How to Connect to MySQL Database Using DSN in Golang
Ethan Miller
Product Engineer · Leapcell
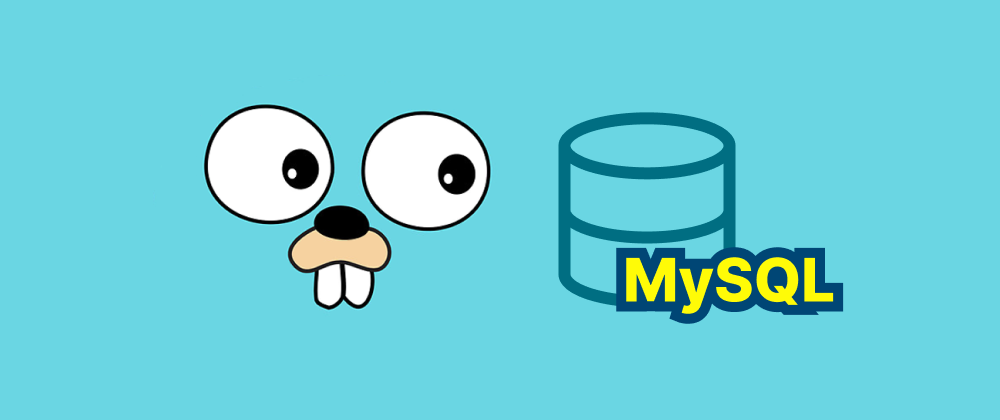
Connecting to a MySQL database in Go involves using the database/sql
package along with a MySQL driver, such as github.com/go-sql-driver/mysql
. A crucial aspect of establishing this connection is correctly formatting the Data Source Name (DSN), which contains the necessary connection parameters. This article provides a comprehensive guide on how to format the DSN in Go for MySQL connections.
Key Takeaways
- The MySQL DSN format in Go follows a structured syntax with essential connection parameters.
- Using
mysql.Config
andFormatDSN
enhances readability and reduces errors in DSN construction. - Additional DSN parameters can customize connection behavior, including timeouts and character sets.
Understanding the DSN Format
The DSN is a string that specifies the connection parameters for the database. The general format for a MySQL DSN in Go is:
[username[:password]@][protocol[(address)]][/dbname][?param1=value1&...¶mN=valueN]
Where:
username
: The MySQL username.password
: The password for the MySQL user.protocol
: The network protocol to use (e.g.,tcp
orunix
).address
: The network address, which can be in the form ofhost[:port]
for TCP connections or the path to the Unix domain socket.dbname
: The name of the database to connect to.param
: Additional connection parameters in the form of key-value pairs.
Example DSN
A typical DSN might look like this:
user:password@tcp(127.0.0.1:3306)/dbname?charset=utf8mb4&parseTime=True&loc=Local
In this example:
user
: The MySQL username.password
: The password for the MySQL user.tcp
: Specifies that the connection should use the TCP protocol.127.0.0.1:3306
: The MySQL server is hosted onlocalhost
at port3306
.dbname
: The name of the database to connect to.charset=utf8mb4
: Sets the character set toutf8mb4
.parseTime=True
: Enables parsing oftime.Time
values.loc=Local
: Sets the time zone to the local time zone.
Constructing the DSN in Go
Instead of manually constructing the DSN string, you can use the Config
struct provided by the mysql
driver to build the DSN programmatically. This approach enhances code readability and reduces the risk of errors.
Here's how you can do it:
package main import ( "database/sql" "log" "time" "github.com/go-sql-driver/mysql" ) func main() { // Capture connection properties. cfg := mysql.Config{ User: "user", Passwd: "password", Net: "tcp", Addr: "127.0.0.1:3306", DBName: "dbname", AllowNativePasswords: true, ParseTime: true, Loc: time.Local, } // Get a database handle. db, err := sql.Open("mysql", cfg.FormatDSN()) if err != nil { log.Fatal(err) } defer db.Close() // Ping the database to verify the connection. if err := db.Ping(); err != nil { log.Fatal(err) } log.Println("Connected to the database successfully!") }
In this code:
We define the connection properties using the mysql.Config
struct.
The FormatDSN
method constructs the DSN string based on the provided configuration.
We use sql.Open
to create a database handle and db.Ping()
to verify the connection.
Additional Connection Parameters
The DSN can include various parameters to customize the connection behavior. Some commonly used parameters include:
charset
: Specifies the character set for the connection.collation
: Sets the collation for the connection.timeout
: Defines the maximum wait time for a new connection.readTimeout
: Sets the I/O read timeout.writeTimeout
: Sets the I/O write timeout.
For a comprehensive list of supported parameters, refer to the Go-MySQL-Driver documentation.
FAQs
The format is [username[:password]@][protocol[(address)]][/dbname][?param1=value1&...]
.
Use mysql.Config
and call cfg.FormatDSN()
to construct a DSN programmatically.
Common parameters include charset
, parseTime
, timeout
, readTimeout
, and writeTimeout
.
Conclusion
Properly formatting the DSN is essential for establishing a successful connection between your Go application and a MySQL database. By leveraging the mysql.Config
struct and its FormatDSN
method, you can construct the DSN in a clear and error-free manner, ensuring a robust and maintainable database connection setup.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ