How to Convert JSON to XML: A Comprehensive Guide
Daniel Hayes
Full-Stack Engineer · Leapcell
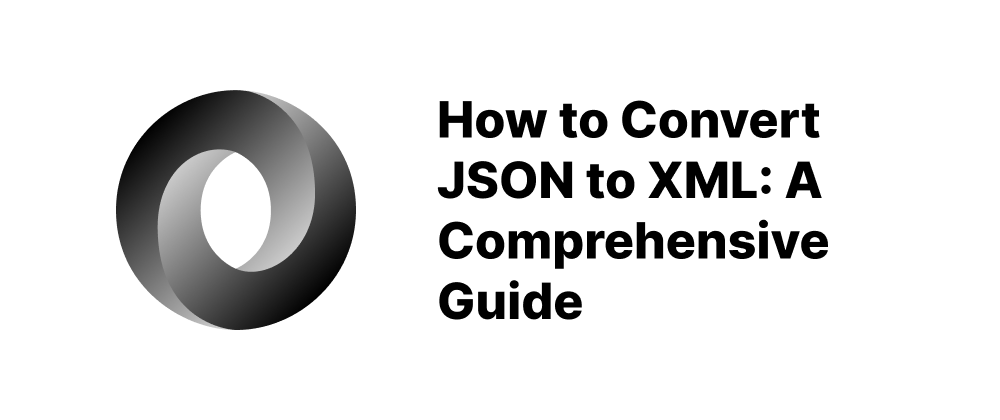
Key Takeaways
- JSON can be converted to XML using online tools or programming libraries.
- Choosing the right method depends on your environment and data complexity.
- Handling root elements and arrays correctly is essential for accurate conversion.
Converting JSON (JavaScript Object Notation) to XML (eXtensible Markup Language) is a common requirement when integrating systems that use different data formats. This guide explores various methods to perform this conversion, catering to different programming environments and user preferences.
1. Online Tools for Quick Conversion
For users seeking a quick and code-free solution, several online tools facilitate JSON to XML conversion:
-
Site24x7 JSON to XML Converter: This tool allows you to convert JSON data to XML format. It supports attributes in XML elements by using specific JSON key conventions.
-
JSON Formatter's JSON to XML Converter: A user-friendly interface that converts JSON to XML, with options to download, save, share, and print the converted data.
-
Oxygen XML Editor: A comprehensive XML editor that includes tools for converting JSON to XML, suitable for more complex or large-scale transformations.
2. Programmatic Conversion Methods
a. Java Using Jackson Library
The Jackson library in Java provides robust support for converting JSON to XML:
import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.dataformat.xml.XmlMapper; public class JsonToXmlExample { public static void main(String[] args) throws Exception { String json = "{\"name\":\"John\", \"age\":30}"; ObjectMapper jsonMapper = new ObjectMapper(); Object obj = jsonMapper.readValue(json, Object.class); XmlMapper xmlMapper = new XmlMapper(); String xml = xmlMapper.writeValueAsString(obj); System.out.println(xml); } }
This code parses a JSON string into a Java object and then writes it as an XML string.
b. JavaScript Using xml-js Library
In JavaScript, the xml-js
library simplifies the conversion process:
const { json2xml } = require('xml-js'); const json = { name: 'Garage', cars: [ { color: 'red', maxSpeed: 120, age: 2 }, { color: 'blue', maxSpeed: 100, age: 3 }, { color: 'green', maxSpeed: 130, age: 2 }, ], }; const options = { compact: true, spaces: 4 }; const xml = json2xml(json, options); console.log(xml);
This script converts a JSON object to an XML string with formatted indentation.
c. .NET Using Newtonsoft.Json (Json.NET)
In .NET environments, the Newtonsoft.Json library (also known as Json.NET) offers methods for converting JSON to XML:
using Newtonsoft.Json; using System.Xml; string json = @"{ 'name': 'John', 'age': 30 }"; XmlDocument doc = JsonConvert.DeserializeXmlNode(json, "Root"); Console.WriteLine(doc.OuterXml);
This code deserializes a JSON string into an XmlDocument
, specifying a root element name.
3. Considerations During Conversion
-
Root Elements: XML requires a single root element. When converting JSON objects with multiple top-level properties, ensure to wrap them within a root element.
-
Attribute Representation: Some tools and libraries allow representing JSON properties as XML attributes. For instance, in Json.NET, prefixing property names with "@" denotes attributes.
-
Arrays Handling: JSON arrays are typically converted to repeated XML elements. Ensure that the conversion tool or library handles arrays according to your requirements.
-
Special Characters: JSON property names with characters not allowed in XML element names (e.g., spaces, symbols) may need to be sanitized or encoded during conversion.
4. Conclusion
Converting JSON to XML can be achieved through various methods, each suitable for different scenarios:
-
Online Tools: Ideal for quick, manual conversions without coding.
-
Programming Libraries: Suitable for automated, repeatable conversions within applications.
Choose the method that best fits your specific needs, considering factors like data complexity, integration requirements, and development environment.
FAQs
Yes, online tools like Site24x7 or JSON Formatter allow code-free conversion.
Java, JavaScript, and .NET all support conversion using libraries like Jackson, xml-js, and Json.NET.
XML requires a single root element to structure the document properly.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ