Efficient File Reading in Go: Techniques and Best Practices
Daniel Hayes
Full-Stack Engineer · Leapcell
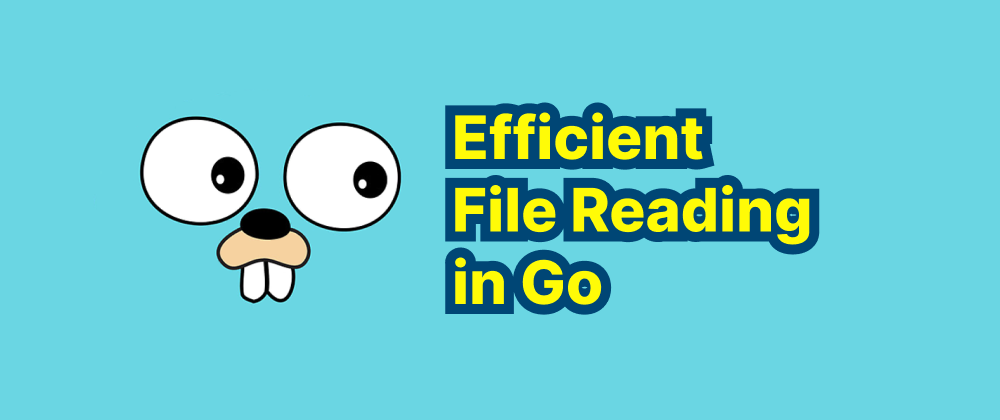
Key Takeaways
- Use
os.Open
andRead
for basic file reading, ensuring proper error handling and resource management. - Buffered reading with
bufio
improves efficiency, especially for large files. ioutil.ReadFile
provides a simple way to read entire files but may not be memory-efficient for large files.
In Go, handling file operations is a fundamental task, especially when dealing with data storage, configuration management, or logging functionalities. The os
package in Go's standard library provides robust support for file reading and writing operations.
Opening and Reading Files
To read a file in Go, you can utilize the os.Open
function, which opens the file in read-only mode and returns an *os.File
pointer. It's crucial to handle any potential errors during this operation and ensure that the file is properly closed after its usage to prevent resource leaks.
Here's an example demonstrating how to open and read a file:
package main import ( "fmt" "io" "os" ) func main() { // Attempt to open the file file, err := os.Open("example.txt") if err != nil { // Handle the error appropriately fmt.Println("Error opening file:", err) return } // Ensure the file is closed after reading defer file.Close() // Create a buffer to hold the file's content buffer := make([]byte, 1024) // Adjust buffer size as needed for { // Read from the file into the buffer bytesRead, err := file.Read(buffer) if err != nil { if err == io.EOF { // End of file reached break } // Handle other potential errors fmt.Println("Error reading file:", err) return } // Process the read bytes fmt.Print(string(buffer[:bytesRead])) } }
In this example, os.Open
is used to open "example.txt". The file.Read
method reads the file's content into a byte slice buffer. Reading continues in a loop until the end of the file is reached, indicated by the io.EOF
error. The defer
statement ensures that the file is closed once all operations are complete.
Using bufio
for Buffered Reading
For more efficient reading, especially with larger files, the bufio
package offers buffered reading capabilities. Buffered readers minimize the number of read system calls by reading larger chunks of data at once.
Here's how you can implement buffered reading:
package main import ( "bufio" "fmt" "os" ) func main() { // Attempt to open the file file, err := os.Open("example.txt") if err != nil { // Handle the error appropriately fmt.Println("Error opening file:", err) return } // Ensure the file is closed after reading defer file.Close() // Create a new buffered reader reader := bufio.NewReader(file) for { // Read the file line by line line, err := reader.ReadString('\n') if err != nil { if err.Error() == "EOF" { // End of file reached break } // Handle other potential errors fmt.Println("Error reading file:", err) return } // Process the line fmt.Print(line) } }
In this scenario, bufio.NewReader
wraps the *os.File
pointer, providing the ReadString
method to read the file line by line until a newline character is encountered. This approach is particularly useful for processing files where data is organized in lines, such as configuration files or logs.
Reading Files Using ioutil
For scenarios where you need to read the entire content of a file at once, the ioutil
package offers a straightforward method: ioutil.ReadFile
. This function reads the file's content into a byte slice, which can then be processed as needed.
Here's an example:
package main import ( "fmt" "io/ioutil" "os" ) func main() { // Read the entire file content content, err := ioutil.ReadFile("example.txt") if err != nil { // Handle the error appropriately fmt.Println("Error reading file:", err) return } // Process the content fmt.Print(string(content)) }
ioutil.ReadFile
simplifies the process by handling the opening and closing of the file internally. However, it's essential to use this method judiciously, especially with large files, to avoid excessive memory consumption.
Best Practices
-
Error Handling: Always check for and handle errors when performing file operations to ensure your program can gracefully handle unexpected situations.
-
Resource Management: Utilize
defer
to close files after operations are complete, ensuring that resources are properly released. -
Performance Considerations: Choose the appropriate reading method based on your use case. For large files or performance-critical applications, buffered reading with
bufio
is often more efficient.
By adhering to these practices and understanding the available tools in Go's standard library, you can effectively manage file reading operations in your applications.
FAQs
Use bufio.NewReader
and ReadString('\n')
to efficiently read files line by line.
Buffered reading with bufio
is the most efficient method for handling large files.
Use defer file.Close()
immediately after opening the file to ensure it’s closed automatically.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ