How to Mock Async Functions in Python
James Reed
Infrastructure Engineer · Leapcell
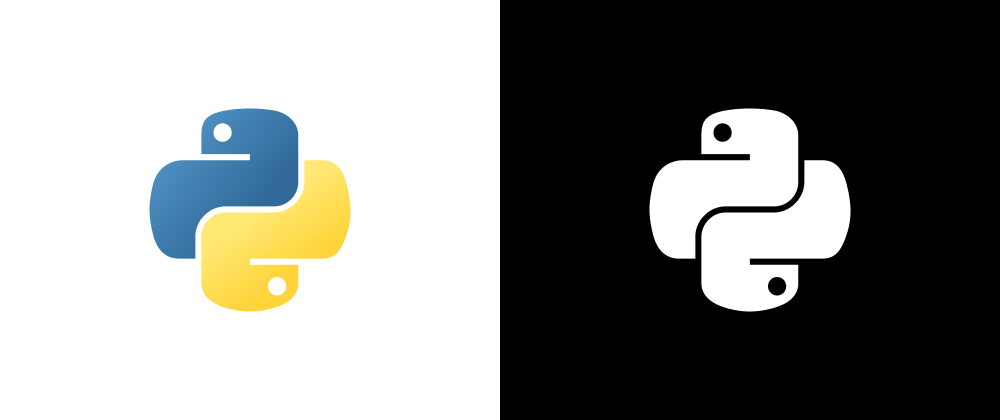
Mocking async
functions in Python is a common requirement when writing unit tests for codebases leveraging asynchronous programming. This practice ensures you can test functions that depend on async
operations without making actual network calls, database queries, or other side-effect-laden processes.
Why Mock Async Functions?
- Isolation: Mocking helps isolate the unit under test from external systems, ensuring the test focuses on the specific logic.
- Performance: Tests run faster because mocks avoid slow I/O operations.
- Determinism: Mocking avoids potential flakiness caused by real-world dependencies.
Tools to Mock Async Functions
Python's unittest.mock
module provides utilities for mocking, including support for asynchronous functions via AsyncMock
. If you're using Python 3.8 or later, AsyncMock
is the recommended approach. For earlier versions, MagicMock
can be adapted to mock async
functions.
Key Takeaways
- Mocking
async
functions isolates tests and improves performance by avoiding real dependencies. AsyncMock
is the recommended tool for mocking in Python 3.8 and later.- Avoid excessive mocking to maintain test reliability and clarity.
Mocking Async Functions with AsyncMock
Here’s a step-by-step guide to mocking async
functions using AsyncMock
:
import unittest from unittest.mock import AsyncMock, patch # Function to test def process_data(data_fetcher): async def wrapper(): data = await data_fetcher() return f"Processed: {data}" return wrapper class TestProcessData(unittest.TestCase): @patch("path.to.data_fetcher", new_callable=AsyncMock) def test_process_data(self, mock_fetcher): # Configure the mock to return a specific value mock_fetcher.return_value = "Mocked Data" # Test the function async def test_logic(): result = await process_data(mock_fetcher)() self.assertEqual(result, "Processed: Mocked Data") # Run the async test import asyncio asyncio.run(test_logic()) if __name__ == "__main__": unittest.main()
Key Points:
- Use
new_callable=AsyncMock
when patching anasync
function. - Configure the mock return value using
.return_value
. - For testing async logic, ensure the test function runs within an event loop.
Using MagicMock
for Older Python Versions
If you’re working with Python versions prior to 3.8, you can use MagicMock
to simulate async
behavior:
from unittest.mock import MagicMock # Mocking async function with MagicMock mock_fetcher = MagicMock() mock_fetcher.return_value = "Mocked Data" mock_fetcher.__aenter__.return_value = "Mocked Data" async def test(): result = await mock_fetcher() print(result) # Output: Mocked Data import asyncio asyncio.run(test())
Although MagicMock
can be used, it is less intuitive and less robust compared to AsyncMock
for mocking async
functions.
Discussion
- When to Mock: Mocking should be used sparingly and only for components external to the unit under test. Overusing mocks can lead to brittle tests.
- Alternatives to Mocking: Instead of mocking, consider using in-memory replacements or lightweight fakes for dependencies.
- Test Readability: Ensure the mocks and test setup are clear and expressive. Complicated mocks can obscure the purpose of the test.
FAQs
AsyncMock
is designed for mocking async
functions, while MagicMock
requires extra configuration for async behavior.
No, AsyncMock
is only available in Python 3.8 and later; use MagicMock
instead.
Use asyncio.run()
to execute the async
test logic within an event loop.
Conclusion
Mocking async
functions in Python enables effective testing of asynchronous code. While AsyncMock
simplifies this process in Python 3.8+, earlier versions can still achieve similar results using MagicMock
. By following best practices and avoiding excessive mocking, you can create maintainable and reliable test suites for your asynchronous Python code.
We are Leapcell, your top choice for deploying Python projects to the cloud.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ