructure Engineer · Leapcell
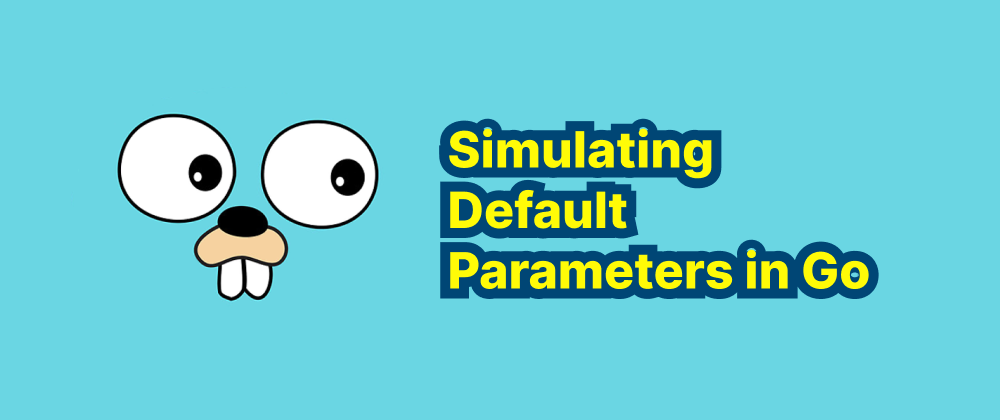
Key Takeaways
- Go does not natively support default parameters to maintain simplicity and explicitness.
- Wrapper functions, variadic arguments, and structs can simulate default values.
- The functional options pattern is a flexible way to handle optional configuration.
Unlike some programming languages like Python or JavaScript, Go does not support default parameters directly. This design decision aligns with Go’s simplicity and explicitness. However, there are idiomatic ways to simulate default parameter behavior when needed.
This article explores how to achieve default parameter functionality in Go using several common patterns.
Why Go Doesn't Support Default Parameters
Go emphasizes clarity and simplicity. Allowing default parameters would introduce implicit behavior, which the language designers intentionally avoided. Instead, Go developers are encouraged to be explicit with function signatures and use alternative patterns to simulate default values.
Common Workarounds for Default Parameters
1. Function Overloading via Wrapper Functions
While Go doesn't support true function overloading, you can write wrapper functions to simulate it:
func greetWithName(name string) { fmt.Println("Hello,", name) } func greet() { greetWithName("Guest") // default name }
This approach is simple and effective for one or two parameters.
2. Using Variadic Parameters
Variadic parameters allow a function to accept zero or more arguments of a certain type. You can use this to apply default values when no arguments are provided.
func greet(names ...string) { name := "Guest" if len(names) > 0 { name = names[0] } fmt.Println("Hello,", name) }
While this method works well for a single optional value, it becomes messy with multiple optional parameters.
3. Using Structs as Parameter Objects
A more scalable approach is to define a configuration struct and pass it to your function. This allows for multiple optional fields with clear defaults.
type Config struct { Name string Age int Debug bool } func runTask(cfg Config) { name := cfg.Name if name == "" { name = "Anonymous" } age := cfg.Age if age == 0 { age = 18 } fmt.Printf("Running task for %s (%d years old)\n", name, age) }
You can call it like this:
runTask(Config{Name: "Alice"}) // Age will default to 18
This is a widely used idiom in Go when dealing with complex configuration.
4. Functional Options Pattern
The functional options pattern is powerful and extensible. It uses functions to apply optional configurations to a struct.
type Server struct { Port int Host string } type Option func(*Server) func WithPort(port int) Option { return func(s *Server) { s.Port = port } } func WithHost(host string) Option { return func(s *Server) { s.Host = host } } func NewServer(opts ...Option) *Server { s := &Server{ Port: 8080, // default port Host: "localhost", } for _, opt := range opts { opt(s) } return s }
Usage:
s := NewServer(WithPort(9090)) fmt.Println(s.Host, s.Port) // localhost 9090
This pattern is common in Go libraries like grpc
, http.Server
, etc.
Conclusion
Go avoids implicit behaviors like default parameters in favor of explicitness and clarity. But by using idiomatic workarounds such as wrapper functions, variadic arguments, configuration structs, or the functional options pattern, you can effectively implement default parameter behavior where needed. Choose the approach that best suits the complexity of your use case.
FAQs
Go emphasizes clarity and avoids implicit behavior, which default parameters can introduce.
Using a struct or the functional options pattern is preferred for readability and scalability.
When only one optional parameter is needed and simplicity is prioritized.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ