How to Convert Milliseconds to time.Time in Golang
Daniel Hayes
Full-Stack Engineer · Leapcell
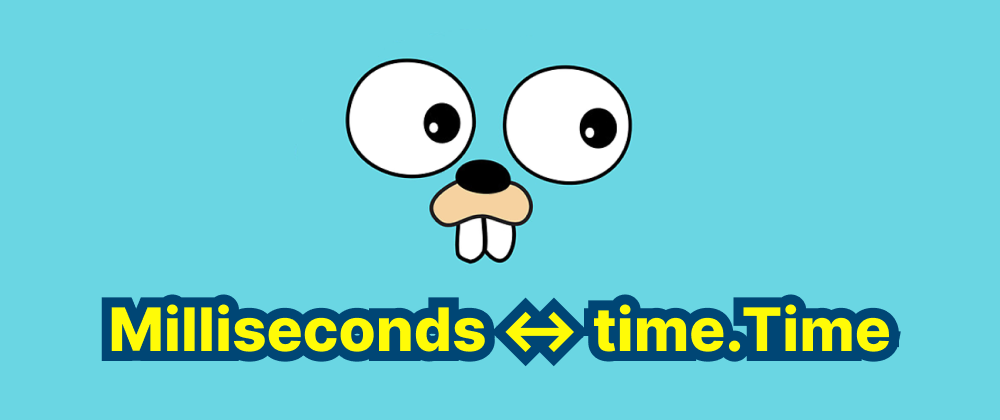
In Go (Golang), handling time in milliseconds is a common requirement, especially when interacting with systems or data that represent time as milliseconds since the Unix epoch (January 1, 1970). This article provides a comprehensive guide on converting milliseconds to Go's time.Time type and vice versa, ensuring precision and ease in your time manipulations.
Key Takeaways
- Convert Milliseconds to
time.Time
– Usetime.Unix(0, millis * int64(time.Millisecond))
ortime.UnixMilli(millis)
. - Convert
time.Time
to Milliseconds – Uset.UnixMilli()
for direct conversion. - Handle JSON Millisecond Timestamps – Store timestamps as
int64
and convert them usingtime.UnixMilli()
.
Converting Milliseconds to time.Time
To convert milliseconds since the Unix epoch to a time.Time
object in Go, you can use the time.Unix
function. This function accepts two parameters: seconds and nanoseconds. Since 1 millisecond equals 1,000,000 nanoseconds, you can calculate the seconds and remaining nanoseconds from the milliseconds value.
Here's how you can perform the conversion:
package main import ( "fmt" "time" ) func main() { // Milliseconds since Unix epoch millis := int64(1610000000000) // Convert milliseconds to time.Time t := time.Unix(0, millis*int64(time.Millisecond)) fmt.Println("Converted time:", t) }
Explanation
millis*int64(time.Millisecond)
converts milliseconds to nanoseconds.time.Unix(0, nanoseconds)
creates atime.Time
object corresponding to the given nanoseconds since the Unix epoch. Starting from Go version 1.17, the standard library introduced thetime.UnixMilli
function, which simplifies this conversion:
t := time.UnixMilli(millis)
This function directly returns a time.Time
object corresponding to the provided milliseconds since the Unix epoch.
Converting time.Time
to Milliseconds
If you have a time.Time
object and need to obtain the milliseconds since the Unix epoch, you can use the UnixMilli
method:
package main import ( "fmt" "time" ) func main() { // Current time t := time.Now() // Convert time.Time to milliseconds since Unix epoch millis := t.UnixMilli() fmt.Println("Milliseconds since epoch:", millis) }
Handling JSON with Milliseconds
Then working with JSON data that includes timestamps in milliseconds, you can define your struct to unmarshal the milliseconds into an int64
field and then convert it to time.Time
.
package main import ( "encoding/json" "fmt" "time" ) type Event struct { Name string `json:"name"` Timestamp int64 `json:"timestamp"` } func (e *Event) Time() time.Time { return time.UnixMilli(e.Timestamp) } func main() { data := []byte(`{"name": "Sample Event", "timestamp": 1610000000000}`) var event Event if err := json.Unmarshal(data, &event); err != nil { fmt.Println("Error:", err) return } fmt.Println("Event Name:", event.Name) fmt.Println("Event Time:", event.Time()) }
FAQs
Use time.UnixMilli(millis)
in Go 1.17+ or time.Unix(0, millis * int64(time.Millisecond))
for older versions.
Call t.UnixMilli()
on a time.Time
object to get the milliseconds since Unix epoch.
Store timestamps as int64
and convert them using time.UnixMilli()
when reading JSON data.
Conclusion
Converting between milliseconds and time.Time
in Go is straightforward, especially with the enhancements introduced in Go 1.17. By utilizing functions like time.UnixMilli
and methods like UnixMilli
, you can handle time conversions efficiently and accurately.
For more detailed information, you can refer to the Go documentation on the time
package.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ