Mastering Concurrency in Go with Goroutines and Channels
James Reed
Infrastructure Engineer · Leapcell
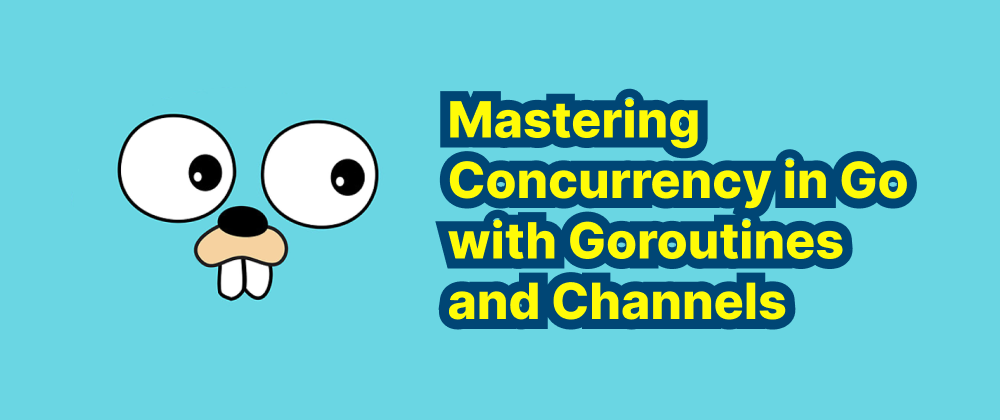
Key Takeaways
- Goroutines are lightweight threads that enable efficient concurrent execution in Go.
- Channels facilitate safe communication and synchronization between goroutines.
- Patterns like worker pools and pipelines help structure and manage concurrency effectively.
Go (often referred to as Golang) is renowned for its built-in support for concurrency, making it a powerful tool for developing efficient and scalable applications. Central to Go's concurrency model are goroutines and channels, which facilitate the execution of multiple tasks simultaneously and enable communication between them.
Goroutines: Lightweight Threads
A goroutine is a lightweight thread managed by the Go runtime. Unlike traditional threads, goroutines are more memory-efficient and are multiplexed onto a smaller number of OS threads, allowing for the creation of thousands of concurrent tasks without significant overhead.
To start a goroutine, simply prefix a function call with the go
keyword:
package main import ( "fmt" "time" ) func sayHello() { fmt.Println("Hello from goroutine") } func main() { go sayHello() time.Sleep(1 * time.Second) // Give the goroutine time to execute fmt.Println("Main function finished") }
In this example, sayHello
runs concurrently with the main
function. The time.Sleep
ensures that the program doesn't exit before the goroutine completes its execution.
Channels: Communication Between Goroutines
Channels in Go provide a way for goroutines to communicate and synchronize their execution. They are typed conduits through which you can send and receive values with the channel operator <-
.
Creating and Using Channels
Here's how you can create and use a channel:
package main import "fmt" func main() { messages := make(chan string) go func() { messages <- "Hello, Channel!" }() msg := <-messages fmt.Println(msg) }
In this code:
make(chan string)
creates a new channel of typestring
.- The anonymous goroutine sends a message into the channel.
- The main function receives the message from the channel and prints it.
Buffered vs. Unbuffered Channels
-
Unbuffered Channels: These channels block the sending goroutine until another goroutine receives the value from the channel. They are useful for synchronization.
-
Buffered Channels: These channels have a capacity and allow sending a limited number of values without a corresponding receive. They are useful when you want to decouple the timing between senders and receivers.
Example of a buffered channel:
package main import "fmt" func main() { messages := make(chan string, 2) messages <- "Buffered" messages <- "Channel" fmt.Println(<-messages) fmt.Println(<-messages) }
Concurrency Patterns in Go
Go's concurrency model enables the implementation of various concurrency patterns. Here are a few common ones:
Worker Pool Pattern
The worker pool pattern involves creating a fixed number of goroutines (workers) that process tasks from a shared channel. This pattern is useful for controlling the concurrency level and efficiently utilizing system resources.
package main import ( "fmt" "sync" ) func worker(id int, jobs <-chan int, results chan<- int, wg *sync.WaitGroup) { defer wg.Done() for j := range jobs { fmt.Printf("Worker %d processing job %d\n", id, j) results <- j * 2 } } func main() { const numJobs = 5 jobs := make(chan int, numJobs) results := make(chan int, numJobs) var wg sync.WaitGroup for w := 1; w <= 3; w++ { wg.Add(1) go worker(w, jobs, results, &wg) } for j := 1; j <= numJobs; j++ { jobs <- j } close(jobs) wg.Wait() close(results) for r := range results { fmt.Println("Result:", r) } }
In this example:
- Three workers process five jobs.
- The
sync.WaitGroup
ensures that the main function waits for all workers to finish before proceeding.
Pipeline Pattern
The pipeline pattern involves chaining a series of stages where each stage is a function that takes an input channel and returns an output channel. This pattern is useful for processing data through multiple steps concurrently.
package main import "fmt" func gen(nums ...int) <-chan int { out := make(chan int) go func() { for _, n := range nums { out <- n } close(out) }() return out } func sq(in <-chan int) <-chan int { out := make(chan int) go func() { for n := range in { out <- n * n } close(out) }() return out } func main() { c := gen(2, 3, 4) out := sq(c) for n := range out { fmt.Println(n) } }
In this pipeline:
gen
generates numbers and sends them to a channel.sq
reads numbers from the channel, squares them, and sends the results to another channel.
Best Practices for Concurrency in Go
-
Avoid Race Conditions: Use channels or synchronization primitives like
sync.Mutex
to prevent concurrent access to shared variables. -
Limit Goroutines: Uncontrolled spawning of goroutines can lead to resource exhaustion. Use worker pools or semaphores to limit concurrency.
-
Graceful Shutdown: Use context cancellation or closing channels to signal goroutines to stop.
-
Error Handling: Design your goroutines to handle errors gracefully and communicate them back to the main function or error-handling routines.
Conclusion
Go's concurrency model, centered around goroutines and channels, provides a robust framework for building concurrent applications. By leveraging these constructs and following established concurrency patterns, developers can write efficient, scalable, and maintainable code that fully utilizes modern multi-core processors.
For further reading and practical examples, consider exploring the following resources:
- Go Concurrency Patterns: Pipelines and cancellation
- Goroutines in Go: A Practical Guide to Concurrency
- Go Concurrency Patterns: A Deep Dive
FAQs
Unbuffered channels block until received, while buffered channels allow limited asynchronous sends.
Goroutines are managed by the Go runtime and are more lightweight, allowing thousands to run concurrently.
Use context cancellation or close channels to signal goroutines for graceful shutdown.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ