How to Convert a String to JSON in JavaScript, Python, and Java
James Reed
Infrastructure Engineer · Leapcell
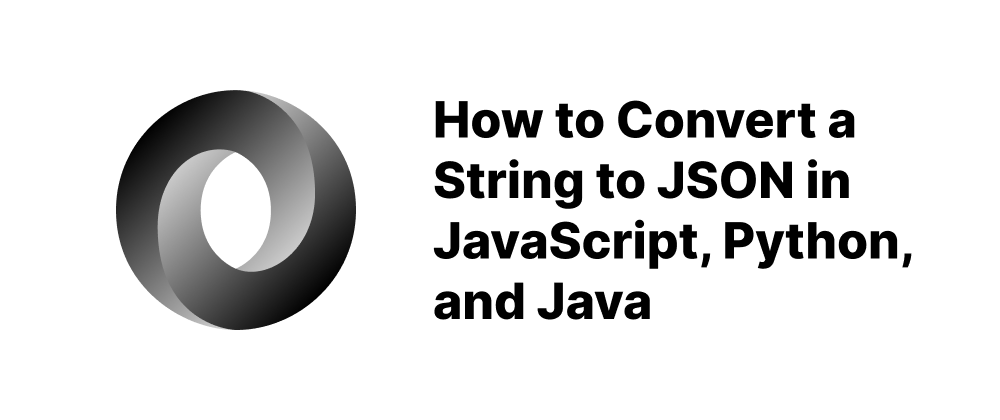
Key Takeaways
- Use built-in methods like
JSON.parse()
in JavaScript andjson.loads()
in Python for safe conversion. - Always validate the string format before converting to avoid runtime errors.
- Java requires external libraries like Gson or
org.json
for JSON parsing.
Converting a string to a JSON object is a common task in software development, especially when dealing with data interchange between systems. This article provides a comprehensive guide on how to perform this conversion in JavaScript, Python, and Java.
JavaScript
In JavaScript, the JSON.parse()
method is the standard way to convert a JSON-formatted string into a JavaScript object.
Example:
const jsonString = '{"name":"John", "age":30, "city":"New York"}'; const obj = JSON.parse(jsonString); console.log(obj.name); // Output: John
Note: Ensure that the string is in valid JSON format; otherwise, JSON.parse()
will throw a SyntaxError
.
Using a Reviver Function
The JSON.parse()
method can accept a reviver function to transform the resulting object before it's returned.
const jsonString = '{"name":"John", "birth":"1986-12-14", "city":"New York"}'; const obj = JSON.parse(jsonString, function (key, value) { if (key === "birth") { return new Date(value); } return value; }); console.log(obj.birth); // Output: Date object
Caution: Avoid using eval()
or the Function
constructor to parse JSON strings, as they can execute arbitrary code and pose security risks.
Python
Python provides several methods to convert a JSON-formatted string into a Python dictionary.
Using json.loads()
The json
module's loads()
function is the most straightforward and recommended method.
import json json_string = '{"name": "John", "age": 30, "city": "New York"}' data = json.loads(json_string) print(data["name"]) # Output: John
Note: If the string is not in valid JSON format, json.loads()
will raise a json.JSONDecodeError
.
Using ast.literal_eval()
The ast
module's literal_eval()
function can safely evaluate strings containing Python literals.
import ast json_string = '{"name": "John", "age": 30, "city": "New York"}' data = ast.literal_eval(json_string) print(data["name"]) # Output: John
Caution: While ast.literal_eval()
is safer than eval()
, it should still be used cautiously and only with trusted input.
Java
In Java, converting a JSON-formatted string to a JSON object can be achieved using libraries like org.json
, Gson, or Jackson.
Using org.json
import org.json.JSONObject; public class Main { public static void main(String[] args) { String jsonString = "{\"name\":\"John\", \"age\":30, \"city\":\"New York\"}"; JSONObject obj = new JSONObject(jsonString); System.out.println(obj.getString("name")); // Output: John } }
Using Gson
import com.google.gson.Gson; import com.google.gson.JsonObject; public class Main { public static void main(String[] args) { String jsonString = "{\"name\":\"John\", \"age\":30, \"city\":\"New York\"}"; Gson gson = new Gson(); JsonObject obj = gson.fromJson(jsonString, JsonObject.class); System.out.println(obj.get("name").getAsString()); // Output: John } }
Note: Gson is a popular library developed by Google for converting Java objects to JSON and vice versa.
Conclusion
Converting strings to JSON objects is essential for data processing and communication in modern applications. Each programming language offers its own set of tools and libraries to facilitate this conversion. Always ensure that the input string is in valid JSON format and handle exceptions appropriately to maintain robust and secure code.
FAQs
Parsing will fail and throw an error like SyntaxError
(JavaScript) or JSONDecodeError
(Python).
No, eval()
poses security risks and should be avoided.
Gson is widely used and offers a simple syntax for converting strings to JSON.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ