How to Convert JSON to YAML: A Practical Guide
Grace Collins
Solutions Engineer · Leapcell
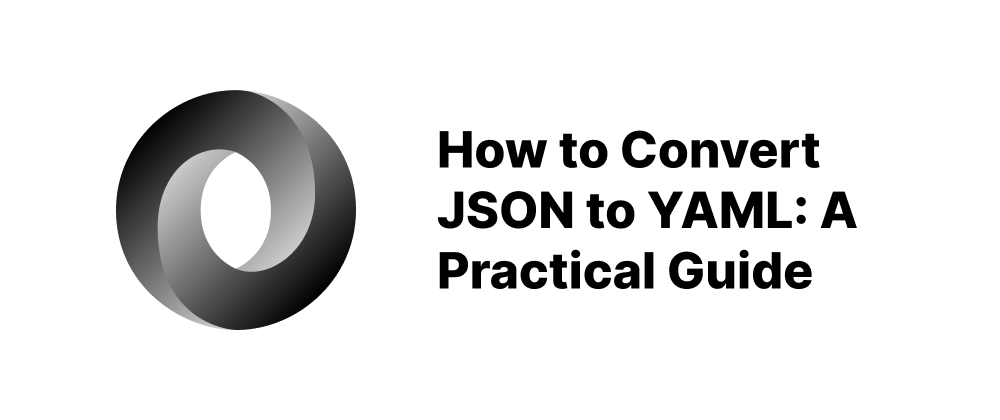
Key Takeaways
- YAML is more readable and concise than JSON, making it ideal for configuration files.
- Conversion can be done via command-line tools like
yq
or online converters. - Programming libraries like Python's PyYAML offer custom automation for conversion.
JSON (JavaScript Object Notation) and YAML (YAML Ain’t Markup Language) are widely used data serialization formats. While JSON is prevalent in web APIs and data interchange, YAML is often favored for configuration files due to its readability. This guide explores various methods to convert JSON to YAML, including command-line tools, online converters, and programming libraries.
Why Convert JSON to YAML?
- Enhanced Readability: YAML's indentation-based structure makes complex data more human-readable.
- Conciseness: YAML often requires fewer characters than JSON, leading to cleaner configuration files.
- Support for Comments: Unlike JSON, YAML allows comments, aiding in documentation.
- Flexibility: YAML supports additional data types like dates and complex structures, offering more versatility.
Method 1: Using Command-Line Tools
1. yq
(Go-based)
The Go-based yq
is a powerful command-line tool for YAML processing. To convert JSON to YAML:
yq -p=json -o=yaml file.json > file.yaml
This command reads file.json
and outputs the equivalent YAML to file.yaml
. Ensure you have the correct version of yq
installed, as there are multiple tools with the same name.
2. jq
and yq
(Python-based)
Alternatively, you can use the Python-based yq
, which wraps around jq
:
jq . file.json | yq -y > file.yaml
This pipeline formats the JSON using jq
and then converts it to YAML using yq
.
Method 2: Online Converters
For quick conversions without installing tools, several online converters are available:
- Online YAML Tools: A free, ad-free tool that converts JSON to YAML directly in your browser.
- JSON Formatter: Offers JSON to YAML conversion with options to download or share the output.
- Site24x7 Tools: Provides a user-friendly interface for JSON to YAML conversion.
These tools are especially useful for small tasks or when working on devices without development environments.
Method 3: Using Programming Languages
Python
Python's PyYAML
library can convert JSON to YAML:
import json import yaml with open('file.json') as json_file: data = json.load(json_file) with open('file.yaml', 'w') as yaml_file: yaml.dump(data, yaml_file, default_flow_style=False)
This script reads file.json
and writes the equivalent YAML to file.yaml
.
Go
In Go, you can use the yaml.v2
package:
package main import ( "encoding/json" "fmt" "log" "gopkg.in/yaml.v2" ) func main() { jsonData := []byte(`{"name": "John", "age": 30}`) var data map[string]interface{} if err := json.Unmarshal(jsonData, &data); err != nil { log.Fatal(err) } yamlData, err := yaml.Marshal(&data) if err != nil { log.Fatal(err) } fmt.Println(string(yamlData)) }
This program converts a JSON object to YAML and prints the result.
Conclusion
Converting JSON to YAML can be achieved through various methods, each suited to different needs:
- Command-Line Tools: Ideal for quick conversions and scripting.
- Online Converters: Convenient for occasional use without installations.
- Programming Libraries: Best for integrating into applications or handling complex data transformations.
Choose the method that aligns with your workflow and project requirements.
FAQs
YAML is more human-readable and supports comments, making it better for configurations.
Online tools or yq
are the easiest and fastest for most users.
Yes, using libraries like PyYAML in Python or yaml.v2
in Go.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ