How to Pretty Print Structs in Go
Ethan Miller
Product Engineer · Leapcell
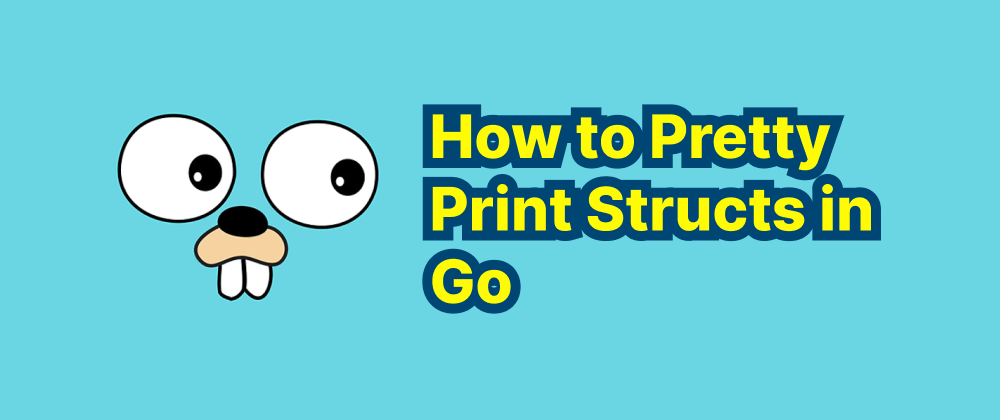
Key Takeaways
- Use
fmt.Printf("%+v", value)
to quickly print struct field names and values. json.MarshalIndent
provides clean, readable JSON output for structs.- The
spew
package helps inspect deeply nested or complex data structures.
When debugging or logging in Go, you often need to inspect the contents of a struct. However, the default output format might not be very readable. This is where pretty printing comes in handy. In this article, we’ll walk through different ways to pretty print structs in Go.
Using the fmt
Package
The standard fmt
package provides basic formatting options for printing structs.
%+v
Verb
To print struct field names along with their values, use the %+v
verb:
package main import ( "fmt" ) type User struct { Name string Email string Age int } func main() { user := User{"Alice", "alice@example.com", 30} fmt.Printf("%+v\n", user) }
Output:
{Name:Alice Email:alice@example.com Age:30}
This is more informative than %v
, which would omit the field names.
Using the encoding/json
Package
For a more readable and formatted output, you can serialize the struct to indented JSON.
package main import ( "encoding/json" "fmt" ) type User struct { Name string `json:"name"` Email string `json:"email"` Age int `json:"age"` } func main() { user := User{"Alice", "alice@example.com", 30} data, err := json.MarshalIndent(user, "", " ") if err != nil { fmt.Println("error:", err) return } fmt.Println(string(data)) }
Output:
{ "name": "Alice", "email": "alice@example.com", "age": 30 }
This approach is especially useful when printing nested structs.
Using the spew
Package
Another handy tool is github.com/davecgh/go-spew/spew
, which recursively prints Go data structures in a readable format.
First, install it:
go get -u github.com/davecgh/go-spew/spew
Then use it like this:
package main import ( "github.com/davecgh/go-spew/spew" ) type User struct { Name string Email string Age int } func main() { user := User{"Alice", "alice@example.com", 30} spew.Dump(user) }
Output:
(main.User) {
Name: (string) (len=5) "Alice",
Email: (string) (len=17) "alice@example.com",
Age: (int) 30
}
This is extremely helpful for inspecting complex or deeply nested structs.
Conclusion
Pretty printing structs in Go can be easily achieved using the standard fmt
package, JSON marshalling, or external packages like spew
. Each approach has its own use case:
- Use
fmt.Printf("%+v", value)
for quick inline debugging. - Use
json.MarshalIndent
for clean, human-readable logs. - Use
spew.Dump
for deep inspection during development.
Choose the method that best fits your needs, and make your debugging process more efficient!
FAQs
fmt.Println
omits field names, making output less informative for debugging.
Use json.MarshalIndent
when you need multi-line, human-friendly output or are logging structured data.
No, but spew
is useful for complex or deeply nested data that standard packages handle less clearly.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ