How to Host Playwright in the Cloud for Free
Min-jun Kim
Dev Intern · Leapcell
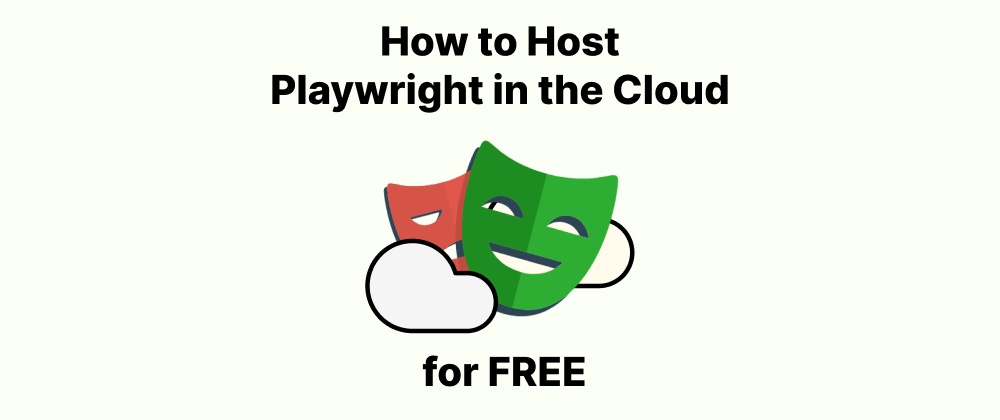
Playwright is a powerful tool designed to automate browser tasks, offering capabilities like screenshot capture, PDF generation, automated testing, web scraping, and more. It enables simulating human interactions with web pages, making it ideal for tasks such as content tracking, uptime monitoring, and web scraping.
Many scenarios benefit from deploying Playwright in the cloud, including:
- Triggering automated tests through APIs in CI/CD pipelines.
- Running cron jobs to check website availability.
- Managing large-scale distributed web scraping tasks.
With the scalable and pay-as-you-go model of serverless computing, cloud deployment for browser automation tasks makes a lot of sense. However, platforms like DigitalOcean often charge for idle time, making it inefficient for use cases requiring periodic browser interactions. Fortunately, a few platforms support serverless execution of Playwright: Leapcell and AWS Lambda.
This article explores how to use these platforms to deploy Playwright tasks, along with their benefits and challenges.
The Task
We’ll focus on a typical Playwright task: capturing a screenshot of a webpage.
The steps include:
- Visiting a given URL.
- Taking a screenshot.
- Returning the image.
Leapcell
Leapcell provides a flexible platform to deploy any application serverlessly, including Playwright automation tasks. Although it's not specifically designed for HTTP request handling, you can easily create an HTTP handler to run your Playwright tasks.
Code Example:
const { chromium } = require('playwright'); const { Hono } = require('hono'); const { serve } = require('@hono/node-server'); const screenshot = async (url) => { const browser = await chromium.launch({ args: ['--single-process'] }); const page = await browser.newPage(); await page.goto(url); const img = await page.screenshot(); await browser.close(); return img; }; const app = new Hono(); app.get('/', async (c) => { const url = c.req.query('url'); if (url) { const img = await screenshot(url); return c.body(img, { headers: { 'Content-Type': 'image/png' } }); } else { return c.text('Please provide a ?url=https://example.com/ parameter'); } }); const port = 8080; serve({ fetch: app.fetch, port }).on('listening', () => { console.log(`Server is running on port ${port}`); });
Leapcell allows you to deploy this Playwright script in a serverless environment, offering great scalability for tasks like web scraping or automated testing. Plus, Leapcell has a simple deployment process that ensures your Playwright automation is hosted without the burden of managing infrastructure.
Local Development
Debugging is easy: run the application like any other Node.js app with node index.js
.
Deployment
Deploy your app on Leapcell by configuring the build command, runtime, and service port. You’ll find it simple to get up and running.
Once the deployment is finished, your Playwright automation is live and accessible.
Summary
✅ Pros:
- Consistent local and cloud environments for easier debugging.
- Direct support for Playwright.
❌ Cons:
- Requires manual setup for HTTP request handling.
AWS Lambda
To run Playwright on AWS Lambda, you can leverage the playwright-core
package with a third-party Chromium library like alixaxel/chrome-aws-lambda
.
Third-party Chromium is necessary, because AWS imposes a 250MB limit on the size of a Lambda function. The default Chromium bundled with Puppeteer easily exceeds this limit (~170MB on macOS, ~282MB on Linux, ~280MB on Windows), making the use of a slimmed-down Chromium necessary.
Code Example:
const chromium = require('chrome-aws-lambda'); const playwright = require('playwright-core'); exports.handler = async (event) => { let browser = null; try { browser = await playwright.chromium.launch({ args: chromium.args, executablePath: await chromium.executablePath, headless: true, }); const page = await browser.newPage(); await page.goto(event.url); const screenshot = await page.screenshot(); return { statusCode: 200, headers: { 'Content-Type': 'image/jpeg' }, body: screenshot.toString('base64'), isBase64Encoded: true, }; } catch (error) { return { statusCode: 500, body: 'Failed to capture screenshot.', }; } finally { if (browser !== null) { await browser.close(); } } };
Local Development
Local debugging can be tricky with AWS Lambda, due to differences in runtime environments. As you can see in alixaxel/chrome-aws-lambda
's guide.
Deployment
Once the code is ready, you can deploy it via ZIP uploads or using Lambda Layers for dependencies. The setup can be tedious, especially when dealing with large files like Playwright's browser binaries.
Summary
✅ Pros:
- Streamlined code structure for Playwright tasks.
- Built-in support for modern browser automation.
❌ Cons:
- Potential risks from third-party dependencies (e.g., Chromium).
- Complex local debugging and deployment.
For projects requiring efficient cloud-hosted Playwright automation, Leapcell stands out as an excellent solution, providing easy deployment and a scalable, cost-effective environment.
For more details, check out the Leapcell Playwright documentation.